Instructions
Objective
Write a program to solve algorithm problems in Java language. Java is a powerful and widely-used programming language known for its portability and robustness. The assignment might involve implementing sorting algorithms, data structures, or solving mathematical challenges. By understanding the principles of object-oriented programming and mastering Java syntax, you can develop efficient and elegant solutions. Completing a Java assignment will not only enhance your coding skills but also deepen your understanding of algorithms and their applications in real-world scenarios.
Requirements and Specifications
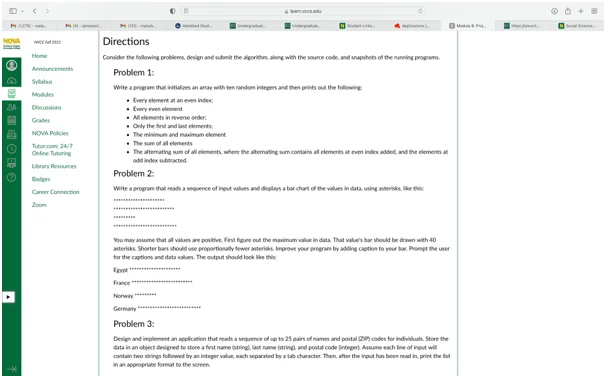
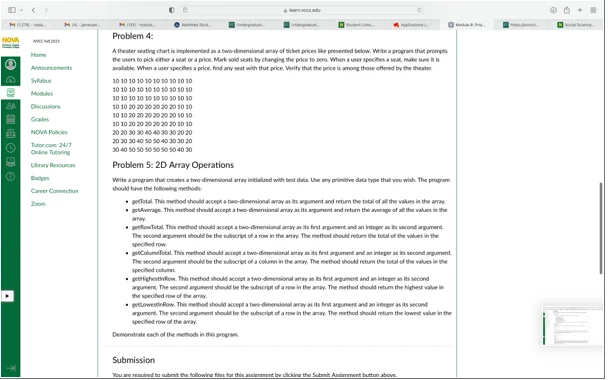
Source Code
PROBLEM 1
import java.util.Random;
public class Problem1
{
public static void main(String[] args) throws Exception {
// First, create an empty array of 10 elements
int array[] = new int[10];
// Now, create a random object
Random random = new Random();
// Now, fill with random integers between 0 and 1000
System.out.print("The array is: ");
for(int i = 0; i < 10; i++) {
array[i] = random.nextInt(1000);
System.out.print(array[i] + " ");
}
System.out.println("");
// Print element at an even index
System.out.print("Elements at even index: ");
for(int i = 0; i < 10; i++) {
if(i%2 == 0) // index is event
{
System.out.print(array[i] + " ");
}
}
System.out.println("");
// Even elements
System.out.print("Even elements: ");
for(int i = 0; i < 10; i++) {
if(array[i]%2 == 0) // element is even
{
System.out.print(array[i] + " ");
}
}
System.out.println("");
// Reverse order
System.out.print("Elements in reverse order: ");
for(int i = 0; i < 9; i++) {
System.out.print(array[10-i-1] + " ");
}
System.out.println("");
// First and last
System.out.println("The first element is: " + array[0]);
System.out.println("The last element is: " + array[9]);
// Get min and max
int min = array[0];
int max = array[0];
for(int i = 1; i < 10; i++) {
if(array[i] < min) // current element is lower than current min
{
min = array[i];
}
if(array[i] > max) // current element is bigger than current max
{
max = array[i];
}
}
System.out.println("The min element is: " + min);
System.out.println("The max element is: " + max);
// Create variable to store sum and alternating sum
int sum = 0, alternating_sum = 0;
for(int i = 0; i < 9; i++)
{
sum += array[i];
if(i%2 == 0) // if index is event, add element to alternating sum
{
alternating_sum += array[i];
}
else { // index is odd, subtract element to alternating sum
alternating_sum -= array[i];
}
}
System.out.println("The sum is: " + sum);
System.out.println("The alternating sum is: " + alternating_sum);
}
}
PROBLEM 2
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
// Create a private class to stoe the label and the value
class Value {
String label;
int value;
public Value(String label, int value) {
this.label = label;
this.value =value;
};
}
public class Problem2 {
static void printElement(Value value, int n_asterisks)
{
System.out.print(value.label + " ");
for(int i = 0; i < n_asterisks; i++)
{
System.out.print("*");
}
System.out.println("");
}
public static void main(String[] args) throws Exception {
// Create Scanner to read user's input
Scanner sc = new Scanner(System.in);
// Create a list of Values
List values = new ArrayList();
// Variable to store the max value
// Variables to receive label and value from user
String label;
int value;
String input;
// Ask user for values until s/he enters an empty line
while(true)
{
System.out.print("Enter label and value separated by space (e.g: Egypt 10): ");
input = sc.nextLine();
// if input is empty, exit loop
if(input.length() == 0) {
break;
}
// Split
String[] data = input.split(" ");
try
{
label = data[0];
value = Integer.valueOf(data[1]);
// Create value object
Value val = new Value(label, value);
// Add to list
values.add(val);
}
catch(Exception ex) // if we get an exception, it must be because user entered a non-integer for the value
{
System.out.println("Please enter a valid label and value.");
}
}
// Now, get max value
int max_value = values.get(0).value;
for(int i = 1; i < values.size(); i++) {
if(values.get(i).value > max_value) {
max_value = values.get(i).value;
}
}
// Now, print
for(int i = 0; i < values.size(); i++)
{
// Calculate number of asteriks for this value
int n_asterisks = values.get(i).value*40/max_value;
// Display
printElement(values.get(i), n_asterisks);
}
}
}
PROBLEM 3
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
// Create class to store the names and zip. This class is named Person
class Person
{
private String firstName;
private String lastName;
private int zip;
public Person(String firstName, String lastName, int zip) {
this.firstName = firstName;
this.lastName = lastName;
this.zip = zip;
}
// getters
public String getFirstName() {return firstName;}
public String getLastName() {return lastName;}
public int getZipCode() {return zip;}
}
public class Problem3 {
public static void main(String[] args) throws Exception {
// Create Scanner
Scanner sc = new Scanner(System.in);
// Number of input to be read
int N = 2;
// List to store inputs
List persons = new ArrayList();
// Read
String input, firstName, lastName;
int zip;
for(int i = 0; i < N; i++) {
input = sc.nextLine();
String[] data = input.split("\t");
firstName = data[0];
lastName = data[1];
zip = Integer.valueOf(data[2]);
// Create Person object
Person person = new Person(firstName, lastName, zip);
// Add to list
persons.add(person);
}
System.out.println("");
// Now, print
System.out.println(String.format("%-10s %25s %25s", "First Name", "Last Name", "ZIP Code"));
System.out.println(String.format("%-10s %25s %25s", "----------", "---------", "--------"));
for(Person p: persons)
{
System.out.println(String.format("%-10s %25s %25d", p.getFirstName(), p.getLastName(), p.getZipCode()));
}
}
}
PROBLEM 4
import java.util.Scanner;
public class Problem4 {
static void printSeats(int[][] seats, int N)
{
// This function prints seats
for(int i = 0; i < N; i++)
{
for(int j = 0; j < N; j++) {
System.out.print(String.format("%5d", seats[i][j]));
}
System.out.println("");
}
}
static void displayMenu()
{
/*
* This function prints the menu to the user
*/
System.out.println("1) Select seat by position");
System.out.println("2) Select seat by price");
System.out.println("3) Exit");
}
static int getInteger(String message, int lb, int ub, Scanner sc) {
/*
* This function will ask user for a positive integer
* If the user enters a valid input, the function will display
* an error message and reprompt
*/
int value;
while(true)
{
try{
System.out.print(message);
value = Integer.valueOf(sc.nextLine());
if(value >= lb && value <= ub) {
return value;
}
else {
System.out.println("Please enter a number between " + lb + " and " + ub + ".");
}
}
catch(Exception ex) {
System.out.println("Please enter a valid number.");
}
}
}
public static void main(String[] args) throws Exception {
// Create Scanner
scanner sc = new Scanner(System.in);
// Define size of theater (we assumed it squared, so same number of rows and columns)
int N = 10;
// Create 2-D array to store seats
int seats[][] = new int[N][N];
// Initialize seats
for(int i = 0; i < N; i++)
{
for(int j = 0; j < N; j++)
{
if(i < 3)
{
seats[i][j] = 10;
}
else if(i >= 3 && i < 6) {
seats[i][j] = 20;
}
else if(i >= 6 && i < 8) {
seats[i][j] = 30;
}
else if(i == 8) {
seats[i][j] =40;
}
else {
seats[i][j] = 50;
}
}
}
// Now, ask user for a seat or price
int option;
while(true)
{
printSeats(seats, N);
System.out.println("");
displayMenu();
// Ask for option
option = getInteger("Enter option: ", 1, 3, sc);
if(option == 1) // enter seat by row and column
{
int row = getInteger("Enter row (1-" + N + "): ", 1, 10, sc);
int column = getInteger("Enter column (1-" + N + "): ", 1, 10, sc);
// Check if seat is availab
if(seats[row-1][column-1] != 0) {
System.out.println("You purchased the seat located at (" + row + "," + column + ").");
seats[row-1][column-1] = 0;
}
else {
System.out.println("Sorry, this seat is already taken.");
}
}
else if(option == 2) {
int price = getInteger("Enter price: ", 10, 50, sc);
// Check if there is a seat with that price available
boolean found = false;
for(int i = 0; i < N; i++) {
for(int j = 0; j < N; j++) {
if(seats[i][j] == price) {
found = true;
seats[i][j] = 0;
System.out.println("You purchased the seat located at (" + (i+1) + "," + (j+1) + ").");
break;
}
}
if(found) {
break;
}
}
if(!found) // no seat found
{
System.out.println("Sorry, there is not seat available with that price.");
}
}
else if(option == 3) {
System.out.println("Good Bye!");
break;
}
}
}
}
PROBLEM 5
import java.util.Random;
import java.util.Scanner;
public class Problem5 {
static void printArray(int[][] array, int N)
{
// This function prints array
for(int i = 0; i < N; i++)
{
for(int j = 0; j < N; j++) {
System.out.print(String.format("%5d", array[i][j]));
}
System.out.println("");
}
}
static void displayMenu()
{
/*
* This function prints the menu to the user
*/
System.out.println("1) Calculate total of data");
System.out.println("2) Calculate average of data");
System.out.println("3) Row total");
System.out.println("4) Column total");
System.out.println("5) Highest in row");
System.out.println("6) Lowest in row");
System.out.println("7) Highest in column");
System.out.println("8) Lowest in column");
System.out.println("9) Exit");
}
static int getInteger(String message, int lb, int ub, Scanner sc) {
/*
* This function will ask user for a positive integer
* If the user enters a valid input, the function will display
* an error message and reprompt
*/
int value;
while(true)
{
try{
System.out.print(message);
value = Integer.valueOf(sc.nextLine());
if(value >= lb && value <= ub) {
return value;
}
else {
System.out.println("Please enter a number between " + lb + " and " + ub + ".");
}
}
catch(Exception ex) {
System.out.println("Please enter a valid number.");
}
}
}
// Operations
static int getTotal(int[][] array)
{
/*
* This function calculates the sum of all values in the 2-D array
*/
int N = array.length;
int sum = 0;
for(int i = 0; i < N; i++)
{
for(int j = 0; j < N; j++)
{
sum += array[i][j];
}
}
return sum;
}
static double getAverage(int[][] array)
{
/*
* This function calculates the average of all values in the 2-D array
*/
int N = array.length;
int sum = getTotal(array);
double average = (double)sum/(N*N);
return average;
}
static int getRowTotal(int[][] array, int row)
{
/*
* Given the array, its size and the row id, this function calculates the sum of that row
*
*/
int N = array.length;
int sum = 0;
for(int column = 0; column < N; column++) {
sum += array[row][column];
}
return sum;
}
static int getHighestInRow(int[][] array, int row)
{
int N = array.length;
// Select the first element in the row as the highest
int highest = array[row][0];
for(int col = 1; col < N; col++) {
if(array[row][col] > highest) {
highest = array[row][col];
}
}
return highest;
}
static int getLowestInRow(int[][] array, int row)
{
int N = array.length;
// Select the first element in the row as the lowest
int lowest = array[row][0];
for(int col = 1; col < N; col++) {
if(array[row][col] < lowest) {
lowest = array[row][col];
}
}
return lowest;
}
static int getHighestInColumn(int[][] array, int column)
{
int N = array.length;
// Select the first element in the column as the highest
int highest = array[0][column];
for(int row = 1; row < N; row++) {
if(array[row][column] > highest) {
highest = array[row][column];
}
}
return highest;
}
static int getLowestInColumn(int[][] array, int column)
{
int N = array.length;
// Select the first element in the column as the lowest
int lowest = array[0][column];
for(int row = 1; row < N; row++) {
if(array[row][column] < lowest) {
lowest = array[row][column];
}
}
return lowest;
}
static int getColumnTotal(int[][] array, int col)
{
/*
* Given the array, its size and the column id, this function calculates the sum of that column
*
*/
int N = array.length;
int sum = 0;
for(int row= 0; row< N; row++) {
sum += array[row][col];
}
return sum;
}
public static void main(String[] args) throws Exception {
// Create Scanner
Scanner sc = new Scanner(System.in);
// create random object
Random random = new Random();
// Define size of array (we assumed it squared, so same number of rows and columns)
int N = 20;
// Create 2-D array to store data
int data[][] = new int[N][N];
// Initialize data with random integers between 1 and 100
for(int i = 0; i < N; i++)
{
for(int j = 0; j < N; j++)
{
data[i][j] = random.nextInt(100);
}
}
// Now, ask user for menu option
int option;
while(true)
{
printArray(data, N);
System.out.println("");
displayMenu();
// Ask for option
option = getInteger("Enter option: ", 1, 9, sc);
if(option == 1) // Calculate total
{
int total = getTotal(data);
System.out.println("The sum of all elements in the data is: " + total);
}
else if(option == 2) // average
{
double avg = getAverage(data);
System.out.println(String.format("The average of all values is: %.2f", avg));
}
else if(option == 3) { // row total
int row = getInteger("Enter row: ", 1, N, sc);
int total = getRowTotal(data, row-1);
System.out.println("The sum of all elements in row " + row + " is: " + total);
}
else if(option == 4) { // column total
int column= getInteger("Enter column: ", 1, N, sc);
int total = getColumnTotal(data, column-1);
System.out.println("The sum of all elements in column " + column + " is: " + total);
}
else if(option == 5) { // highest in row
int row = getInteger("Enter row: ", 1, N, sc);
int total = getHighestInRow(data, row-1);
System.out.println("The highest element in row " + row + " is: " + total);
}
else if(option == 6) { // lowest in row
int row = getInteger("Enter row: ", 1, N, sc);
int total = getLowestInRow(data, row-1);
System.out.println("The lowest element in row " + row + " is: " + total);
}
else if(option == 7) { // highest in column
int column = getInteger("Enter column: ", 1, N, sc);
int total = getHighestInColumn(data, column-1);
System.out.println("The highest element in column " + column + " is: " + total);
}
else if(option == 8) { // lowest in column
int column = getInteger("Enter column: ", 1, N, sc);
int total = getLowestInColumn(data, column-1);
System.out.println("The lowest element in column " + column + " is: " + total);
}
else if(option == 9) {
System.out.println("Good Bye!");
break;
}
}
}
}
Similar Samples
At Programming Homework Help, we provide top-quality assistance to help you tackle your programming challenges. Our samples showcase our expertise across various languages and projects, demonstrating our commitment to delivering accurate, timely, and effective solutions. Check out our samples and see how we can help you succeed.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java