Instructions
Objective
Write a java assignment program to solve array problems.
Requirements and Specifications
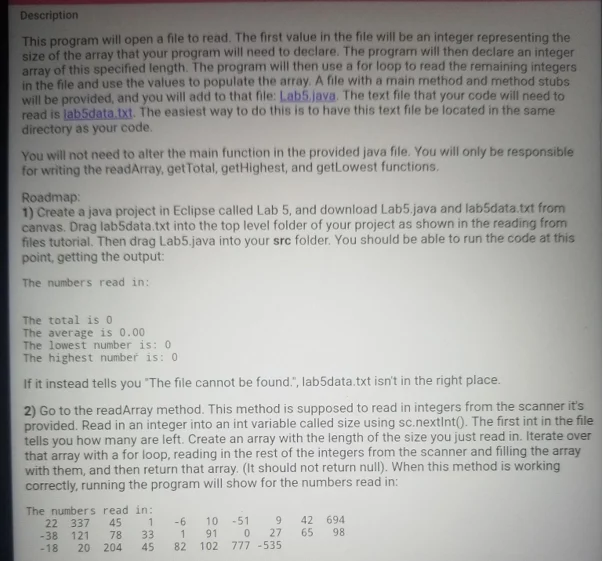
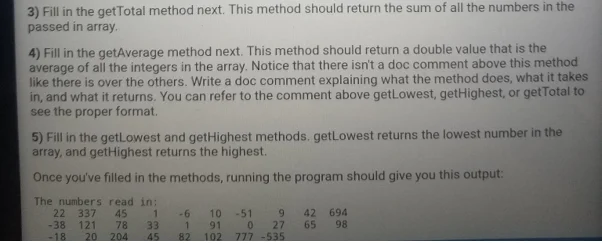
Source Code
import java.io.File;
import java.util.Scanner;
public class Lab5 {
public static int[] readArray(Scanner sc)
{
/*
* Given a file_name, this function will read the content
* from that file and generate an array of integers based on the values
* in the file
*/
// Read first line containing the size of the array
int size = sc.nextInt();
// Create Array
int array[] = new int[size];
// Now, read the next n lines
for(int i = 0; i < size; i++) {
array[i] = sc.nextInt();
}
// return array
return array;
}
static int getTotal(int[] array)
{
/*
* Given an array of integers, this function returns the sum of all the elements in the array
*/
// get size
int size = array.length;
int total = 0; // store total in this variable
// Loop through array
for(int i = 0; i < size; i++) {
total += array[i];
}
return total;
}
static double getAverage(int[] array)
{
/*
* Given an array of inegers, this function returns the average between all values in the array
*/
// get size
int size = array.length;
// get total sum of all elements in array
int total = getTotal(array);
// calculate average
double average = (double)total/size;
return average;
}
static int getLowest(int array[])
{
/*
* Given an array of integers, this function returns the smallest integer on it
*/
// get size
int size = array.length;
// assume that the lowest is the first element
int lowest = array[0];
// Loop through array starting at index 1
for(int i = 1; i < size; i++) {
if(array[i] < lowest) { // current element at index i is lower than 'lowest'
lowest = array[i];
}
}
return lowest;
}
static int getHighest(int array[])
{
/*
* Given an array of integers, this function returns the highest integer on it
*/
// get size
int size = array.length;
// assume that the highest is the first element
int highest = array[0];
// Loop through array starting at index 1
for(int i = 1; i < size; i++) {
if(array[i] > highest) { // current element at index i is jhigher than 'highest'
highest = array[i];
}
}
return highest;
}
public static void main(String[] args) throws Exception {
// read array
Scanner sc = new Scanner(new File("lab5data.txt"));
int array[] = readArray(sc);
System.out.println("The numbers read from file are: ");
for(int i = 0; i < array.length; i++) {
System.out.print(array[i] + " ");
}
System.out.println("");
System.out.println("Total: " + getTotal(array));
System.out.println("Average: " + getAverage(array));
System.out.println("Lowest: " + getLowest(array));
System.out.println("Highest: " + getHighest(array));
}
}
Similar Samples
Explore our sample section to see how ProgrammingHomeworkHelp.com can assist you with your coding assignments. Our expertly crafted examples showcase the quality and depth of our work, ensuring you receive the best support for your programming projects. Check out our samples today!
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java