Instructions
Objective
Write a program to implement playlist handling system in C++.
Requirements and Specifications
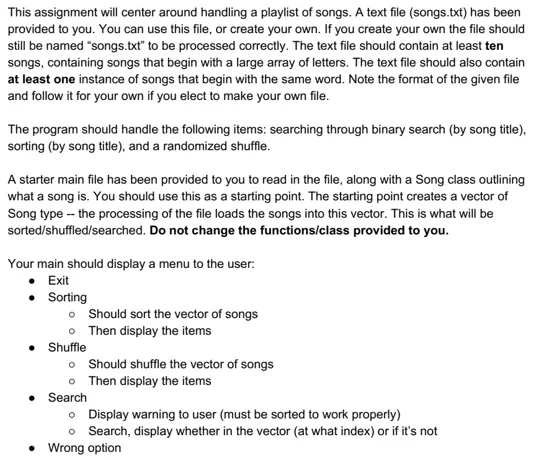
Source Code
SONG
#include "song.h"
Song::Song()
{
title = "";
artist = "";
}
Song::Song(string title, string artist)
{
this->title = title;
this->artist = artist;
}
// getters
string Song::getTitle()
{
return title;
}
string Song::getArtist()
{
return artist;
}
// setters
void Song::setTitle(string title)
{
this->title = title;
}
void Song::setArtist(string artist)
{
this->artist = artist;
}
MAIN
/*
Name: NetID:
Date: Due Date:
Description:
*/
/*
The shuffle algorithm is called 'fisher-yates'.
Reference: https://www.techiedelight.com/shuffle-vector-cpp/
The sort algorithm is called 'bubble sort'.
Reference: https://www.geeksforgeeks.org/bubble-sort/
The search algorithm is called 'binary search'.
Reference: https://www.geeksforgeeks.org/binary-search/
*/
#include
#include
#include
#include
#include
#include
#include "song.h"
using namespace std;
// given to you
void processFile(vector &playlist);
// you should create
void shuffle(vector &playlist);
void bubbleSort(vector &playlist);
void displayPlaylist(vector &playlist);
int binarySearch(vector &playlist, string &title);
// add your own sort function
int main()
{
vector playlist;
// sets up playlist
processFile(playlist);
cout << "\nInitial playlist: " << endl;
displayPlaylist(playlist);
while (true)
{
cout << endl;
// Display a menu
cout << "Welcome to the playlist display manager." << endl << endl;
cout << "0. Exit" << endl;
cout << "1. Sort Playlist" << endl;
cout << "2. Shuffle Playlist" << endl;
cout << "3. Search Playlist" << endl;
cout << "Which option would you like? ";
string option;
getline(cin, option);
cout << endl;
// Execute user's option
if (option == "0")
break;
if (option == "1")
{
// Do sorting
bubbleSort(playlist);
displayPlaylist(playlist);
}
else if (option == "2")
{
// Do shuffling
shuffle(playlist);
displayPlaylist(playlist);
}
else if (option == "3")
{
// So searching, binary search requires the list
// to be sorted so we make sure it is sorted first
;bubbleSort(playlist);
cout << "Enter a song title: ";
string title;
getline(cin, title);
int i = binarySearch(playlist, title);
if (i == -1)
cout << "Error: The song title does not exist." << endl;
else
cout << playlist[i].getTitle() << " - " << playlist[i].getArtist() << endl;
}
else
{
cout << "Error: Invalid option." << endl;
}
}
cout << "Good-bye!" << endl;
return 0;
}
// Perform a binary search
int binarySearch(vector &playlist, string &title)
{
int left = 0;
int right = (int) playlist.size() - 1;
while (left <= right)
{
int middle = left + (right - left) / 2;
if (playlist[middle].getTitle() == title)
return middle;
if (playlist[middle].getTitle() < title)
left = middle + 1;
else
right = middle - 1;
}
return -1;
}
// Sort the play list by title
void bubbleSort(vector &playlist)
{
int n = (int)playlist.size();
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n - i - 1; j++)
{
if (playlist[j].getTitle() > playlist[j + 1].getTitle())
{
Song temp = playlist[j];
playlist[j] = playlist[j + 1];
playlist[j + 1] = temp;
}
}
}
}
// Shuffle the play list
void shuffle(vector &playlist)
{
int n = (int) playlist.size();
for (int i = 0; i < n; i++)
{
int j = i + rand() % (n - i);
Song temp = playlist[i];
playlist[i] = playlist[j];
playlist[j] = temp;
}
}
// Print out the play list
void displayPlaylist(vector &playlist)
{
for (unsigned i = 0; i < playlist.size(); i++)
cout << playlist[i].getTitle() << " - " << playlist[i].getArtist() << endl;
}
// Load the contens of songs file
void processFile(vector &playlist)
{
ifstream infile;
string line;
infile.open("songs.txt");
if (infile.is_open())
{
cout << "Successful songs opening." << endl;
}
else
{
cout << "Couldn't locate file. Program closing." << endl;
exit(EXIT_FAILURE);
}
while (getline(infile, line))
{
// first line --> song
// second line --> artist
if (line != "")
{
string song, artist;
song = line;
getline(infile, artist);
Song temp(song, artist);
playlist.push_back(temp);
}
}
return;
}
Related Samples
Struggling with your C++ assignments? Check out our detailed sample to see how our experts handle complex programming tasks with ease. From basic syntax to advanced algorithms, we provide comprehensive solutions tailored to your needs. Let us help you excel in your programming coursework!
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++