Instructions
Objective
Write a python assignment program to implement regexinator plus.
Requirements and Specifications
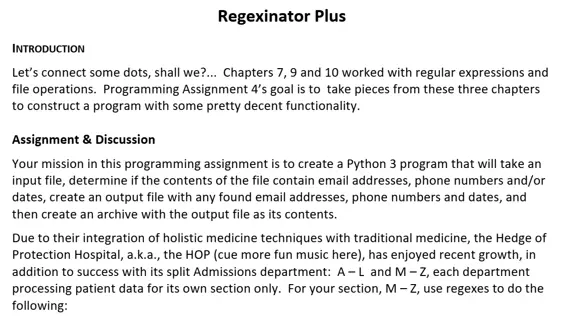
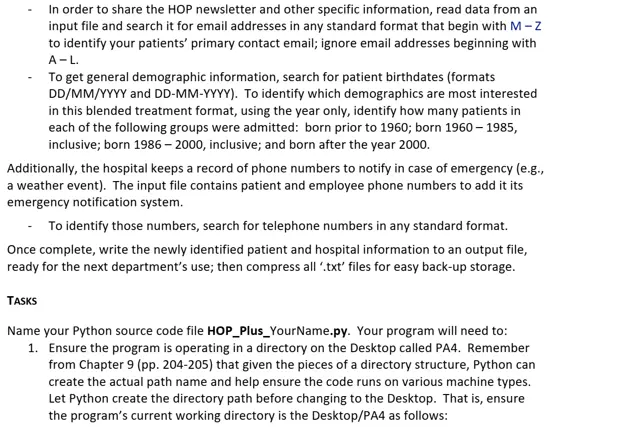
Source Code
# U.R. Name
# AIST 2120: Programming Assignment 4 #! Python 3
# Submission D
import re
import os
import sys
if __name__ == '__main__':
# Define the folder
homeFolder = "C:\\"
userDir = "Eduardo"
desktopPath = os.path.join(homeFolder, 'Users', userDir, 'Desktop', 'PA4')
os.chdir(desktopPath)
# Define here the input filename
INPUT_FILENAME = "AdminIn.txt"
# Define the regex for emails
email_regex = r'([M-Zm-z][A-Za-z0-9]+@[A-Za-z]+(\.com|\.org))'
dates_regex = r'([1-9]|0[1-9]|[12][0-9]|3[01])(/|-)([1-9]|0[1-9]|1[012])(/|-)(19|20)\d\d'
phone_regex = r'(\d{3}[-\.\s]??\d{3}[-\.\s]??\d{4}|\(\d{3}\)\s*\d{3}[-\.\s]??\d{4}|\d{3}[-\.\s]??\d{4})'
# Create lists to store emails, dates and phones
emails = []
dates = []
phones = []
"""
PRINT HEADER
"""
print("{:>50s}".format('-'*43))
print("{:>10s}{:>20s}{:>20s}".format("---", "AIST 2120","---"))
print("{:>10s}{:>30s}{:>10s}".format("---", "Programming Assignment 4", "---"))
print("{:>50s}".format('-'*43))
print()
print("{:>2s}{:>45}{:>5s}".format("***", "WELCOME to the HEDGE OF PROTECTION HOSPITAL", "***"))
print("{:>4s}{:>43s}{:>5s}".format("***", "ADMISSIONS DEPARTMENT: M - Z Patient Data", "***"))
print("{:>5s}{:>35s}{:>10s}".format("***", '*'*30, "***"))
print()
"""
PRINT INFO
"""
print(f"The input filename is /{INPUT_FILENAME}/")
print()
print("An output file name for storing patient email and birthdate information, along\nwith available hospital phone numbers, is needed.")
output_filename = input("Please enter a file name ending in .txt: ")
print()
print(f"Admin section's M - Z output filename is /{output_filename}/")
print()
print()
# Open file
with open(INPUT_FILENAME, 'r') as f:
# Read lines
lines = f.readlines()
# Loop through lines
for line in lines:
# Find emails
match = re.search(email_regex, line)
if match != None:
emails.append(match.group())
match = re.search(dates_regex, line)
if match != None:
dates.append(match.group())
match = re.search(phone_regex, line)
if match != None:
phones.append(match.group())
# Check for emails
if len(emails) > 0:
print("Patient emails to be added to the HOP newsletter distribution are:")
print('[\t', end='')
for email in emails:
print(f"\t'{email}'\n",end='')
print(']\n',end='')
else:
print("Admission Section M - Z has no new patients, therefore no patient EMAILS pending at this time.")
print()
# Check of BIRTHDATES
if len(dates) > 0:
print("Basic age demographics for new M = Z admissions are as follow:")
print('[\t', end='')
for date in dates:
print(f"\t'{date}'\n", end='')
print(']\n', end='')
else:
print("No new patient BIRTHDATES are available at this time.")
print()
# Check for phones
if len(phones) > 0:
print("The new PHONE NUMBERS for adding to the emergency notification system are:")
print('[\t', end='')
for phone in phones:
print(f"\t'{phone}'\n", end='')
print(']\n', end='')
else:
print("No new PHONE NUMBERS are available for adding to the emergency notification system.")
print()
# Check if the file has to be created
if len(emails) > 0 or len(dates) > 0 or len(emails) > 0:
with open(output_filename, 'w+') as fo:
for phone in phones:
fo.write(phone + '\n')
for email in emails:
fo.write(email + '\n')
for date in dates:
fo.write(date + '\n')
else:
print("No new HOP Admin M - Z section matches were found, so NO OUTPUT file was created!")
print()
print("*** Processing of ADMISSIONS DEPARTMENT (M - Z) data is COMPLETE ***")
input("Press any key to exit.")
sys.exit(0)
"""
# Now, display
print(emails)
print(phones)
print(dates)
line = 'maria is aerewrfmaria@gmail.comwefewf aaa'
line2 = 'qj9paocivjczpoxic10/31/2021ajsidjvojpcxozijc;lkekjlmnfer'
line3 = 'gsdf800-567-4566asdgsdf800-567-4566asd'
matched = bool(re.match(phone_regex, line3))
if matched:
print("Found")
else:
print("Not found")
print(re.search(dates_regex, line2))
"""
Similar Samples
Discover our expertise through a collection of programming homework samples at ProgrammingHomeworkHelp.com. Our samples span various languages and difficulty levels, showcasing our proficiency in delivering high-quality solutions. Whether you're tackling algorithms, data structures, or web development tasks, our samples demonstrate our commitment to excellence in programming assistance.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python