Instructions
Objective
Write a python assignment program to measure lengths of time.
Requirements and Specifications
.webp)
Screenshots
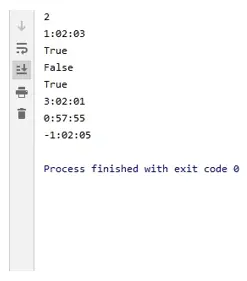
Source Code
class time:
hour = 0
minute = 0
second = 0
def __init__(self, hour, minute, second):
self.hour = hour
self.minute = minute
self.second = second
def __str__(self):
return str(self.hour) + ':' + '{:02d}'.format(self.minute) + ':' + '{:02d}'.format(self.second)
def __repr__(self):
return str(self)
def __eq__(self, other):
return self.hour == other.hour and self.minute == other.minute and self.second == other.second
def __le__(self, other):
if self.hour < other.hour:
return True
if self.hour > other.hour:
return False
if self.minute < other.minute:
return True
if self.minute > other.minute:
return False
return self.second <= other.second
def __lt__(self, other):
if self.hour < other.hour:
return True
if self.hour > other.hour:
return False
if self.minute < other.minute:
return True
if self.minute > other.minute:
return False
return self.second < other.second
def __add__(self, other):
s = (other.second + self.second) % 60
carry_s = (other.second + self.second) // 60
m = (other.minute + self.minute + carry_s) % 60
carry_m = (other.minute + self.minute + carry_s) // 60
h = other.hour + self.hour + carry_m
return time(h, m, s)
def __sub__(self, other):
s = (self.second - other.second + 60) % 60
carry_s = 0
if self.second < other.second:
carry_s = 1
m = (self.minute - other.minute - carry_s + 60) % 60
carry_m = 0
if self.minute < other.minute + carry_s:
carry_m = 1
h = other.hour - self.hour - carry_m
return time(h, m, s)
if __name__ == '__main__':
time1 = time(1, 2, 3)
print(time1.minute)
print(time1)
time2 = time(1, 59, 58)
print(time1 < time2)
print(time1 == time2)
print(time1 <= time2)
print(time1 + time2)
print(time2 - time1)
print(time1 - time2)
Similar Samples
Explore our curated collection of programming homework samples at ProgrammingHomeworkHelp.com. These examples showcase our proficiency in coding across multiple languages and complexities. Each sample reflects our dedication to delivering high-quality solutions tailored to academic requirements. Gain insights into our approach and discover how we can assist you with your programming assignments.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python