Instructions
Objective
Write a program to implement package explorer in java language.
Requirements and Specifications
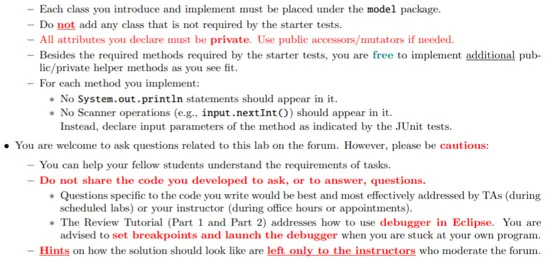
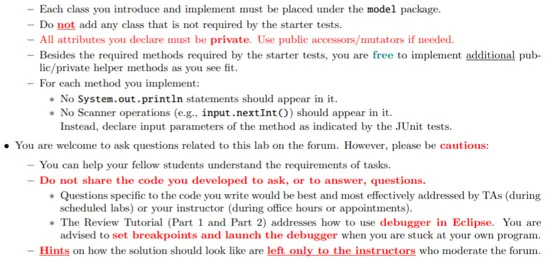
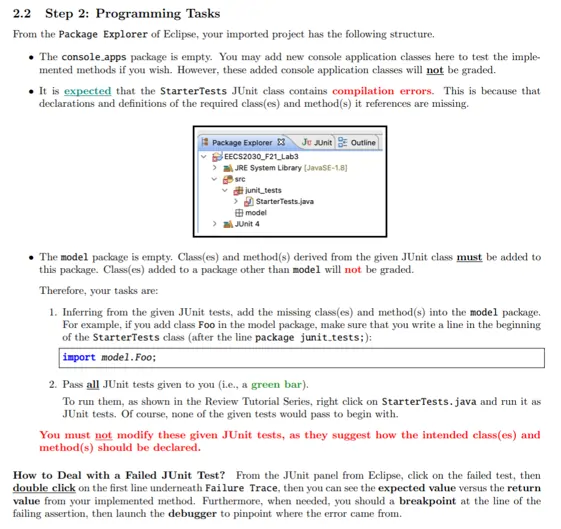
Source Code
package model;
// A bedroom, office, etc.
public class Unit {
private String function;
private double width;
private double length;
private String unit;
// Create a unit in square feet
public Unit(String function, int width, int length) {
this.function = function;
this.width = width;
this.length = length;
unit = "feet";
}
// Convert from feet to meters or vice-versa
public void toogleMeasurement() {
if (unit.equals("feet")) {
// Convert feet to meters
width *= 0.3048;
length *= 0.3048;
unit = "meters";
} else {
// Convert meters to feet
width *= 3.28084;
length *= 3.28084;
unit = "feet";
}
}
// Return the current unit being used
public String getUnit() {
return unit;
}
// Return the total area of this unit
public double getArea() {
return width * length;
}
// Return the function
public String getFunction() {
return function;
}
// Return the width
public double getWidth() {
return width;
}
// Return the length
public double getLength() {
return length;
}
// Return a string representation
@Override
public String toString() {
if (unit.equals("feet"))
return "A unit of " + (int) getArea() + " square " + unit + " (" + (int) width + "' wide and "
+ (int) length + "' long) functioning as " + function;
return "A unit of " + String.format("%.2f", getArea()) + " square " + unit + " ("
+ String.format("%.2f", width) + " m wide and " + String.format("%.2f", length)
+ " m long) functioning as " + function;
}
// Check if two units are of equal area and of the same function
@Override
public boolean equals(Object other) {
if (this == other)
return true;
if (!(other instanceof Unit))
return false;
Unit otherUnit = (Unit) other;
return function.equals(otherUnit.function) && getArea() == otherUnit.getArea();
}
}
one(self, item):
"""
Delete the first occurrence of the item
"""
if item in self.__items:
self.__items.remove(item)
def erase_all(self, item):
"""
Delete all occurrence of the item in the list
"""
while item in self.__items:
self.__items.remove(item)
def count(self, item):
"""
Count the occurrence of item in the list
"""
occurrence = 0
for some_item in self.__items:
if some_item == item:
occurrence += 1
return occurrence
def items(self):
"""
Return distinct items in the list
"""
distinct_items = list()
for item in self.__items:
if item not in distinct_items:
distinct_items.append(item)
return distinct_items
def __str__(self):
"""
Return a string representation of the container
"""
return str(self.__items)[1:-1]
def __repr__(self):
"""
Returns a printable representation of the container
"""
return str(self.__items)[1:-1]
def __getitem__(self, idx):
"""
Access an item from the list given the index
"""
return self.__items[idx]
def __add__(self, other_container):
"""
Return a new container that contains all the items
that is here and the other container
"""
new_container = Container()
new_container.__items = self.__items + other_container.__items
return new_container
def __sub__(self, other_container):
"""
Return a new container where items here does not exist
on the other container
"""
paired_indices = list()
for i in range(len(other_container.__items)):
paired_indices.append(False)
new_container = Container()
for i in range(len(self.__items)):
pair_found = False
for j in range(len(other_container.__items)):
if self.__items[i] == other_container.__items[j] and not paired_indices[j]:
pair_found = True
paired_indices[j] = True
break
if not pair_found:
new_container.__items.append(self.__items[i])
return new_container
def __mul__(self, other_container):
"""
Find the intersection of two containers
"""
paired_indices = list()
for i in range(len(other_container.__items)):
paired_indices.append(False)
new_container = Container()
for i in range(len(self.__items)):
pair_found = False
for j in range(len(other_container.__items)):
if self.__items[i] == other_container[j] and not paired_indices[j]:
pair_found = True
paired_indices[j] = True
break
if pair_found:
new_container.__items.append(self.__items[i])
return new_container
Related Samples
Explore our free Java assignment samples for clarity on complex topics. These samples offer detailed solutions and practical examples, guiding you through various Java programming challenges. Use them as a valuable resource to improve your approach and solve your assignments more efficiently.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java