Instructions
Requirements and Specifications
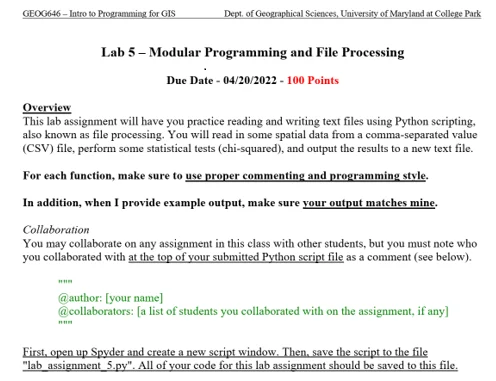
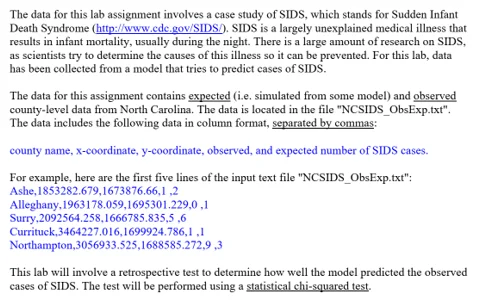
Source Code
import math
from os.path import exists
def calculateZscore(fin, fout):
# List to store z values
zlist = list()
# Read all lines
lines = fin.readlines()
# Close file
fin.close()
# Loop through lines
for line in lines:
# Strip
line = line.strip()
# Split
row = line.split(',')
county = row[0]
x = float(row[1])
y = float(row[2])
Oi = int(row[3])
Ei = int(row[4])
# Calculate zi
zi = (Oi-Ei)/math.sqrt(Ei)
# Append to list
zlist.append(zi)
# Write to output file
fout.write(line + "," + str(zi) + "\n")
return zlist
def globalchiSquare(zlist):
# Variable to store globalchi square
X2 = 0.0
# Loop over the z scores
for zi in zlist:
# Add the square value of this zi score
X2 += zi**2
#Get number of records
n = len(zlist)
# Return
return (X2, n)
def chiCritical(chiFile, n, siglevel):
# First, read the chiFile. Get all lines
lines = chiFile.readlines()
# Since the first line contains the header, we will ignore it
lines.pop(0)
# Now, read all values, split and store into a list of sublist
chitable = list()
for line in lines:
# Strip
line = line.strip()
# Split
row = line.split(' ')
# Convert all elements in row to float
sublist = list()
for x in row:
if len(x) > 0:
sublist.append(float(x))
chitable.append(sublist)
# Now, get degree of freedon
df = n - 1
# Search for the row where df is equal to this value
Pcritical = None
for row in chitable:
if row[0] == df:
# Get P value
if siglevel == 5.0:
Pcritical = row[1]
elif siglevel == 1.0:
Pcritical = row[2]
else:
Pcritical = row[3]
break
return Pcritical
def main():
# Ask for filename
while True:
filenamein = input("What is the name of the input file?: ")
# Check that files exist
if not exists(filenamein):
print('***Error: Input File Not Found, try Again***')
else:
break
while True:
filename_chi = input("What is the name of the chi-squared table? ")
if not exists(filename_chi):
print('***Error: Input File Not Found, try Again***')
else:
break
filenameout = input("What is the name of the output file? ")
# Ask for significance level
while True:
try:
siglevel = float(input("What is the significance level? 5, 1, or 0.1? (%) "))
if siglevel == 5.0 or siglevel == 1.0 or siglevel == 0.1:
break
else:
print("***Error: Invalid Significance Leel, Try Again***")
except:
print("***Error: Invalid Significance Leel, Try Again***")
# Open file with data
inFile = open(filenamein, 'r')
# Open output file
outFile = open(filenameout, 'w+')
# Open chitable file
chiFile = open(filename_chi, 'r')
print("Performing chi-squared test on SIDS cases in North Carolina")
zList = calculateZscore(inFile, outFile)
(X2, n) = globalchiSquare(zList)
Pcritical = chiCritical(chiFile, n, siglevel)
print(f'There are {n} Counties in the sample dataset')
print('The global chi-squared statistic is {:.2f}'.format(X2))
print('Critical value at {:.1f}% significance level: {:.2f}'.format(siglevel, Pcritical))
if X2 > Pcritical:
print('The null hypothesis of no spatial pattern *is rejected* at {:.1f}% significance level'.format(siglevel))
else:
print('The null hypothesis of no spatial pattern *cannot be rejected* at {:.1f}% significance level'.format(siglevel))
# Close
inFile.close()
outFile.close()
chiFile.close()
if __name__ == '__main__':
main()
Related Samples
Discover our Python Assignments samples, covering essential topics including data structures, functions, modules, and algorithms. These comprehensive solutions are designed to assist students in mastering Python programming concepts, providing clarity and practical insights to excel in assignments and coursework.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python