Instructions
Objective
Write a program to work with queues stacks and ADTs in java language.
Requirements and Specifications
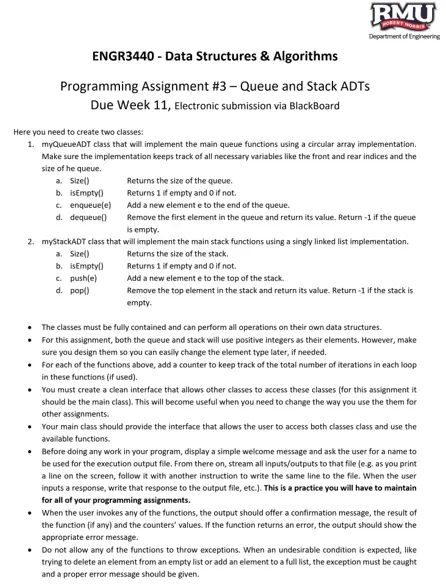
Source Code
QUEUE
//ENGR3440 - Data Structures and Algorithms for Engineers
//Spring 2022
//Assignment #3, Queue and Stack ADTs.
//Dominic Reagle
import java.util.NoSuchElementException;
public class myQueueADT {
private static class Node {
private E data;
private Node next;
public Node(E data, Node next) {
this.data = data;
this.next = next;
}
}
private Node front;
private Node rear;
private int size;
public myQueueADT() {
front = null;
rear = null;
size = 0;
}
public int Size() {
return size;
}
public boolean isEmpty() {
return size == 0;
}
public void enqueue(E e) {
Node newNode = new Node(e, null);
if (isEmpty()) {
front = newNode;
rear = newNode;
}
else {
rear.next = newNode;
rear = newNode;
}
size++;
}
public E dequeue() {
if (isEmpty()) {
throw new NoSuchElementException();
}
E result = front.data;
front = front.next;
if (front == null) {
rear = null;
}
size--;
return result;
}
}
STACK
//ENGR3440 - Data Structures and Algorithms for Engineers
//Spring 2022
//Assignment #3, Queue and Stack ADTs.
//Dominic Reagle
import java.util.NoSuchElementException;
public class myStackADT {
private static class Node {
private E data;
private Node next;
public Node(E data, Node next) {
this.data = data;
this.next = next;
}
}
private Node top;
private int size;
public myStackADT() {
top = null;
size = 0;
}
public int Size() {
return size;
}
public boolean isEmpty() {
return size == 0;
}
public void push(E e) {
Node newNode = new Node(e, top);
top = newNode;
size++;
}
public E pop() {
if (isEmpty()) {
throw new NoSuchElementException();
}
E result = top.data;
top = top.next;
size--;
return result;
}
}
Similar Samples
Explore our Samples section to see a range of completed programming assignments. These examples highlight our proficiency in various programming languages and showcase the quality of our solutions. Whether you need help with basic coding or complex projects, our samples demonstrate our ability to deliver top-notch assistance to meet your academic needs.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java