Instructions
Requirements and Specifications
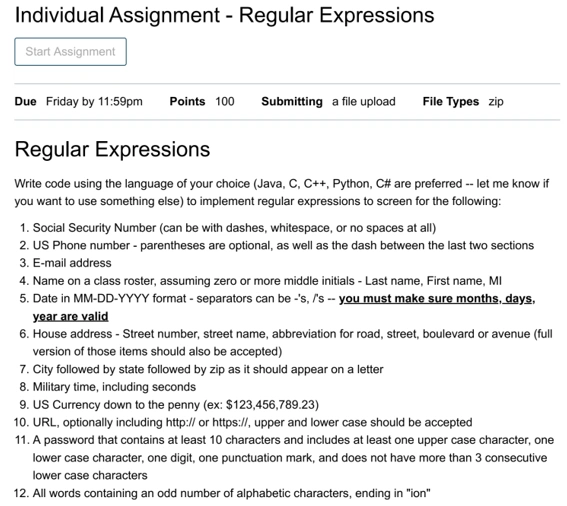
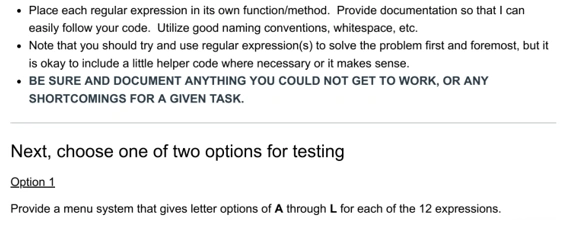
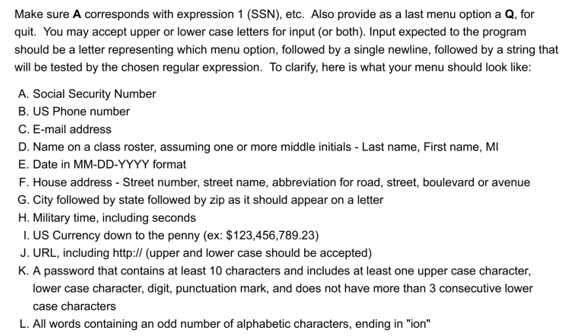
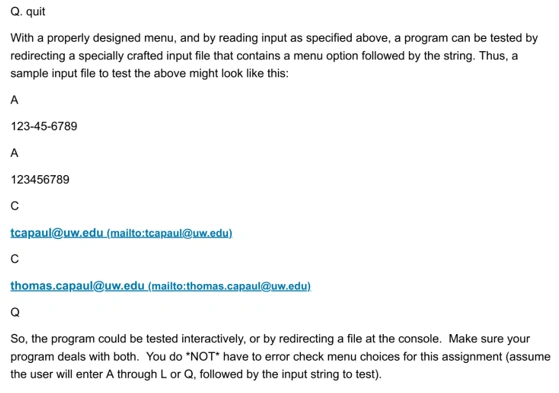
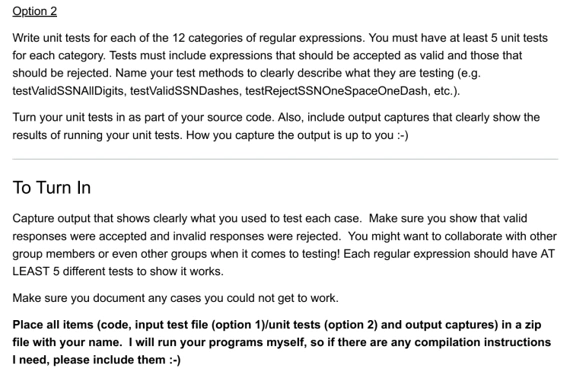
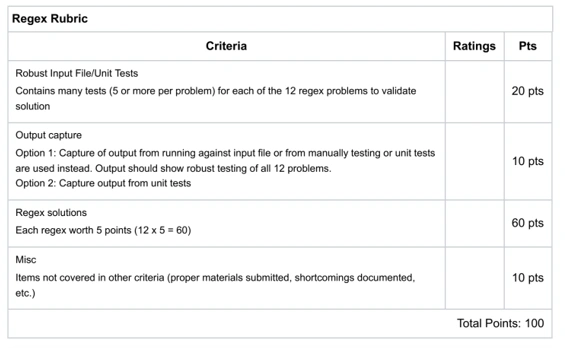
Source Code
import org.junit.Assert;
import org.junit.Test;
public class RegexTest {
@Test
public void aValidAllDigits() {
Assert.assertTrue(RegexUtils.tryParseA("1234567890"));
Assert.assertTrue(RegexUtils.tryParseA("7777777777"));
Assert.assertTrue(RegexUtils.tryParseA("0987654321"));
}
@Test
public void aValidDashes() {
Assert.assertTrue(RegexUtils.tryParseA("123-456-7890"));
Assert.assertTrue(RegexUtils.tryParseA("777-777-7777"));
Assert.assertTrue(RegexUtils.tryParseA("098-765-4321"));
}
@Test
public void aValidWhitespaces() {
Assert.assertTrue(RegexUtils.tryParseA("123 456 7890"));
Assert.assertTrue(RegexUtils.tryParseA("777 777 7777"));
Assert.assertTrue(RegexUtils.tryParseA("098 765 4321"));
}
@Test
public void aRejectOneSpaceOneDash() {
Assert.assertFalse(RegexUtils.tryParseA("1293-456 7890"));
Assert.assertFalse(RegexUtils.tryParseA("777 77777-77"));
Assert.assertFalse(RegexUtils.tryParseA("098 76-54321"));
}
@Test
public void aRejectMisc() {
Assert.assertFalse(RegexUtils.tryParseA("sdfsfsdfsdfs"));
Assert.assertFalse(RegexUtils.tryParseA("12313443"));
Assert.assertFalse(RegexUtils.tryParseA(""));
}
@Test
public void bValidWithParenthesis() {
Assert.assertTrue(RegexUtils.tryParseB("(123)456-7890"));
Assert.assertTrue(RegexUtils.tryParseB("(777)777-7777"));
Assert.assertTrue(RegexUtils.tryParseB("(098)765-4321"));
}
@Test
public void bValidWithoutParenthesis() {
Assert.assertTrue(RegexUtils.tryParseB("123-456-7890"));
Assert.assertTrue(RegexUtils.tryParseB("777-777-7777"));
Assert.assertTrue(RegexUtils.tryParseB("098-765-4321"));
}
@Test
public void bRegectWithParenthesis() {
Assert.assertFalse(RegexUtils.tryParseB("(1236)56-7890"));
Assert.assertFalse(RegexUtils.tryParseB("(777)7677-777"));
Assert.assertFalse(RegexUtils.tryParseB("(098)76-46321"));
}
@Test
public void bRegectWithoutParenthesis() {
Assert.assertFalse(RegexUtils.tryParseB("1236-56-7890"));
Assert.assertFalse(RegexUtils.tryParseB("777-7677-777"));
Assert.assertFalse(RegexUtils.tryParseB("098-76-46321"));
}
@Test
public void bRejectMisc() {
Assert.assertFalse(RegexUtils.tryParseB("sdfsfsdfsdfs"));
Assert.assertFalse(RegexUtils.tryParseB("12313443"));
Assert.assertFalse(RegexUtils.tryParseB(""));
}
@Test
public void cValid() {
Assert.assertTrue(RegexUtils.tryParseC("jsmith@gmail.com"));
Assert.assertTrue(RegexUtils.tryParseC("janedoe@yahoo.com"));
Assert.assertTrue(RegexUtils.tryParseC("123123@mail.com"));
}
@Test
public void cRejectNoDomain() {
Assert.assertFalse(RegexUtils.tryParseC("jsmith@gmail."));
Assert.assertFalse(RegexUtils.tryParseC("janedoe@yahoo."));
Assert.assertFalse(RegexUtils.tryParseC("123123@mail."));
}
@Test
public void cRejectNoAt() {
Assert.assertFalse(RegexUtils.tryParseC("jsmithgmail.com"));
Assert.assertFalse(RegexUtils.tryParseC("janedoeyahoo.com"));
Assert.assertFalse(RegexUtils.tryParseC("123123mail.com"));
}
@Test
public void cRejectNoDot() {
Assert.assertFalse(RegexUtils.tryParseC("jsmith@gmailcom"));
Assert.assertFalse(RegexUtils.tryParseC("janedoe@yahoocom"));
Assert.assertFalse(RegexUtils.tryParseC("123123@mailcom"));
}
@Test
public void cRejectNoUsername() {
Assert.assertFalse(RegexUtils.tryParseC("@gmail.com"));
Assert.assertFalse(RegexUtils.tryParseC("@yahoo.com"));
Assert.assertFalse(RegexUtils.tryParseC("@mail.com"));
}
@Test
public void dValid0MiddleInitials() {
Assert.assertTrue(RegexUtils.tryParseD("Smith, John"));
Assert.assertTrue(RegexUtils.tryParseD("Doe, Jane"));
Assert.assertTrue(RegexUtils.tryParseD("Brown, Sam"));
}
@Test
public void dValid1MiddleInitials() {
Assert.assertTrue(RegexUtils.tryParseD("Smith, John, A"));
Assert.assertTrue(RegexUtils.tryParseD("Doe, Jane, B"));
Assert.assertTrue(RegexUtils.tryParseD("Brown, Sam, C"));
}
@Test
public void dValidSeveralMiddleInitials() {
Assert.assertTrue(RegexUtils.tryParseD("Smith, John, AB"));
Assert.assertTrue(RegexUtils.tryParseD("Doe, Jane, ABCD"));
Assert.assertTrue(RegexUtils.tryParseD("Brown, Sam, AHKJSH"));
}
@Test
public void dRejectWrongMiddleInitials() {
Assert.assertFalse(RegexUtils.tryParseD("Smith, John, A B"));
Assert.assertFalse(RegexUtils.tryParseD("Doe, Jane, ABrD"));
Assert.assertFalse(RegexUtils.tryParseD("Brown, Sam, AH43JSH"));
}
@Test
public void dRejectMisc() {
Assert.assertFalse(RegexUtils.tryParseD("Smith"));
Assert.assertFalse(RegexUtils.tryParseD(""));
Assert.assertFalse(RegexUtils.tryParseD("Brown, S4m, AHJSH"));
}
@Test
public void eValidDash() {
Assert.assertTrue(RegexUtils.tryParseE("01-01-1992"));
Assert.assertTrue(RegexUtils.tryParseE("28-02-2000"));
Assert.assertTrue(RegexUtils.tryParseE("31-12-2012"));
}
@Test
public void eValidSlash() {
Assert.assertTrue(RegexUtils.tryParseE("01/01/1992"));
Assert.assertTrue(RegexUtils.tryParseE("28/02/2000"));
Assert.assertTrue(RegexUtils.tryParseE("31/12/2012"));
}
@Test
public void eInvalidMonth() {
Assert.assertFalse(RegexUtils.tryParseE("01-21-1992"));
Assert.assertFalse(RegexUtils.tryParseE("28-32-2000"));
Assert.assertFalse(RegexUtils.tryParseE("31-42-2012"));
}
@Test
public void eInvalidDay() {
Assert.assertFalse(RegexUtils.tryParseE("32-21-1992"));
Assert.assertFalse(RegexUtils.tryParseE("48-32-2000"));
Assert.assertFalse(RegexUtils.tryParseE("91-42-2012"));
}
@Test
public void eInvalidDate() {
Assert.assertFalse(RegexUtils.tryParseE("30-02-1992"));
Assert.assertFalse(RegexUtils.tryParseE("31-04-2000"));
Assert.assertFalse(RegexUtils.tryParseE("31-11-2012"));
}
@Test
public void fValidFullAbbrevation() {
Assert.assertTrue(RegexUtils.tryParseF("4, Baker, street"));
Assert.assertTrue(RegexUtils.tryParseF("33, Hollywood, avenue"));
Assert.assertTrue(RegexUtils.tryParseF("234, Jameson, boulevard"));
}
@Test
public void fValidShortAbbrevation() {
Assert.assertTrue(RegexUtils.tryParseF("4, Baker, st"));
Assert.assertTrue(RegexUtils.tryParseF("33, Hollywood, ave"));
Assert.assertTrue(RegexUtils.tryParseF("234, Jameson, blvd"));
}
@Test
public void fRejectWrongAbbrevation() {
Assert.assertFalse(RegexUtils.tryParseF("4, Baker, ssdft"));
Assert.assertFalse(RegexUtils.tryParseF("33, Hollywood, sdf"));
Assert.assertFalse(RegexUtils.tryParseF("234, Jameson, bl43vd"));
}
@Test
public void fRejectWrongNumber() {
Assert.assertFalse(RegexUtils.tryParseF("4sdf, Baker, st"));
Assert.assertFalse(RegexUtils.tryParseF("tt, Hollywood, ave"));
Assert.assertFalse(RegexUtils.tryParseF("twentytwo, Jameson, blvd"));
}
@Test
public void fRejectWrongMisc() {
Assert.assertFalse(RegexUtils.tryParseF(""));
Assert.assertFalse(RegexUtils.tryParseF("3232342"));
Assert.assertFalse(RegexUtils.tryParseF("sdfsdfsdf"));
}
@Test
public void gValid() {
Assert.assertTrue(RegexUtils.tryParseG("Atlanta GA 12345"));
Assert.assertTrue(RegexUtils.tryParseG("Juneau AL 77777"));
Assert.assertTrue(RegexUtils.tryParseG("St.Paul MN 98765"));
}
@Test
public void gWrongCity() {
Assert.assertFalse(RegexUtils.tryParseG("Atl anta GA 12345"));
Assert.assertFalse(RegexUtils.tryParseG("Jun??eau AL 77777"));
Assert.assertFalse(RegexUtils.tryParseG("St4Paul MN 98765"));
}
@Test
public void gWrongState() {
Assert.assertFalse(RegexUtils.tryParseG("Atlanta GDA 12345"));
Assert.assertFalse(RegexUtils.tryParseG("Juneau A4 77777"));
Assert.assertFalse(RegexUtils.tryParseG("St.Paul N 98765"));
}
@Test
public void gWrongZip() {
Assert.assertFalse(RegexUtils.tryParseG("Atlanta GA 123495"));
Assert.assertFalse(RegexUtils.tryParseG("Juneau AL 77F77"));
Assert.assertFalse(RegexUtils.tryParseG("St.Paul MN 9875"));
}
@Test
public void gWrongMisc() {
Assert.assertFalse(RegexUtils.tryParseG(""));
Assert.assertFalse(RegexUtils.tryParseG("dfsdfsdfsdf"));
Assert.assertFalse(RegexUtils.tryParseG("2342323"));
}
@Test
public void hValid() {
Assert.assertTrue(RegexUtils.tryParseH("00:00"));
Assert.assertTrue(RegexUtils.tryParseH("11:11"));
Assert.assertTrue(RegexUtils.tryParseH("23:59"));
}
@Test
public void hRejectNoColon() {
Assert.assertFalse(RegexUtils.tryParseH("0000"));
Assert.assertFalse(RegexUtils.tryParseH("1111"));
Assert.assertFalse(RegexUtils.tryParseH("2359"));
}
@Test
public void hRejectWrongHours() {
Assert.assertFalse(RegexUtils.tryParseH("99:00"));
Assert.assertFalse(RegexUtils.tryParseH("24:11"));
Assert.assertFalse(RegexUtils.tryParseH("45:59"));
}
@Test
public void hRejectWrongMinutes() {
Assert.assertFalse(RegexUtils.tryParseH("00:99"));
Assert.assertFalse(RegexUtils.tryParseH("11:65"));
Assert.assertFalse(RegexUtils.tryParseH("23:60"));
}
@Test
public void hRejectMisc() {
Assert.assertFalse(RegexUtils.tryParseH(""));
Assert.assertFalse(RegexUtils.tryParseH("234234234"));
Assert.assertFalse(RegexUtils.tryParseH("adfsfsdfs"));
}
@Test
public void iValid() {
Assert.assertTrue(RegexUtils.tryParseI("$0.00"));
Assert.assertTrue(RegexUtils.tryParseI("$123,456,789.23"));
Assert.assertTrue(RegexUtils.tryParseI("$100.98"));
}
@Test
public void iRejectNoDollar() {
Assert.assertFalse(RegexUtils.tryParseI("0.00"));
Assert.assertFalse(RegexUtils.tryParseI("123,456,789.23"));
Assert.assertFalse(RegexUtils.tryParseI("100.98"));
}
@Test
public void iRejectNoPennies() {
Assert.assertFalse(RegexUtils.tryParseI("$0"));
Assert.assertFalse(RegexUtils.tryParseI("$123,456,789"));
Assert.assertFalse(RegexUtils.tryParseI("$100"));
}
@Test
public void iRejectNoSeparators() {
Assert.assertFalse(RegexUtils.tryParseI("$1000000"));
Assert.assertFalse(RegexUtils.tryParseI("$123456789"));
Assert.assertFalse(RegexUtils.tryParseI("$1000"));
}
@Test
public void iRejectNotDigit() {
Assert.assertFalse(RegexUtils.tryParseI("$0.Y0"));
Assert.assertFalse(RegexUtils.tryParseI("$1 3,456,789.23"));
Assert.assertFalse(RegexUtils.tryParseI("$10p.98"));
}
@Test
public void jValidHttpLower() {
Assert.assertTrue(RegexUtils.tryParseJ("http://yahoo.com"));
Assert.assertTrue(RegexUtils.tryParseJ("http://google.com/search"));
Assert.assertTrue(RegexUtils.tryParseJ("http://www.mail.com/authentication"));
}
@Test
public void jValidHttpsLower() {
Assert.assertTrue(RegexUtils.tryParseJ("https://yahoo.com"));
Assert.assertTrue(RegexUtils.tryParseJ("https://google.com/search"));
Assert.assertTrue(RegexUtils.tryParseJ("https://www.mail.com/authentication"));
}
@Test
public void jValidHttpUpper() {
Assert.assertTrue(RegexUtils.tryParseJ("HTTP://YAHOO.COM"));
Assert.assertTrue(RegexUtils.tryParseJ("HTTP://GOOGLE.COM/SEARCH"));
Assert.assertTrue(RegexUtils.tryParseJ("HTTP://WWW.MAIL.COM/AUTHENTICATION"));
}
@Test
public void jValidHttpsUpper() {
Assert.assertTrue(RegexUtils.tryParseJ("HTTPS://YAHOO.COM"));
Assert.assertTrue(RegexUtils.tryParseJ("HTTPS://GOOGLE.COM/SEARCH"));
Assert.assertTrue(RegexUtils.tryParseJ("HTTPS://WWW.MAIL.COM/AUTHENTICATION"));
}
@Test
public void jRejectMisc() {
Assert.assertFalse(RegexUtils.tryParseJ("HTTP://YAH OO.COM"));
Assert.assertFalse(RegexUtils.tryParseJ("ftp://GOOGLE.COM/SEARCH"));
Assert.assertFalse(RegexUtils.tryParseJ("://WWW.MAIL.COM/AUTHENTICATION"));
}
@Test
public void kValid() {
Assert.assertTrue(RegexUtils.tryParseK("Ab.0AAAAAA"));
Assert.assertTrue(RegexUtils.tryParseK("1?45HjGHoi434io"));
Assert.assertTrue(RegexUtils.tryParseK(",.,GHfk54ooT"));
}
@Test
public void kRejectLength() {
Assert.assertFalse(RegexUtils.tryParseK("Ab.0AAAA"));
Assert.assertFalse(RegexUtils.tryParseK("1?45Hj4io"));
Assert.assertFalse(RegexUtils.tryParseK(",4ooT"));
}
@Test
public void kRejectNoDigit() {
Assert.assertFalse(RegexUtils.tryParseK("Ab.OAAAAAA"));
Assert.assertFalse(RegexUtils.tryParseK("l?RTHjGHoiYTYio"));
Assert.assertFalse(RegexUtils.tryParseK(",.,GHfkGHooT"));
}
@Test
public void lRejectNoPunct() {
Assert.assertFalse(RegexUtils.tryParseK("Abp0AAAAAA"));
Assert.assertFalse(RegexUtils.tryParseK("14RTHjGHoiYTYio"));
Assert.assertFalse(RegexUtils.tryParseK("090GHfkGHooT"));
}
@Test
public void kReject3Lowers() {
Assert.assertFalse(RegexUtils.tryParseK("Ab.OAAaaaa"));
Assert.assertFalse(RegexUtils.tryParseK("1?RTHjGHoiYTyio"));
Assert.assertFalse(RegexUtils.tryParseK(",.,GHfkGhooT"));
}
@Test
public void lValid() {
Assert.assertTrue(RegexUtils.tryParseL("ion"));
Assert.assertTrue(RegexUtils.tryParseL("liion"));
Assert.assertTrue(RegexUtils.tryParseL("abcdefghijklmnoprsion"));
}
@Test
public void lRejectEven() {
Assert.assertFalse(RegexUtils.tryParseL("lion"));
Assert.assertFalse(RegexUtils.tryParseL("lioion"));
Assert.assertFalse(RegexUtils.tryParseL("xabcdefghijklmnoprsion"));
}
@Test
public void lRejectIncorrectSuffix() {
Assert.assertFalse(RegexUtils.tryParseL("abc"));
Assert.assertFalse(RegexUtils.tryParseL("abcef"));
Assert.assertFalse(RegexUtils.tryParseL("xabcdefghijklmnoprs"));
}
@Test
public void lRejectNotLetters() {
Assert.assertFalse(RegexUtils.tryParseL("li!on"));
Assert.assertFalse(RegexUtils.tryParseL("44liion"));
Assert.assertFalse(RegexUtils.tryParseL("abcdefghi lmn p sion"));
}
@Test
public void lRejectShort() {
Assert.assertFalse(RegexUtils.tryParseL(""));
Assert.assertFalse(RegexUtils.tryParseL("i"));
Assert.assertFalse(RegexUtils.tryParseL("io"));
}
}
public class RegexUtils {
public static boolean tryParseA(String s) {
return s.matches("\\d{3}[\\s\\-]?\\d{3}[\\s\\-]?\\d{4}");
}
public static boolean tryParseB(String s) {
return s.matches("(\\(\\d{3}\\)|\\d{3}-)\\d{3}[\\s\\-]?\\d{4}");
}
public static boolean tryParseC(String s) {
return s.matches("\\w+\\@\\w+\\.\\w+");
}
public static boolean tryParseD(String s) {
return s.matches("[a-zA-Z\\-]+,\\s[a-zA-Z\\-]+(,\\s[A-Z]+)?");
}
public static boolean tryParseE(String s) {
return s.matches("((((0\\d|1\\d|2\\d|(30))\\-((04)|(06)|(09)|(11)))|((0\\d|1\\d|2\\d|(3[0-1]))\\-((01)|(03)|(05)|(07)|(08)|(10)|(12)))|((0\\d|1\\d|2[0-8])\\-02))\\-\\d{4})|" +
"((((0\\d|1\\d|2\\d|(30))\\/((04)|(06)|(09)|(11)))|((0\\d|1\\d|2\\d|(3[0-1]))\\/((01)|(03)|(05)|(07)|(08)|(10)|(12)))|((0\\d|1\\d|2[0-8])\\/02))\\/\\d{4})");
}
public static boolean tryParseF(String s) {
return s.matches("\\d+,\\s[\\s\\w]+,\\s([sS][tT]|[aA][vV][eE]|[bB][lL][vV][dD]|" +
"[sS][tT][rR][eE][eE][tT]|[aA][vV][eE][nN][uU][eE]|[bB][oO][uU][lL][eE][vV][aA][rR][dD])");
}
public static boolean tryParseG(String s) {
return s.matches("[a-zA-Z\\.\\-]+\\s[A-Z][A-Z]\\s\\d{5}");
}
public static boolean tryParseH(String s) {
return s.matches("([01]\\d|2[0-3]):([0-5]\\d)");
}
public static boolean tryParseI(String s) {
return s.matches("\\$(\\d|\\d{2}|\\d{3})(,\\d{3})*\\.\\d{2}");
}
public static boolean tryParseJ(String s) {
return s.matches("(http:\\/\\/|HTTP:\\/\\/|https:\\/\\/|HTTPS:\\/\\/)[\\w\\.\\/]+");
}
public static boolean tryParseK(String s) {
return s.matches("(?=.*[a-z])(?=.*[A-Z])(?=.*[0-9])(?=.*[\\.,\\?\\!])(?=.{10,})(?!.*[a-z]{3}).*");
}
public static boolean tryParseL(String s) {
return s.matches("([a-zA-Z][a-zA-Z])*ion");
}
}
Related Samples
Discover our Java Assignment Samples for expert solutions to programming challenges. Covering topics from object-oriented programming to data structures and algorithms, these examples provide clear explanations and step-by-step implementations. Ideal for students looking to enhance their Java skills and excel academically with practical, educational resources.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java