Instructions
Requirements and Specifications
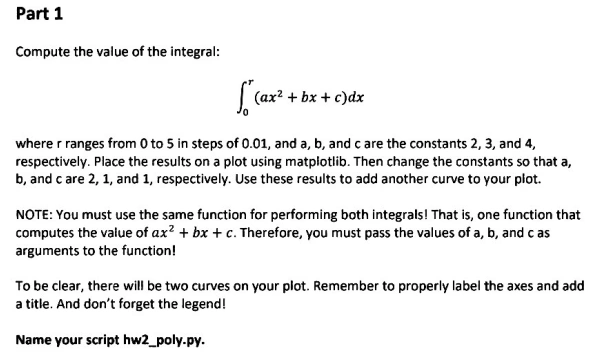
Source Code
POLYNOMIAL
from scipy import integrate
import numpy as np
import matplotlib.pyplot as plt
# Create a function that computes the value of ax^2 + bx + c
def f(x, a, b, c):
return a*x**2 + b*x + c
if __name__ == '__main__':
# First, define the range of r
r = np.arange(0, 5, 0.01)
# Define constants a, b, and c
a = 2
b = 3
c = 4
# Now, compute the first curve
curve1 = list()
for ri in r:
curve1.append(integrate.quad(f, 0, ri, args=(a,b,c))[0])
# Now, change the values for the second curve
a, b, c = 2, 1, 1
# Compute econd curve
curve2 = list()
for ri in r:
curve2.append(integrate.quad(f, 0, ri, args=(a,b,c))[0])
# Finally, plot both curves
plt.figure()
plt.plot(r, curve1, label = 'Curve 1')
plt.plot(r, curve2, label = 'Curve 2')
plt.grid(True)
plt.legend()
plt.xlabel('r')
plt.ylabel('ax^2+bx+c')
plt.show()
Related Samples
Explore our Python Assignments sample section, where you'll find meticulously crafted solutions to diverse programming tasks. From foundational exercises to intricate algorithms, each sample offers clear, annotated code for learning and reference. Perfect for students and professionals looking to sharpen their Python skills and excel in programming assignments. Dive into Python proficiency with our curated samples today!
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python