Instructions
Objective
Write a java assignment program to implement recursion.
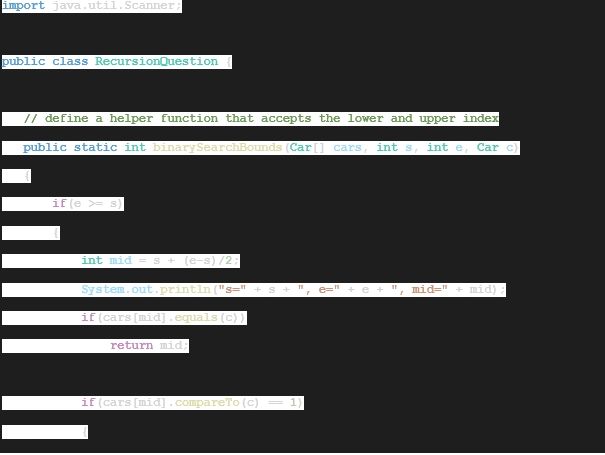
Source Code
ANNOUNCEMENT
interface Announcements {
String departure() ;
String arrival();
String doNotDisturbTheDriver();
}
BICYCLE
public class Bicycle extends Vehicle implements Comparable {
private double weight;
private static double ACCURACY_WEIGHT = 0.5;
public Bicycle()
{
super(1, 1);
weight = 0;
}
public Bicycle(Person driver)
{
super(1,1);
personsOnBoard[0][0] = driver;
weight = 0;
}
public Bicycle(Person driver, double weight)
{
super(1,1);
personsOnBoard[0][0] = driver;
if(weight < 0)
weight = 0;
this.weight = weight;
}
public double getWeight() {return weight;}
public void setWeight(double w) {
if(w < 0)
w = 0;
weight = w;
}
@Override
public boolean equals(Object o)
{
if(!(o instanceof Bicycle))
return false;
Bicycle other = (Bicycle)o;
double diff = Math.abs(getWeight() - other.getWeight());
return diff <= ACCURACY_WEIGHT;
}
@Override
public void setDriver(Person p) throws InvalidDriverException
{
if(p.getAge() >= 3)
personsOnBoard[0][0] = p;
else
throw new InvalidDriverException();
}
@Override
public int compareTo(Bicycle o) {
// TODO Auto-generated method stub
if(getWeight() + ACCURACY_WEIGHT < o.getWeight())
return -1;
else if(getWeight() > o.getWeight() + ACCURACY_WEIGHT)
return 1;
return 0;
}
@Override
public boolean loadPassenger(Person p) {
// TODO Auto-generated method stub
return false;
}
@Override
public int loadPassengers(Person[] peeps) {
// TODO Auto-generated method stub
return 0;
}
@Override
public String toString()
{
return "Bicycle [ rider= " + getDriver().getName() + " | weight= " + weight + " ]";
}
}
BUS
public class Bus extends Car {
public Bus(int[] numSeatsPerRow)
{
super(2, 2*numSeatsPerRow.length-1, numSeatsPerRow);
}
public Bus(Person driver, int[] numSeatsPerRow) {
super(2, 2*numSeatsPerRow.length-1, driver, numSeatsPerRow);
}
public boolean canOpenDoor(Person p)
{
if(getDriver().equals(p)) // person is the driver
return true;
if(p.getAge() <= 5 || p.getHeight() <= 40) // not above 5 years or not above 40 inches
return false;
// get the index of the last populated row
int rowIdx = -1;
for(int i = 0; i < getNumberOfRows(); i++)
{
if(getNumberOfPeopleInRow(i) == 0)
{
rowIdx = i - 1;
break;
}
}
if(rowIdx < 0) // no persons in row
return false;
// check if the person is not in the last populated row
int[] pos = getLocationOfPersonInVehicle(p);
if(pos[0] == rowIdx)
return true;
return false;
}
public boolean canOpenWindow(Person p)
{
return super.canOpenWindow(p) && p.getAge() > 5;
}
@Override
public String toString()
{
return "Bus is an extension of " + super.toString();
}
@Override
public boolean loadPassenger(Person p) {
// TODO Auto-generated method stub
// assign passenger to the first available seat
if(getNumberOfAvailableSeats() < 1)
return false;
for(int i = 0; i < getNumberOfRows(); i++)
{
for(int j = 0; j < getNumberOfSeatsPerRow()[i]; j++)
{
if(getPersonInSeat(i, j) == null) // no person in this seat
{
setPassenger(p, i, j);
return true;
}
}
}
return false;
}
@Override
public int loadPassengers(Person[] peeps) {
// TODO Auto-generated method stub
int loaded = 0;
for(Person p: peeps)
{
if(loadPassenger(p))
loaded++;
}
return loaded;
}
public String departure() {return super.departure() + "The Bus\n";};
public String arrival() {return super.arrival() + "Of The Bus\n";};
public String doNotDisturbTheDriver() {return super.doNotDisturbTheDriver() + "On The Bus\n";};
}
CAR
public class Car extends Vehicle implements Comparable, Announcements {
private int numDoors;
private int numWindows;
public String departure() {return "All Aboard\n";};
public String arrival() {return "Everyone Out\n";};
public String doNotDisturbTheDriver() {return "No Backseat Driving\n";};
// getters
public int getNumDoors() {return numDoors;}
public int getNumWindows() {return numWindows;}
public Car(int numDoors, int numWindows)
{
super(2, 2);
this.numDoors = numDoors;
this.numWindows = numWindows;
}
public Car(int numDoors, int numWindows, int numSeatsPerRow)
{
super(2, numSeatsPerRow);
this.numDoors = numDoors;
this.numWindows = numWindows;
}
public Car(int numDoors, int numWindows, int[] numSeatsPerRow)
{
super(numSeatsPerRow);
this.numDoors = numDoors;
this.numWindows = numWindows;
}
public Car(int numDoors, int numWindows, Person driver, int[] numSeatsPerRow)
{
super(driver, numSeatsPerRow);
this.numDoors = numDoors;
this.numWindows = numWindows;
}
boolean canOpenDoor(Person p)
{
if(p.getAge() <= 5)
return false;
int[] pos = getLocationOfPersonInVehicle(p);
int row = pos[0];
int col = pos[1];
// chheck if person is in the outer seats
if(col == 0 ||col == numSeatsPerRow[row]-1 || col == numSeatsPerRow[row])
{
// now, check num of windows
if(numDoors < 2*numberOfRows)
{
if(row <= (numDoors/2 - 1))
return true;
else
return false;
}
else
{
return true;
}
}
else
return false;
}
boolean canOpenWindow(Person p)
{
if(p.getAge() <= 2)
return false;
int[] pos = getLocationOfPersonInVehicle(p);
int row = pos[0];
int col = pos[1];
// chheck if person is in the outer seats
if(col == 0 ||col == numSeatsPerRow[row]-1 || col == numSeatsPerRow[row])
{
// now, check num of windows
if(numWindows < 2*numberOfRows)
{
if(row <= (numWindows/2 - 1))
return true;
else
return false;
}
else
{
return true;
}
}
else
return false;
}
@Override
public boolean equals(Object other)
{
// check that object is an instance of car
if(!(other instanceof Car))
return false;
Car o = (Car)other;
// check that both cars have same seat configuration
if(getNumDoors() != o.getNumDoors())
return false;
if(getNumWindows() != o.getNumWindows())
return false;
if(getNumberOfMaxSeatsPerRow() != o.getNumberOfMaxSeatsPerRow())
return false;
if(getNumberOfRows() != o.getNumberOfRows())
return false;
// check same seats configuration
for(int i = 0; i < getNumberOfRows(); i++)
{
if(getNumberOfSeatsPerRow()[i] != o.getNumberOfSeatsPerRow()[i])
return false;
}
return true;
}
@Override
public String toString()
{
String seats_per_row = "[";
for(int i = 0; i < getNumberOfRows(); i++)
{
seats_per_row += getNumberOfSeatsPerRow()[i];
if(i < getNumberOfRows()-1)
seats_per_row += ",";
}
seats_per_row += "]";
String people_onboard = "[";
int n_persons = 0;
for(int i = 0; i < getNumberOfRows(); i++)
{
Person[]persons = getPeopleInRow(i);
if(persons != null)
{
for(int j = 0; j < getPeopleInRow(i).length; j++)
{
Person thisperson = getPeopleInRow(i)[j];
if(thisperson != null)
{
if(n_persons == 0)
people_onboard += thisperson.getName();
else
people_onboard += "," + thisperson.getName();
n_persons++;
}
}
}
}
people_onboard += "]";
return String.format("Car: number of doors = %02d | number of windows = %02d | number of rows = %02d | seats per row = %s | names of people on board = %s\n", getNumDoors(), getNumWindows(), getNumberOfRows(), seats_per_row, people_onboard);
}
@Override
public int compareTo(Car o) {
// TODO Auto-generated method stub
int n1 = 0; // hold number of seats of calling object
int n2 = 0; // hold number of seats of passed object
for(int i = 0; i < getNumberOfRows(); i++)
n1 += getNumberOfSeatsPerRow()[i];
for(int i = 0; i < o.getNumberOfRows(); i++)
n2 += o.getNumberOfSeatsPerRow()[i];
if(n1 < n2)
return -1;
else if(n1 > n2)
return 1;
return 0;
}
@Override
public boolean loadPassenger(Person p) {
// TODO Auto-generated method stub
// assign passenger to the first available seat
if(getNumberOfAvailableSeats() < 1)
return false;
for(int i = 0; i < getNumberOfRows(); i++)
{
for(int j = 0; j < getNumberOfSeatsPerRow()[i]; j++)
{
if(getPersonInSeat(i, j) == null) // no person in this seat
{
if(i == 0) // first row
{
if(p.getAge() >= 5 && p.getHeight() >= 36)
{
setPassenger(p, i, j);
return true;
}
}
else
{
setPassenger(p, i, j);
return true;
}
}
}
}
return false;
}
@Override
public int loadPassengers(Person[] peeps) {
// TODO Auto-generated method stub
int loaded = 0;
for(Person p: peeps)
{
if(loadPassenger(p))
loaded++;
}
return loaded;
}
}
Related Samples
Explore our Java programming homework samples to see firsthand how our expert team tackles complex assignments. Our samples showcase comprehensive solutions that demonstrate Java proficiency and problem-solving skills. Whether you're struggling with Java basics or advanced concepts, these samples offer valuable insights into our approach to delivering top-notch programming homework help.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java