Instructions
Objective
Writing a program to implement sets in C++ can be a valuable learning experience. Sets are containers that store unique elements in no particular order, making them useful for various applications. By utilizing the standard template library (STL) in C++, you can easily create and manipulate sets. If you need help with your C++ assignment focused on set implementation or any related concepts, feel free to ask for guidance and clarification.
Requirements and Specifications
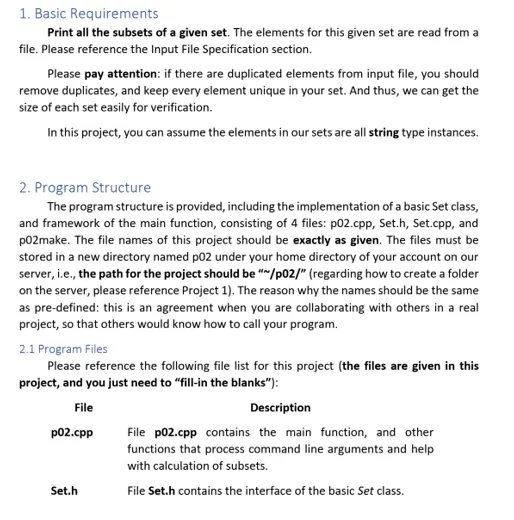
Source Code
#include
#include
#include
#include
#include
#include "Set.h"
#include
#ifndef MAX_FILE_LENGTH
#define MAX_FILE_LENGTH 255
#endif // MAX_FILE_LENGTH
using namespace std;
/**
* Read the input set from the input stream, and output the subsets
* to the output stream.
*/
void get_subsets(istream& i1, ostream& o)
{
Set set1;
set1.scan(i1); // read the set from the input file
set1.print(o, "A="); // print the set to the output file
/* Please put your code here. */
o << "Subsets of A:" << std::endl;
// Loop from 0 to 2^n-1 where n is the size of the set
int n = set1.getsize();
int n_subsets = pow(2, n);
for(int i = 0; i < n_subsets; i++) {
o << "{";
for(int j = 0; j < n; j++) {
if(i & (1 << j)) { // binary operator
o << set1.getelements()[j];
if(j < n-1) {
o << ",";
}
}
}
o << "}";
if(i < n_subsets-1) {
o << ",";
}
else {
o << ".";
}
o << std::endl;
}
// Finally, write the number of subsets
o << "There are " << pow(2, set1.getsize()) << " subsets of A.";
// TODO: get all the subsets of the input set and print the result
}
/*
* This program accepts three command line arguments:
* argv[1] is for the path to input file
* argv[2] is for the path to the output file
*/
int main(int argc, char* argv[])
{
char file1[MAX_FILE_LENGTH], file2[MAX_FILE_LENGTH];
// file1 is for the input, file2 is for the output
strcpy(file1, argv[1]);
strcpy(file2, argv[2]);
ifstream i1 (file1);
ofstream o (file2);
if (i1.is_open() && o.is_open()) {
get_subsets(i1, o);
i1.close();
o.close();
}
else {
cout << "Unable to open the file(s)." << endl;
}
}
Related Samples
Explore our comprehensive Data Structures and Algorithms assignment samples to enhance your understanding. These expertly crafted examples cover a wide range of topics, offering clear explanations and practical solutions to complex problems. Perfect for students aiming to excel in their coursework.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++