Instructions
Objective
We understand that starting with a new programming concept can be challenging, but don't worry, we're here to provide you assistance with python assignment you need. With our step-by-step guidance and examples, you'll be able to write a program that implements the Turtle library in Python in no time. Whether you're a beginner or just looking to expand your coding skills, we've got you covered. So, let's dive in and create some exciting graphical wonders with Python.
Requirements and Specifications
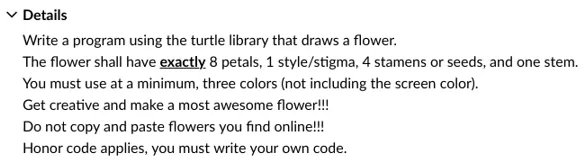
Source Code
import turtle as turtle
from math import pi, cos, sin
import random
# DEFINE HERE THE FLOWER COLORS
PETALS_COLOR = 'orange'
STIGMA_COLOR = 'brown'
SEEDS_COLOR = 'yellow'
STEM_COLOR = 'green'
def stem(pen):
"""
This function draws the flower stem
:param pen: Turtle pen
:return:
"""
pen.penup()
pen.goto(0,0)
pen.color(STEM_COLOR)
pen.fillcolor(STEM_COLOR)
pen.begin_fill()
# Now draw
pen.penup()
pen.goto(-15,0)
pen.right(90)
pen.pendown()
pen.begin_fill()
pen.forward(400)
pen.left(90)
pen.forward(30)
pen.left(90)
pen.forward(400)
pen.left(90)
pen.forward(30)
pen.end_fill()
pen.penup()
# Define the flower equation
def flower(n_petals: int, pen) -> None:
"""
This function draws a flower using a Polar equation.
According to [1], the flowers can be draw using a Mathematical Formula
in polar coordinates
[1] https://www.youtube.com/watch?v=Kq9nR_vZNJk
:param n_petals: Number of petals
:param pen: Turtle pen
:return:
"""
# Create angle theta
theta = []
theta0 = 0
for i in range(201):
theta.append(theta0)
theta0 += 2*pi/200.0
# Now, draw
pen.begin_fill()
pen.fillcolor(PETALS_COLOR)
pen.pencolor(PETALS_COLOR)
pen.pendown()
for th in theta:
r = 250*cos(n_petals//2 *th)
x = r*cos(th)
y = r*sin(th)
pen.setpos(x,y)
pen.end_fill()
pen.penup()
def stigma(pen):
"""
This function draws the flower's stigma at its center
:param pen: Turtle pen
:return:
"""
pen.penup()
pen.setpos(0,0)
pen.pencolor(STIGMA_COLOR)
pen.pendown()
# Radius of stigma
R = 50
theta = []
theta0 = 0
for i in range(201):
theta.append(theta0)
theta0 += 2 * pi / 200.0
pen.begin_fill()
pen.fillcolor(STIGMA_COLOR)
for th in theta:
x = R*cos(th)
y = R*sin(th)
pen.goto(x,y)
pen.end_fill()
def seeds(pen, n_seeds: int):
"""
This function draw the flower seeds
:param pen: Turtle pen
:param n_seeds: Number of seeds
:return:
"""
pen.pencolor(SEEDS_COLOR)
R = 50 # the radius of the stigma
theta = []
theta0 = 0
for i in range(201):
theta.append(theta0)
theta0 += 2 * pi / 200.0
# Now, select random positions inside the stigma
for _ in range(n_seeds):
# Pick a random size for the seed between 5 and 10
r = random.uniform(3,10)
#r = np.random.uniform(3, 10)
# Pick a random position inside the stigma
x = random.uniform(-R+r,R-r)
y = random.uniform(-R+r,R-r)
pen.penup()
pen.goto(x,y)
pen.pendown()
# Now, draw seed
pen.begin_fill()
pen.fillcolor(SEEDS_COLOR)
for th in theta:
xx = x+r*cos(th)
yy = y+r*sin(th)
pen.goto(xx,yy)
pen.end_fill()
pen.penup()
if __name__ == '__main__':
# Create turtle object
wn = turtle.Screen()
wn.screensize(500,500)
wn.bgcolor("white")
wn.title("Flower")
pen = turtle.Turtle()
pen.speed('fastest')
# Put pen at center of screen
pen.penup()
pen.setpos(0,0)
pen.pendown()
# Draw
stem(pen)
flower(8, pen)
stigma(pen)
seeds(pen, 4)
wn.exitonclick()
Related Samples
On ProgrammingHomeworkHelp.com, we offer a valuable resource for students tackling Python assignments. Our website provides expertly crafted sample solutions to guide you through complex Python problems. Whether you're struggling with data structures, algorithms, or advanced Python concepts, our detailed samples can help clarify difficult topics and improve your understanding. Each sample is designed to illustrate best practices and efficient coding techniques, making it easier for you to complete your assignments with confidence. Explore our Python samples to enhance your learning experience and achieve academic success.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python