Enter two strings and Concatenate It
Write an assembly language assignment program with a subroutine STRCAT which will ask the user for two different strings and will display the concatenated string back to the user. A sample run of the program is:
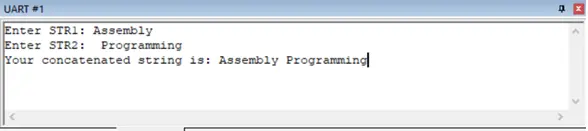
Solution
EXPORT main ; this line is needed to interface with init.s
IMPORT GetCh ; Input one ASCII character from the UART #1 window (from keyboard)
IMPORT PutCh ; Output one ASCII character to the UART #1 window
IMPORT PutCRLF ; Output CR and LF to the UART #1 window
IMPORT UDivMod ; Perform unsigned division to obtain quotient and remainder
IMPORT GetDec ; Input a signed number from the UART #1 window
IMPORT PutDec ; Output a signed number to the UART #1 window
IMPORT GetStr ; Input a CR-terminated ASCII string from the UART #1 window
IMPORT PutStr ; Output null-terminated ASCII string to the UART #1 window
AREA MyCode, CODE, READONLY
ALIGN
main
PUSH {LR} ; save return address of caller in init.s
; Prompt user to enter first string
LDR R0, =Prompt1
BL PutStr
; Read string from keyboard, save in STR1
LDR R0, =STR1
MOV R1,#MaxSTR1
BL GetStr
; Prompt user to enter second string
LDR R0, =Prompt2
BL PutStr
; Read string from keyboard, save in STR2
LDR R0, =STR2
MOV R1,#MaxSTR2
BL GetStr
LDR R0,=STR1 ; point to start of first string
LDR R1,=STR2 ; point to start of second string
BL STRCAT ; concatenate strings using subroutine
; Print result message
LDR R0, =Result
BL PutStr
; Print concatenated string
LDR R0, =STR1
BL PutStr
POP {PC} ; return from main (our last executable instruction)
; Subroutine to concatenate the two strings with addresses R0 and R1
; by adding second string at the end of the first one
STRCAT
PUSH {LR} ; save return address of caller
LOOP1
LDRB R2, [R0] ; load character from first string
CMP R2, #0 ; check if it's end of string
BEQ LOOP2 ; if so, go to next loop
ADD R0, R0, #1 ; else, increment position in string
B LOOP1 ; repeat loop
LOOP2
LDRB R2, [R1], #1 ; load character from second string and increment
STRB R2, [R0], #1 ; save character in first string and increment
CMP R2, #0 ; check if it's end of string
BNE LOOP2 ; if not zero, repeat loop
POP {PC} ; return from subroutine
MaxSTR1 EQU 200 ; maximum string length for first string
MaxSTR2 EQU 100 ; maximum string length for second string
Prompt1 DCB "Enter STR1: ",0
Prompt2 DCB "Enter STR2: ",0
Result DCB "Your concatenated string: ",0
ALIGN
AREA MyData, DATA, READWRITE
STR1 SPACE MaxSTR1+1
STR2 SPACE MaxSTR2+1
ALIGN
;-------------------- END OF MODIFIABLE CODE ----------------------
ALIGN
END ; end of source program in this file
Similar Samples
Explore our comprehensive collection of programming homework samples at ProgrammingHomeworkHelp.com. Our diverse examples cover various programming languages and topics, showcasing our proficiency in delivering clear, well-commented solutions. Whether you're struggling with algorithms, data structures, or software development projects, our samples provide valuable insights and guidance to excel in your programming assignments.
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language