Instructions
Objective
Write a java assignment program to implement object oriented programming.
Requirements and Specifications
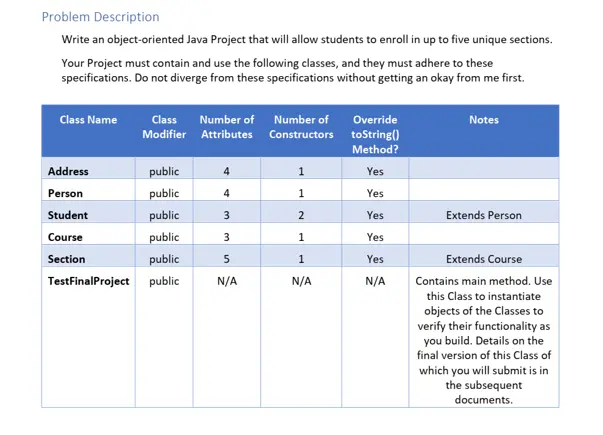
Source Code
import java.io.File;
import java.util.Scanner;
public class DataStore {
private static DataStore instance;
private final String SECTIONS_FILENAME = "sections1.txt";
private Section[] sections;
private int numSections;
// Load the sections from file
private DataStore() {
sections = new Section[10];
numSections = 0;
try {
Scanner inFile = new Scanner(new File(SECTIONS_FILENAME));
while (inFile.hasNext()) {
String subject = inFile.next();
String number = inFile.next();
String name = inFile.next();
String id = inFile.next();
String days = inFile.next();
String startTime = inFile.next();
String building = inFile.next();
String room = inFile.next();
Section section = new Section(subject, number, name, id,
days, startTime, building, room);
addSection(section);
}
inFile.close();
} catch (Exception e) {
e.printStackTrace(System.out);
System.exit(0);
}
}
// Return a list of sections
public Section[] getSections() {
Section[] result = new Section[numSections];
for (int i = 0; i < numSections; i++) {
result[i] = sections[i];
}
return result;
}
// Add a new section to the data store
public void addSection(Section section) {
if (numSections >= sections.length) {
sections = expandSections(sections);
}
sections[numSections++] = section;
}
// Expand the objects twice its size, made it an 'object' instead
// of a specific data type to make it more generic and applicable
// to other arrays as well if in case we expand this program
private Section[] expandSections(Section[] objects) {
Section[] newSections = new Section[objects.length * 2];
for (int i = 0; i < objects.length; i++) {
newSections[i] = objects[i];
}
return newSections;
}
// Return the only instance of a data store
public static DataStore getInstance() {
if (instance == null) {
instance = new DataStore();
}
return instance;
}
}
Similar Samples
At ProgrammingHomeworkHelp.com, explore our curated samples of programming assignments across various languages and difficulty levels. These examples demonstrate our expertise in delivering precise, well-commented solutions. Whether you're grappling with Java, Python, or C++, our samples showcase how we ensure clarity and quality in every task. Discover how we can elevate your programming skills today.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java