Instructions
Objective
If you need assistance with a Java assignment, to write a program to implement a table pattern in Java. In this program, we will use loops to create a table-like pattern using asterisks or any other symbol of your choice. By defining the rows and columns, we can easily generate a visually appealing pattern that resembles a table. Java provides powerful control structures that make it relatively simple to accomplish this task.
Requirements and Specifications
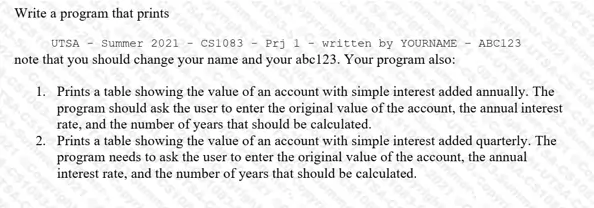
Source Code
import java.util.Scanner;
public class Tables {
// Constants
private static final Scanner CONSOLE = new Scanner(System.in);
// Entry point of the program
public static void main(String[] args) {
System.out.println("UTSA - Summer 2021 - CS1083 - Prj 1 - written by YOURNAME - ABC123");
System.out.println();
// Ask for the inputs for calculation
System.out.print("Enter original amount: ");
double principalAmount = CONSOLE.nextDouble();
System.out.print("Enter annual interest rate: ");
double annualInterestRate = CONSOLE.nextDouble();
System.out.print("Enter years: ");
int years = CONSOLE.nextInt();
// Calculate the annual interest to pay
System.out.println();
System.out.println("Year\tValue");
for (int year = 0; year <= years; year++) {
double value = principalAmount * (1 + year * annualInterestRate / 100);
System.out.print(year + "\t");
System.out.printf("%.2f", value);
System.out.println();
}
System.out.println();
// Ask for the inputs for calculation
System.out.print("Enter original amount: ");
principalAmount = CONSOLE.nextDouble();
System.out.print("Enter annual interest rate: ");
annualInterestRate = CONSOLE.nextDouble();
System.out.print("Enter years: ");
years = CONSOLE.nextInt();
// Calculate the quarterly interest to pay
System.out.println();
System.out.println("Year\tQ1 Value\tQ2 Value\tQ3 Value\tQ4 Values");
for (int year = 1; year <= years; year++) {
System.out.print(year + "\t");
// Calculate each quarter for the year
for (int quarter = 1; quarter <= 4; quarter++) {
double value = principalAmount * (1 + (year - 1 + quarter / 4.0) * annualInterestRate / 100);
System.out.printf("%.2f\t\t", value);
}
System.out.println();
}
}
}
Related Samples
See our free Java assignment samples for clarity on various concepts and techniques. Our samples provide detailed solutions and practical examples, helping you navigate through complex Java topics. Whether you're working on basic syntax or advanced programming, these resources are designed to support your learning process and academic success.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java