Instructions
Objective
Write a c++ assignment program to implement menu driven program.
Requirements and Specifications
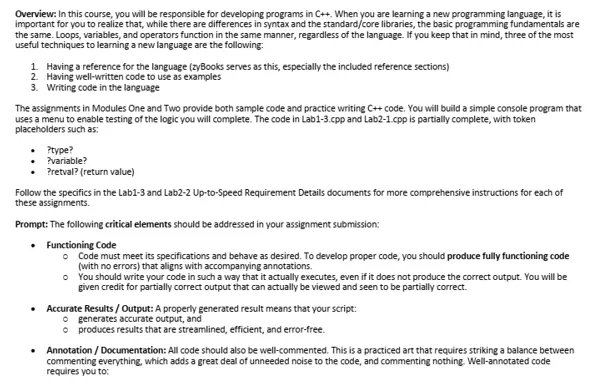
Source Code
//============================================================================
// Name : Lab2-2.cpp
// Author : Your Name
// Version : 1.0
// Copyright : Copyright © 2017 SNHU COCE
// Description : Lab 2-2 Up to Speed in C++, Part 2
//============================================================================
#include
#include
#include
// FIXME (1): Reference the CSVParser library
using namespace std;
//============================================================================
// Global definitions visible to all methods and classes
//============================================================================
// forward declarations
double strToDouble(string str, char ch);
struct Bid {
string title;
string fund;
double amount;
Bid() {
amount = 0.0;
}
};
//============================================================================
// Static methods used for testing
//============================================================================
/**
* Display the bid information
*
* @param bid struct containing the bid info
*/
void displayBid(Bid bid) {
cout << bid.title << " | " << bid.amount << " | " << bid.fund << endl;
return;
}
/**
* Prompt user for bid information
*
* @return Bid struct containing the bid info
*/
Bid getBid() {
Bid bid;
cout << "Enter title: ";
cin.ignore();
getline(cin, bid.title);
cout << "Enter fund: ";
cin >> bid.fund;
cout << "Enter amount: ";
cin.ignore();
string strAmount;
getline(cin, strAmount);
bid.amount = strToDouble(strAmount, '$');
return bid;
}
/**
* Load a CSV file containing bids into a container
*
* @param csvPath the path to the CSV file to load
* @return a container holding all the bids read
*/
vector loadBids(string csvPath) {
// Define a vector data structure to hold a collection of bids.
vector bids;
// initialize the CSV Parser using the given path
csv::Parser file = csv::Parser(csvPath);
// loop to read rows of a CSV file
for (unsigned int i = 0; i < file.rowCount(); i++) {
// data structure to hold data from each row and add to vector
Bid bid;
bid.title = file[i][0];
bid.fund = file [i][8];
bid.amount = strToDouble(file[i][4], '$');
bids.push_back(bid);
}
return bids;
}
/**
* Simple C function to convert a string to a double
* after stripping out unwanted char
*
* credit: http://stackoverflow.com/a/24875936
*
* @param ch The character to strip out
*/
double strToDouble(string str, char ch) {
str.erase(remove(str.begin(), str.end(), ch), str.end());
return atof(str.c_str());
}
/**
* The one and only main() method
*/
int main(int argc, char* argv[]) {
// process command line arguments
string csvPath;
switch (argc) {
case 2:
csvPath = argv[1];
break;
default:
csvPath = "eBid_Monthly_Sales_Dec_2016.csv";
}
// Define a vector to hold all the bids
vector allBids;
// Define a timer variable
clock_t start;
clock_t end;
int choice = 0;
while (choice != 9) {
cout << "Menu:" << endl;
cout << " 1. Enter a Bid" << endl;
cout << " 2. Load Bids" << endl;
cout << " 3. Display All Bids" << endl;
cout << " 9. Exit" << endl;
cout << "Enter choice: ";
cin >> choice;
switch (choice) {
case 1:
cout << "Not currently implemented." << endl;
break;
case 2:
// Initialize a timer variable before loading bids
start = clock();
// Complete the method call to load the bids
loadBids("eBid_Monthly_Sales_Dec_2016.csv");
// Calculate elapsed time and display result
end = clock();
cout << "time: " << end - start << " milliseconds" << endl;
cout << "time: " << (end-start)*1.0/CLOCKS_PER_SEC << " seconds" << endl;
break;
case 3:
// Loop and display the bids read
vector bids = loadBids("eBid_Monthly_Sales_Dec_2016.csv");
for ( unsigned int i = 0; i < bids.size(); ++i) {
displayBid(bids[i]);
}
cout << endl;
break;
}
}
cout << "Good bye." << endl;
return 0;
}
Similar Samples
Visit ProgrammingHomeworkHelp.com to explore our sample programming assignments. Our curated examples demonstrate proficiency in solving diverse programming problems across multiple languages. These samples showcase our commitment to delivering high-quality solutions tailored to your academic needs. See firsthand how we can assist you in mastering programming concepts effectively.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++