Tip of the day
News
Instructions
Objective
Write a C++ homework to implement a phone book system.
Requirements and Specifications
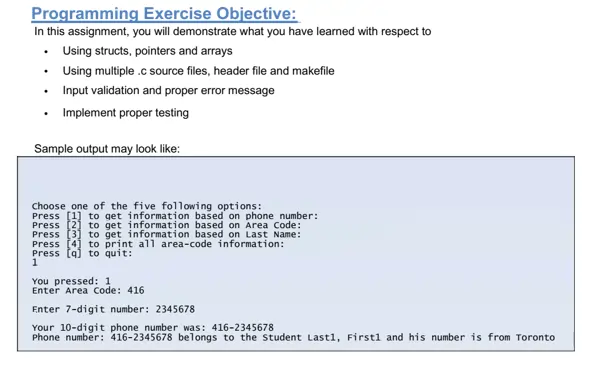
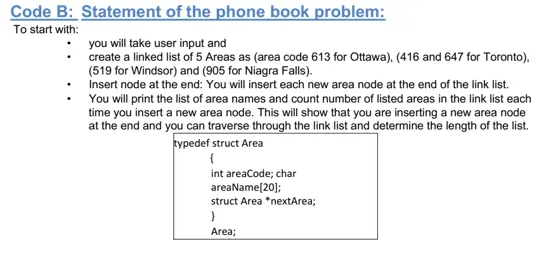
Source Code
#include "phentries.h"
#include
#include
#include
/* Create a new entry node */
PhEntry *phentries_create(int area_code, int phone_number, const char *last_name,
const char *first_name)
{
PhEntry *entry;
entry = (PhEntry *)malloc(sizeof(PhEntry));
entry->areaCode = area_code;
entry->phoneNumber = phone_number;
strcpy(entry->lastName, last_name);
strcpy(entry->firstName, first_name);
entry->nextPhEntry = NULL;
entry->prevPhEntry = NULL;
return entry;
}
/* Display an individual entry information */
void phentries_print_entry(PhEntry *entry)
{
printf("Area Code: %d\n", entry->areaCode);
printf("Phone Number: %d\n", entry->phoneNumber);
printf("Last Name: %s\n", entry->lastName);
printf("First Name: %s\n", entry->firstName);
}
/* Insert a new entry on top of the list */
void phentries_insert_entry(PhEntry **entries, PhEntry *entry)
{
entry->nextPhEntry = *entries;
if (entry->nextPhEntry != NULL)
entry->nextPhEntry->prevPhEntry = entry;
*entries = entry;
}
/* Find the entry that holds the given last name */
PhEntry *phentries_find_by_lastname(PhEntry *entries, char last_name[])
{
PhEntry *current;
current = entries;
while (current != NULL)
{
if (strcmp(current->lastName, last_name) == 0)
return current;
current = current->nextPhEntry;
}
return NULL;
}
/* Find the entry that holds the given phone number*/
PhEntry *phentries_find_by_phone(PhEntry *entries, int area_code, int phone)
{
PhEntry *current;
current = entries;
while (current != NULL)
{
if (current->areaCode == area_code && current->phoneNumber == phone)
return current;
current = current->nextPhEntry;
}
return NULL;
}
/* Find the first entry that has the given area code */
PhEntry *phentries_find_by_area(PhEntry *entries, int area_code)
{
PhEntry *current;
current = entries;
while (current != NULL)
{
if (current->areaCode == area_code)
return current;
current = current->nextPhEntry;
}
return NULL;
}
/* Deallocate all entry nodes */
void phentries_delete_all(PhEntry **entries)
{
PhEntry *next;
PhEntry *current;
current = *entries;
while (current != NULL)
{
next = current->nextPhEntry;
free(current);
current = next;
}
*entries = NULL;
}
/* Display all entries */
void ph_entries_print_all(PhEntry *entries)
{
PhEntry*current;
current = entries;
while (current != NULL)
{
printf("Area Code: %d, Phone: %d, Last Name: %s, First Name: %s\n",
current->areaCode,
current->phoneNumber,
current->lastName,
current->firstName);
current = current->nextPhEntry;
}
}
Similar Sample
Explore our C++ assignment sample to understand how we tackle complex programming tasks. At ProgrammingHomeworkHelp.com, we provide clear, well-commented solutions that illustrate best practices in C++ programming. Whether it's basic syntax or advanced algorithms, our samples demonstrate our expertise in delivering high-quality assignments.
C++
Word Count
6780 Words
Writer Name:Alexander Gough
Total Orders:2435
Satisfaction rate:
C++
Word Count
6185 Words
Writer Name:Neven Bell
Total Orders:2376
Satisfaction rate:
C++
Word Count
5749 Words
Writer Name:Professor Kevin Tan
Total Orders:569
Satisfaction rate:
C++
Word Count
4786 Words
Writer Name:Dr. Isabelle Taylor
Total Orders:580
Satisfaction rate:
C++
Word Count
3997 Words
Writer Name:Professor Oliver Bennett
Total Orders:304
Satisfaction rate:
C++
Word Count
6830 Words
Writer Name:Dr. Maddison Todd
Total Orders:865
Satisfaction rate:
C++
Word Count
4424 Words
Writer Name:Dr. Alexandra Mitchell
Total Orders:589
Satisfaction rate:
C++
Word Count
7250 Words
Writer Name:Mr. Harrison Yu
Total Orders:311
Satisfaction rate:
C++
Word Count
4894 Words
Writer Name:James Miller
Total Orders:824
Satisfaction rate:
C++
Word Count
5544 Words
Writer Name:Callum Chambers
Total Orders:932
Satisfaction rate:
C++
Word Count
3533 Words
Writer Name:Aaliyah Armstrong
Total Orders:658
Satisfaction rate:
C++
Word Count
3645 Words
Writer Name:Len E. Villasenor
Total Orders:489
Satisfaction rate:
C++
Word Count
4033 Words
Writer Name:Daniel M. Harrison
Total Orders:700
Satisfaction rate:
C++
Word Count
3576 Words
Writer Name:Dr. Isabella Wong
Total Orders:652
Satisfaction rate:
C++
Word Count
3600 Words
Writer Name:Prof. Liam Wilson
Total Orders:554
Satisfaction rate:
C++
Word Count
1035 Words
Writer Name:Professor Marcus Tan
Total Orders:544
Satisfaction rate:
C++
Word Count
6288 Words
Writer Name:Dr. Stephanie Kim
Total Orders:812
Satisfaction rate:
C++
Word Count
1586 Words
Writer Name:Ethan Patel
Total Orders:556
Satisfaction rate:
C++
Word Count
3852 Words
Writer Name:Sarah Rodriguez
Total Orders:689
Satisfaction rate:
C++
Word Count
4701 Words
Writer Name:Harry F. Grimmett
Total Orders:342
Satisfaction rate: