Instructions
Objective
Write a C++ assignment program to implement story game.
Requirements and Specifications
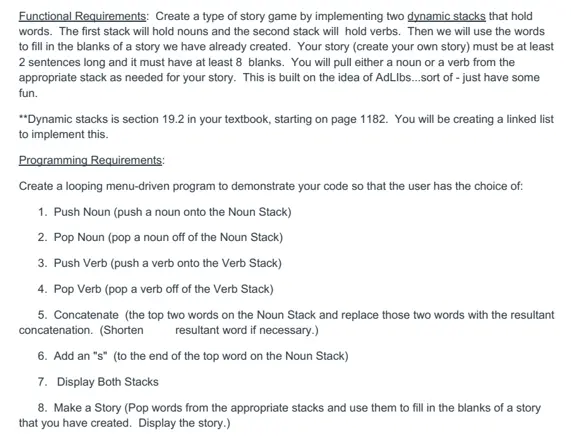
.webp)
Source Code
#ifndef wordstack_h
#define wordstack_h
#include
#include
using namespace std;
// A stack that holds words
class WordStack
{
private:
// Node to be used to building a linked list of stack
struct Node
{
string word;
Node *next;
// Create a new node that holds a word
Node(const string &word)
{
this->word = word;
next = NULL;
}
};
Node *top;
int numWords;
public:
// Create a stack
WordStack()
{
top = NULL;
numWords = 0;
}
// Destructor to delete nodes if there are still left
~WordStack()
{
Node *current = top;
while (current != NULL)
{
Node *next = current->next;
delete current;
current = next;
}
}
// Add a new word to the stack
void push(const string &word)
{
Node *node = new Node(word);
node->next = top;
top = node;
numWords++;
}
// Remove the top element and return it
string pop()
{
if (top == NULL)
return "";
string word = top->word;
top = top->next;
numWords--;
return word;
}
// Return the number of elements
int size()
{
return numWords;
}
// Check if stack is empty
bool isEmpty()
{
return numWords == 0;
}
// Print the stack to the output stream
friend ostream& operator << (ostream &out, const WordStack &stack)
{
Node *current = stack.top;
while (current != NULL)
{
out << current->word;
current = current->next;
if (current != NULL)
out << ", ";
}
return out;
}
}
#endif
Similar Samples
Discover our diverse range of programming homework samples at ProgrammingHomeworkHelp.com. From introductory exercises to advanced projects in languages like Java, Python, and more, our examples showcase our expertise and dedication to academic excellence. Explore how our solutions can help you understand and excel in programming concepts effectively.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++