Instructions
Objective
Write a C++ assignment program to implement ham radio countries.
Requirements and Specifications
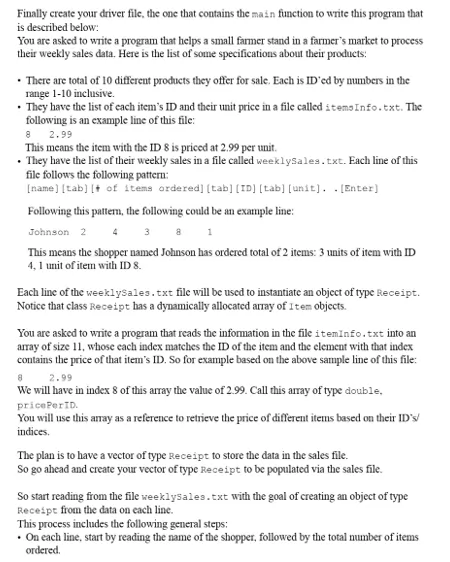
Source Code
#include "Receipt.h"
#include
#include
// Create a receipt
Receipt::Receipt(string shopperName)
: shopperName(shopperName)
{
shoppingList = new Item[maxItems];
numItems = 0;
}
// Initialize the shopper name
void Receipt::setShopperName(string shopperName)
{
this->shopperName = shopperName;
}
// Return the shopper name
string Receipt::getShopperName()
{
return shopperName;
}
// Initialize the shopping list
void Receipt::setShoppingList(Item *shoppingList)
{
this->shoppingList = shoppingList;
}
// Return the shopping list
Item *Receipt::getShoppingList() const
{
return shoppingList;
}
// Return the number of itmes
int Receipt::getNumItems()
{
return numItems;
}
// Add a new item
void Receipt::addItem(Item item)
{
if (numItems >= maxItems)
return;
shoppingList[numItems++] = item;
}
// Display the content of the shopping list
void Receipt::displayTheShoppingList()
{
cout << "Shopper Name: " << shopperName << endl;
for (int i = 0; i < numItems; i++)
shoppingList[i].displayItemInfo();
cout << " Total Charge: $" << computeTotalCharge() << endl;
}
// Calculate the total charge
double Receipt::computeTotalCharge()
{
double total = 0;
for (int i = 0; i < numItems; i++)
total += shoppingList[i].computePicePerItem();
return total;
}
Related Samples
Explore our C++ sample solutions, showcasing our expertise in delivering high-quality, error-free code. Whether it's basic syntax or advanced algorithms, our samples highlight the precision and clarity we bring to every project. Trust us to help you excel in your programming assignments.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++