Instructions
Objective
Write a c++ assignment program to implement temperature statistics.
Requirements and Specifications
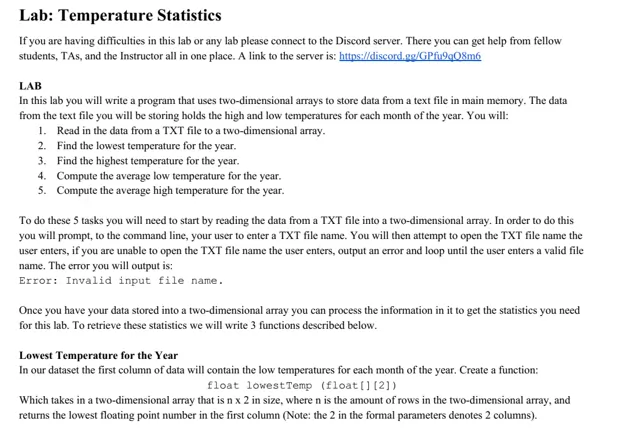
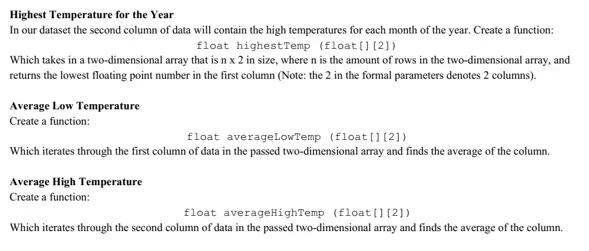
Source Code
#include
#include
#include
#include
#include
#include
using namespace std;
// Function prototypes
float lowestTemp(float temperatures[][2]);
float highestTemp(float temperatures[][2]);
float averageLowTemp(float temperatures[][2]);
float averageHighTemp(float temperatures[][2]);
// Entry point of the program
int main()
{
cout << "TEMPERATURE STATISTICS" << endl;
// Open file for reading and loading of temperature
ifstream inFile;
while (true)
{
cout << "What is the data file name?" << endl;
cout << "**";
string filename;
getline(cin, filename);
inFile.open(filename.c_str());
if (inFile.is_open())
break;
cout << "Error: Invalid input file name." << endl;
}
cout << endl;
// Read the title
string line;
getline(inFile, line);
cout << line << endl;
cout << endl;
// Load the temperatures into an array, 12 months
float temperatures[12][2];
for (int i = 0; i < 12; i++)
{
getline(inFile, line);
stringstream ss(line);
ss >> temperatures[i][0];
ss >> temperatures[i][1];
}
inFile.close();
// Report statistics
char degreeSign = '\370';
cout << "Lowest temperature of the year was " << lowestTemp(temperatures) << degreeSign << " F." << endl;
cout << "Highest temperature of the year was " << highestTemp(temperatures) << degreeSign << " F." << endl;
cout << "Average low temperature of the year was " << averageLowTemp(temperatures) << degreeSign << " F." << endl;
cout << "Average high temperature of the year was " << averageHighTemp(temperatures) << degreeSign << " F." << endl;
return 0;
}
// Find the lowest temperature
float lowestTemp(float temperatures[][2])
{
float lowest = 0;
for (int i = 0; i < 12; i++)
if (i == 0 || temperatures[i][0] < lowest)
lowest = temperatures[i][0];
// Round to 2 decimal places
lowest = ceil(lowest * 100.0f) / 100.0f;
return lowest;
}
// Find the highest temperature
float highestTemp(float temperatures[][2])
{
float highest = 0;
for (int i = 0; i < 12; i++)
if (i == 0 || temperatures[i][1] > highest)
highest = temperatures[i][1];
// Round to 2 decimal places
highest = ceil(highest * 100.0f) / 100.0f;
return highest;
}
// Return the average low temperature
float averageLowTemp(float temperatures[][2])
{
float total = 0;
for (int i = 0; i < 12; i++)
total += temperatures[i][0];
float average = total / 12;
// Round to 2 decimal places
average = ceil(average * 100.0f) / 100.0f;
return average;
}
// Return the average high temperature
float averageHighTemp(float temperatures[][2])
{
float total = 0;
for (int i = 0; i < 12; i++)
total += temperatures[i][1];
float average = total / 12;
// Round to 2 decimal places
average = ceil(average * 100.0f) / 100.0f;
return average;
}
Related Samples
Explore our free C++ assignment samples to gain a clear perspective on programming concepts. These samples provide detailed solutions and practical examples, showcasing various C++ applications and techniques. Whether you're working on basic syntax or complex algorithms, our samples offer valuable insights and clarity, making it easier to approach your C++ assignments with confidence.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++