Instructions
Objective
Write a c++ assignment program to implement puzzle.
Requirements and Specifications
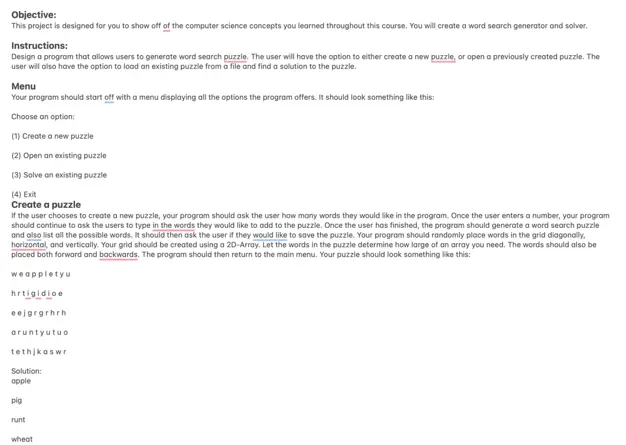
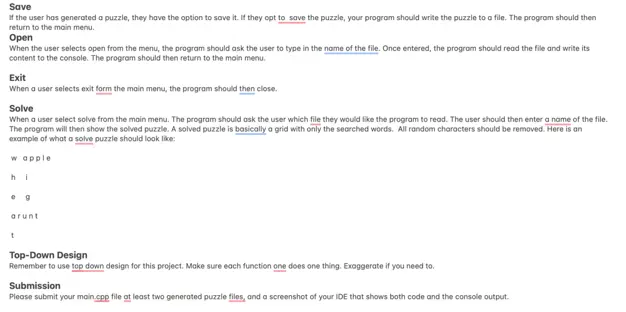
Source Code
#include
#include
#include
using namespace std;
string puzzleMaker(string str, int L)
{
int Len = L * 2;
char word[Len];
int WordLen = str.length();
int count = 0;
for (int i = 0; i < Len; i++)
{
if (count < WordLen and i % 2 != 0)
{
word[i] = str[count];
count++;
}
else
{
word[i] = 'a' + rand() % 26;
}
}
return word;
}
void createNew()
{
int noOfWords = 0, highLen = 0;
cout << "How many words you like to type?:";
cin >> noOfWords;
string words[noOfWords];
string puzzles[noOfWords];
for (int i = 0; i < noOfWords; i++)
{
cout << "Enter word [" << i + 1 << "]:";
cin >> words[i];
int tempSize = words[i].length();
if (tempSize >= highLen)
{
highLen = tempSize;
}
}
for (int i = 0; i < noOfWords; i++)
{
string tempWord = puzzleMaker(words[i], highLen);
puzzles[i] = tempWord;
}
cout << "Do you want to save this?(Y/N):";
char ans;
cin >> ans;
if (ans == 'y' || ans == 'Y')
{
string fileName;
cout << "Enter file name to save puzzle:";
cin >> fileName;
fstream puzzleData;
puzzleData.open(fileName + ".txt", ios::out);
if (puzzleData.is_open())
{
puzzleData << "Puzzles";
for (int i = 0; i < noOfWords; i++)
{
puzzleData << puzzles[i] << "";
}
puzzleData << "Solutions";
for (int i = 0; i < noOfWords; i++)
{
puzzleData << words[i] << "";
}
}
}
}
void openOld(string fileName)
{
fstream puzzleData;
puzzleData.open(fileName + ".txt", ios::in);
if (puzzleData.is_open())
{
string tempData;
while (getline(puzzleData, tempData))
{
cout << tempData << "";
}
}
}
class Node
{
public:
string data;
Node *next;
Node(string value)
{
data = value;
next = NULL;
}
};
void insert(Node *&head, string value)
{
Node *n = new Node(value);
if (head == NULL)
{
head = n;
return;
}
Node *temp = head;
while (temp->next != NULL)
{
temp = temp->next;
}
temp->next = n;
}
void solvePuzzle(string fileName)
{
Node *puzzles = NULL;
Node *solutions = NULL;
fstream puzzleData;
puzzleData.open(fileName + ".txt", ios::in);
if (puzzleData.is_open())
{
string tempData;
int val = 0;
while (getline(puzzleData, tempData))
{
if (val == 2)
{
insert(puzzles, tempData);
}
if (val == 1)
{
insert(solutions, tempData);
}
if (tempData == "Puzzles")
{
val = 2;
}
if (tempData == "Solutions")
{
val = 1;
}
}
Node *temp1 = solutions;
Node *temp2 = puzzles;
while (temp1 != NULL)
{
while (temp2 != NULL)
{
if (temp1 == NULL)
{
temp2 = NULL;
continue;
}
string selectedSolution = temp1->data;
string selectedPuzzle = temp2->data;
int lenPuzzle = strlen(selectedPuzzle.c_str());
int lenSolution = strlen(selectedSolution.c_str());
for (int i = 0; i < lenSolution; i++)
{
for (int j = 0; j < lenPuzzle; j++)
{
if (selectedSolution[i] == selectedPuzzle[j])
{
cout << selectedSolution[i];
i = i + 1;
}
else
{
cout << " ";
}
}
}
cout << "";
temp2 = temp2->next;
temp1 = temp1->next;
}
}
}
}
int main()
{
bool start = true;
while (start)
{
cout << "Choose an option:" << endl;
cout << "(1) Create a new puzzle" << endl;
cout << "(2) Open an existing puzzle" << endl;
cout << "(3) Solve an existing puzzle" << endl;
cout << "(4) Exit" << endl;
int userInput = 0;
cin >> userInput;
string fileName1, fileName2;
switch (userInput)
{
case 1:
createNew();
break;
case 2:
cout << "Enter file name to read old puzzle:";
cin >> fileName1;
openOld(fileName1);
break;
case 3:
cout << "Enter file name to read old puzzle:";
cin >> fileName2;
solvePuzzle(fileName2);
break;
case 4:
start = false;
break;
default:
cout << "Please enter a valid choice 1-4!" << endl;
break;
}
}
}
Similar Samples
Explore our curated collection of programming homework samples at ProgrammingHomeworkHelp.com. From Java and Python to Machine Learning and Data Structures, our samples exemplify our expertise in solving diverse programming challenges. Each example is designed to illustrate best practices and provide insights into tackling assignments effectively. Dive into our samples to see how we can assist you in mastering programming concepts and achieving academic excellence
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++