Instructions
Objective
To enhance your understanding of data structures and programming in object-oriented languages, you have been tasked to write a Java assignment. Your assignment involves implementing a program to create a tree structure using the Java language. Trees are fundamental data structures that find applications in various domains of computer science and software development. By completing this assignment, you will not only grasp the concepts of tree structures but also strengthen your skills in Java programming.
Requirements and Specifications
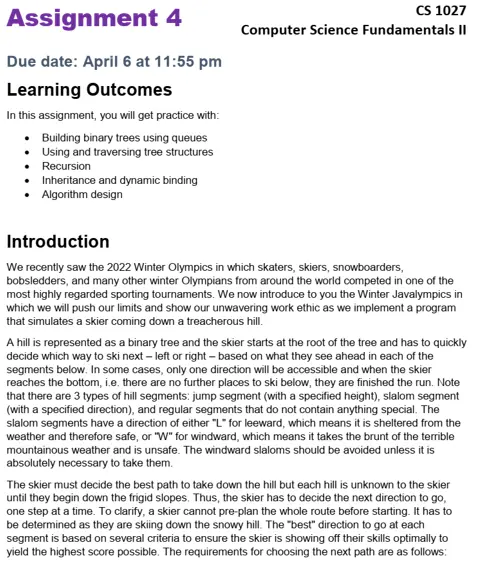
Source Code
public class Ski {
/**
* Ski mountain tree root node
*/
private BinaryTreeNode root;
public Ski(String[] data) {
// creating segment array of the same length as input data array
SkiSegment[] segments = new SkiSegment[data.length];
// iterating through input string array
for (int i = 0; i < data.length; i++) {
// if string value is null, segment is also null
if (data[i] == null) {
segments[i] = null;
} else if (data[i].contains("jump")) {
// if string data contains "jump", we create jump segment
segments[i] = new JumpSegment(String.valueOf(i), data[i]);
} else if (data[i].contains("slalom")) {
// if string data contains "slalom", we create slalom segment
segments[i] = new SlalomSegment(String.valueOf(i), data[i]);
} else {
// otherwise, we create regular ski segment
segments[i] = new SkiSegment(String.valueOf(i), data[i]);
}
}
// creating tree with tree builder and getting root of created tree
root = new TreeBuilder().buildTree(segments).getRoot();
}
/**
* Getter method for root field of class
* @return root node of ski mountatin tree
*/
public BinaryTreeNode getRoot() {
return root;
}
/**
* Method for processing given mountain tree node and choosing the branch to continue, if there is
* @param node current mountain tree node
* @param sequence ski segments chosen so far
*/
public void skiNextSegment(BinaryTreeNode node, ArrayUnorderedList sequence) {
// adding current segment to path sequence
sequence.addToRear(node.getData());
// getting left child node of current one
BinaryTreeNode left = node.getLeft();
// getting right child node of current one
BinaryTreeNode right = node.getRight();
// this variable will store the next chosen node
BinaryTreeNode chosen = null;
// processing case, when both children exist
if (left != null && right != null) {
// if both segments are jump segments, choosing the one with greater height.
// if both heights are the same, choosing right one
if (left.getData() instanceof JumpSegment && right.getData() instanceof JumpSegment) {
int leftHeight = ((JumpSegment) left.getData()).getHeight();
int rightHeight = ((JumpSegment) right.getData()).getHeight();
if (leftHeight > rightHeight) {
chosen = left;
}
else {
chosen = right;
}
}
// if there is only one jump segment, choosing it
else if (left.getData() instanceof JumpSegment) {
chosen = left;
}
else if (right.getData() instanceof JumpSegment) {
chosen = right;
}
// processing case, when both segments are slalom segments
else if (left.getData() instanceof SlalomSegment && right.getData() instanceof SlalomSegment) {
// choosing L-segment
if (((SlalomSegment) left.getData()).getDirection().equals("L")) {
chosen = left;
}
else {
chosen = right;
}
}
// processing case, when one segment is slalom and one is regular
else if (left.getData() instanceof SlalomSegment) {
// choosing slalom segment only if it is L
if (((SlalomSegment) left.getData()).getDirection().equals("L")) {
chosen = left;
}
else {
chosen = right;
}
}
else if (right.getData() instanceof SlalomSegment) {
// choosing slalom segment only if it is L
if (((SlalomSegment) right.getData()).getDirection().equals("L")) {
chosen = right;
}
else {
chosen = left;
}
}
else {
// if both segments are regular, choosing the right one
chosen = right;
}
} else if (left != null) {
// if there is only left child, choosing it
chosen = left;
} else if (right != null) {
// if there is only right child, choosing it
chosen = right;
}
// if any node was chosen, continue sequence with chosen node by calling method recursively
if (chosen != null) {
skiNextSegment(chosen, sequence);
}
}
}
Similar Samples
Explore our diverse collection of programming samples at ProgrammingHomeworkHelp.com. Our curated examples span various languages and difficulty levels, showcasing our expertise in solving coding challenges. Whether you're working on basic assignments or complex projects, these samples demonstrate clarity, efficiency, and adherence to academic standards. Dive into our repository to see how we can assist you in mastering programming concepts effectively.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java