Instructions
Objective
Write a java assignment program to work with arrays and characters.
Requirements and Specifications
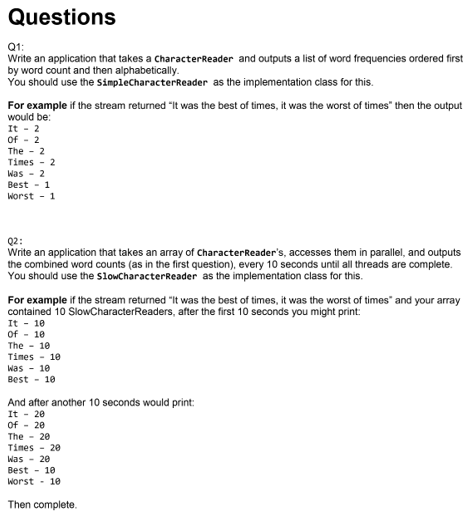
Screenshot
Source Code
QUESTION 1
package javatest;
import javatest.exception.EndOfStreamException;
import javatest.reader.CharacterReader;
import javatest.reader.SimpleCharacterReader;
import java.util.*;
public class Q1 {
public static void main(String[] args) {
Map map = new HashMap<>();
StringBuilder builder = new StringBuilder();
CharacterReader reader = new SimpleCharacterReader();
try {
while(true) {
char c = reader.getNextChar();
if (Character.isAlphabetic(c)) {
builder.append(builder.length() == 0 ? Character.toUpperCase(c) : Character.toLowerCase(c));
}
else {
if (builder.length() > 0) {
map.merge(builder.toString(), 1, Integer::sum);
builder = new StringBuilder();
}
}
}
}
catch (EndOfStreamException e) {
if (builder.length() > 0) {
map.merge(builder.toString(), 1, Integer::sum);
}
}
List> pairs = new ArrayList<>(map.entrySet());
pairs.sort((o1, o2) -> {
int diff = o2.getValue() - o1.getValue();
if (diff == 0) {
return o1.getKey().compareTo(o2.getKey());
}
return diff;
});
for (Map.Entry pair : pairs) {
System.out.println(pair.getKey() + " - " + pair.getValue());
}
}
}
QUESTION 2
package javatest;
import javatest.exception.EndOfStreamException;
import javatest.reader.CharacterReader;
import javatest.reader.SimpleCharacterReader;
import javatest.reader.SlowCharacterReader;
import java.util.*;
public class Q2 {
private static final Map map = new HashMap<>();
private static boolean[] completed;
private static void printState() {
List> pairs;
synchronized (map) {
pairs = new ArrayList<>(map.entrySet());
}
pairs.sort((o1, o2) -> {
int diff = o2.getValue() - o1.getValue();
if (diff == 0) {
return o1.getKey().compareTo(o2.getKey());
}
return diff;
});
for (Map.Entry pair : pairs) {
System.out.println(pair.getKey() + " - " + pair.getValue());
}
}
public static void main(String[] args) {
int threadNum = 10;
Thread[] threads = new Thread[threadNum];
completed = new boolean[threadNum];
for (int i = 0; i < threadNum; i++) {
final int index = i;
threads[i] = new Thread(() -> {
CharacterReader reader = new SlowCharacterReader();
StringBuilder builder = new StringBuilder();
try {
while (true) {
char c = reader.getNextChar();
if (Character.isAlphabetic(c)) {
builder.append(builder.length() == 0 ? Character.toUpperCase(c) : Character.toLowerCase(c));
} else {
if (builder.length() > 0) {
synchronized (map) {
map.merge(builder.toString(), 1, Integer::sum);
}
builder = new StringBuilder();
}
}
}
} catch (EndOfStreamException e) {
if (builder.length() > 0) {
synchronized (map) {
map.merge(builder.toString(), 1, Integer::sum);
}
}
completed[index] = true;
}
});
threads[i].start();
}
long startTime = System.currentTimeMillis();
long seconds = 0;
while(true) {
boolean stop = true;
for (boolean b : completed) {
if (!b) {
stop = false;
break;
}
}
if (stop) {
break;
}
if ((System.currentTimeMillis() - startTime) / 1000 >= (seconds + 10)) {
seconds += 10;
printState();
System.out.println();
}
}
for (int i = 0; i < threadNum; i++) {
try {
threads[i].join();
} catch (Exception ignored) {
}
}
System.out.println("Final:");
printState();
}
}
Similar Samples
Explore ProgrammingHomeworkHelp.com for a preview of our expertly crafted programming assignment samples. From Java and Python to C++ and beyond, these examples showcase our proficiency in delivering precise solutions. Whether you're a student seeking clarity or a professional refining skills, our samples demonstrate our commitment to excellence in tackling programming challenges effectively."
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java