Instructions
Objective
Write a python assignment program to print string after removing vowels.
Requirements and Specifications
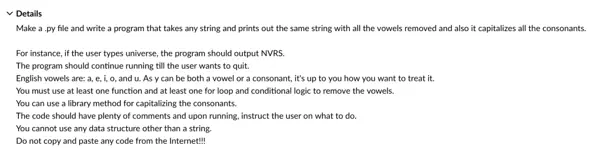
Source Code
def process_string(s):
"""
Method for processing given string.
Returns the same string with all the vowels removed and also capitalizes all the cononsnats
:param s: input string
:return: result of string processing
"""
# initializing result string with an empty string
result = ''
# iterating over all the characters of the given string
for c in s:
if c.isalpha():
# if character is a letter, not skipping it only if it is not a vowel
if c.lower() not in ['a', 'e', 'i', 'o', 'u']:
# if letter is not a vowel (consonant) - adding uppercased letter to the result
result += c.upper()
else:
# if character is not a letter, just keeping it in result string
result += c
# returning obtained result
return result
if __name__ == '__main__':
keep_asking = True
while keep_asking:
# prompting user to input a string
s1 = input('Please, enter a string to process: ')
# getting processed string
s2 = process_string(s1)
# outputting result
print('Result:', s2)
Related Samples
Explore our curated collection of expertly solved Python assignment samples. Whether you're tackling basic syntax or complex data structures, our samples showcase comprehensive solutions crafted by Python programming experts. Gain insights and inspiration for your projects.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python