Instructions
Objective
Write a java assignment program to implement shapes and colors.
Requirements and Specifications
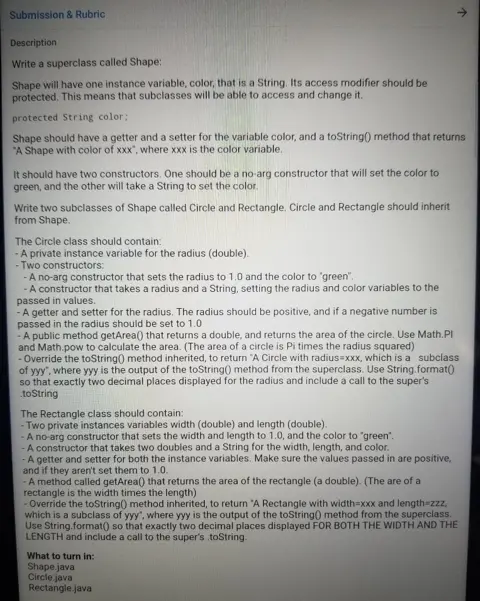
Source Code
CIRCLE
public class Circle extends Shape {
private double radius;
// Default constructor
public Circle() {
super(); // calling the super-class default constructor initializes the color to 'green'
radius = 1.0;
}
// Overloaded constructor
public Circle(double rad, String col) {
super(col); // call super-class constructor with the given color
setRadius(rad);
}
// getters and setters
public double getRadius() {return radius;}
public void setRadius(double new_radius) {
if(new_radius < 0) // if the given radius is negative, set it to 1.0
{
new_radius = 1.0;
}
radius = new_radius;
}
// methods
public double getArea() {
return Math.PI*Math.pow(getRadius(), 2);
}
@Override
public String toString() {
return String.format("A Circle with radius=%.2f, which is a subclass of %s", getRadius(), super.toString());
}
}
DRIVER
public class Driver {
public static void main(String[] args) throws Exception {
// Create a red Circle with radius -1
Circle circle = new Circle(-1, "red");
// If we print it, it should display a radius of 1.0 not -1
System.out.println("This circle is " + circle.getColor()); // print color
System.out.println(circle.toString());
System.out.println(String.format("Area: %.2f", circle.getArea()));
System.out.println("");
// Create a yellow circle of radius 2
circle = new Circle(2.0, "yellow");
System.out.println("This circle is " + circle.getColor()); // print color
System.out.println(circle.toString());
System.out.println(String.format("Area: %.2f", circle.getArea()));
System.out.println("");
// Rectangles
// Create a rectangle with width = 5 and length = 2
Rectangle rect = new Rectangle(5.0,2.0,"black");
System.out.println(rect.toString());
System.out.println(String.format("Area: %.2f", rect.getArea()));
System.out.println("");
// Create a rectangle with negative values. Both its width and length should be setted to 1
rect = new Rectangle(-100, -500, "white");
System.out.println(rect.toString());
System.out.println("");
}
}
RECTANGLE
import java.lang.ref.WeakReference;
import javax.sql.rowset.WebRowSet;
public class Rectangle extends Shape {
private double width;
private double length;
// No-arg constrcutor
public Rectangle() {
super();
width = 1.0;
length = 1.0;
}
// Overloaded constructor
public Rectangle(double w, double l, String col) {
super(col);
setWidth(w);
setLength(l);
}
// getters and setters
public double getWidth() {
return width;
}
public void setWidth(double w) {
if(w < 0.0) {
w = 1.0;
}
width = w;
}
public double getLength() {
return length;
}
public void setLength(double l) {
if(l < 0.0) {
l = 1.0;
}
length = l;
}
// methods
public double getArea() {return getWidth()*getLength();}
@Override
public String toString(){
return String.format("A Rectangle with width=%.2f and length=%.2f, which is a subclass of %s", getWidth(), getLength(), super.toString());
}
}
SHAPE
public class Shape
{
protected String color;
// default constructor
public Shape() {
color = "green";
}
// Overloaded constructor
public Shape(String col) {
color = col;
}
// getter and setter
public void setColor(String new_color) { color = new_color;}
public String getColor() {return color;}
// toString
@Override
public String toString() {
return "A Shape with color " + getColor();
}
}
Similar Samples
Welcome to ProgrammingHomeworkHelp.com, your trusted partner for all programming assignments. We offer expert assistance in languages like C++, Python, Java, and more. With a team of experienced programmers, we deliver precise solutions tailored to your requirements. Whether you're a student or a professional, count on us for reliable, on-time delivery and top-notch quality. Excelling in programming has never been easier—explore our services today!
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java