Tip of the day
News
Instructions
Objective
Write a C++ assignment program to implement sorting techniques.
Requirements and Specifications
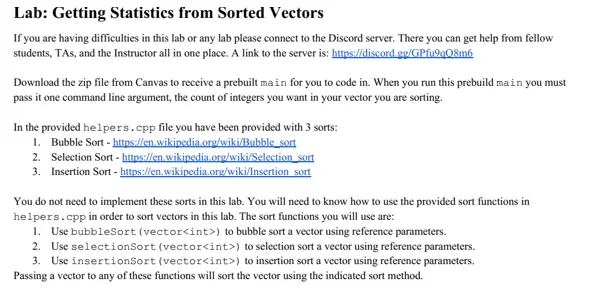
Source Code
/*
* Name:
* Description:
* Input:
* Output:
*/
#include
#include
#include "helpers.cpp"
using namespace std;
//---------- YOUR FUNCTIONS HERE ----------
// minimum, maximum, sum, average, reverseVector
/*
* minimum: Finds the minimum in a vector of ints
* parameters: Vector to find minimum of
* return value: Minimum of vector
*/
int minimum(const vector &elements)
{
return elements.front();
}
/*
* maximum: Finds the maximum in a vector of ints
* parameters: Vector to find max of
* return value: Maximum of vector
*/
int maximum(const vector &elements)
{
return elements.back();
}
/*
* sum: Sums a vector of ints
* parameters: Vector to find sum of
* return value: Sum of vector
*/
int sum(const vector &elements)
{
int total = 0;
for (unsigned i = 0, numElements = elements.size(); i < numElements; i++)
total += elements[i];
return total;
}
/*
* average: Finds the average of a vector of ints
* parameters: Vector to find average of
* return value: Average of vector
*/
double average(const vector &elements)
{
return (double)sum(elements) / elements.size();
}
/*
* reverseVector: Reverses a vector of ints
* parameters: Vector to reverse
* return value: Reverse of vector
*/
void reverseVector(vector &elements)
{
int i = 0;
int j = (int)(elements.size() - 1);
while (i < j)
{
int temp = elements[i];
elements[i] = elements[j];
elements[j] = temp;
i++;
j--;
}
}
//---------- END YOUR FUNCTIONS ----------
int main(int argc, char const *argv[]) {
if (argc == 1) cout << "Usage: ./a.out \n";
else if (argc != 2) cout << "Error: Invalid argument count.\n";
else {
int wantedSize = atoi(argv[1]);
//------- SECTION 1 BUBBLE SORT -------
cout << "BUBBLE SORT" << endl;
vector list = createVector(wantedSize);
cout << "Unsorted: ";
printVector(list);
chrono::steady_clock::time_point start = chrono::steady_clock::now();
// YOUR CODE HERE
// Bubble Sort, Statistics, Reverse
// Sort the vector (1)
bubbleSort(list);
cout << "Sorted: ";
printVector(list);
// Statistics on the vector (2-5)
cout << "Minimum: " << minimum(list) << endl;
cout << "Maximum: " << maximum(list) << endl;
cout << "Sum: " << sum(list) << endl;
cout << "Average: " << average(list) << endl;
// Reverse the vector (6)
reverseVector(list);
cout << "Reverse: ";
printVector(list);
cout << endl;
// END OF YOUR CODE
chrono::steady_clock::time_point end = chrono::steady_clock::now();
cout << "Elapsed time in seconds: "
<< chrono::duration_cast(end - start).count()
<< " microseconds.\n\n";
//------- SECTION 2 SELECTION SORT -------
cout << "SELECTION SORT" << endl;
list = createVector(wantedSize);
cout << "Unsorted: ";
printVector(list);
start = chrono::steady_clock::now();
// YOUR CODE HERE
// Selection Sort, Statistics, Reverse
// Sort the vector (1)
selectionSort(list);
cout << "Sorted: ";
printVector(list);
// Statistics on the vector (2-5)
cout << "Minimum: " << minimum(list) << endl;
cout << "Maximum: " << maximum(list) << endl;
cout << "Sum: " << sum(list) << endl;
cout << "Average: " << average(list) << endl;
// Reverse the vector (6)
reverseVector(list);
cout << "Reverse: ";
printVector(list);
cout << endl;
// END OF YOUR CODE
end = chrono::steady_clock::now();
cout << "Elapsed time in seconds: "
<< chrono::duration_cast(end - start).count()
<< " microseconds.\n\n";
//------- SECTION 3 INSERTION SORT -------
cout << "INSERTION SORT" << endl;
list = createVector(wantedSize);
cout << "Unsorted: ";
printVector(list);
start = chrono::steady_clock::now();
// YOUR CODE HERE
// Insertion Sort, Statistics, Reverse
// Sort the vector (1)
insertionSort(list);
cout << "Sorted: ";
printVector(list);
// Statistics on the vector (2-5)
cout << "Minimum: " << minimum(list) << endl;
cout << "Maximum: " << maximum(list) << endl;
cout << "Sum: " << sum(list) << endl;
cout << "Average: " << average(list) << endl;
// Reverse the vector (6)
reverseVector(list);
cout << "Reverse: ";
printVector(list);
cout << endl;
// END OF YOUR CODE
end = chrono::steady_clock::now();
cout << "Elapsed time in seconds: "
<< chrono::duration_cast(end - start).count()
<< " microseconds.\n\n";
}
return 0;
}
Similar Sample
Explore our C++ assignment sample to see the quality and depth of our work. This example showcases our expertise in C++ programming, providing clear, well-commented code solutions and thorough explanations. Trust Programming Homework Help to deliver top-notch assistance for your C++ projects and assignments.
C++
Word Count
6780 Words
Writer Name:Alexander Gough
Total Orders:2435
Satisfaction rate:
C++
Word Count
6185 Words
Writer Name:Neven Bell
Total Orders:2376
Satisfaction rate:
C++
Word Count
5749 Words
Writer Name:Professor Kevin Tan
Total Orders:569
Satisfaction rate:
C++
Word Count
4786 Words
Writer Name:Dr. Isabelle Taylor
Total Orders:580
Satisfaction rate:
C++
Word Count
3997 Words
Writer Name:Professor Oliver Bennett
Total Orders:304
Satisfaction rate:
C++
Word Count
6830 Words
Writer Name:Dr. Maddison Todd
Total Orders:865
Satisfaction rate:
C++
Word Count
4424 Words
Writer Name:Dr. Alexandra Mitchell
Total Orders:589
Satisfaction rate:
C++
Word Count
7250 Words
Writer Name:Mr. Harrison Yu
Total Orders:311
Satisfaction rate:
C++
Word Count
4894 Words
Writer Name:James Miller
Total Orders:824
Satisfaction rate:
C++
Word Count
5544 Words
Writer Name:Callum Chambers
Total Orders:932
Satisfaction rate:
C++
Word Count
3533 Words
Writer Name:Aaliyah Armstrong
Total Orders:658
Satisfaction rate:
C++
Word Count
3645 Words
Writer Name:Len E. Villasenor
Total Orders:489
Satisfaction rate:
C++
Word Count
4033 Words
Writer Name:Daniel M. Harrison
Total Orders:700
Satisfaction rate:
C++
Word Count
3576 Words
Writer Name:Dr. Isabella Wong
Total Orders:652
Satisfaction rate:
C++
Word Count
3600 Words
Writer Name:Prof. Liam Wilson
Total Orders:554
Satisfaction rate:
C++
Word Count
1035 Words
Writer Name:Professor Marcus Tan
Total Orders:544
Satisfaction rate:
C++
Word Count
6288 Words
Writer Name:Dr. Stephanie Kim
Total Orders:812
Satisfaction rate:
C++
Word Count
1586 Words
Writer Name:Ethan Patel
Total Orders:556
Satisfaction rate:
C++
Word Count
3852 Words
Writer Name:Sarah Rodriguez
Total Orders:689
Satisfaction rate:
C++
Word Count
4701 Words
Writer Name:Harry F. Grimmett
Total Orders:342
Satisfaction rate: