Tip of the day
News
Instructions
Objective
Write a python assignment program to implement list and tuples.
Requirements and Specifications
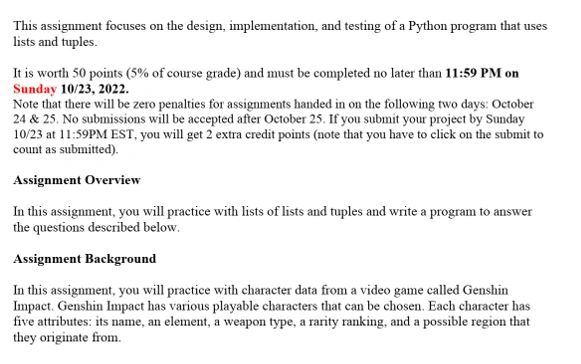
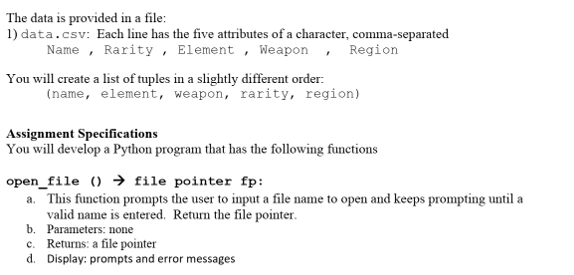
Source Code
# -*- coding: utf-8 -*-
"""
Created on Tue Oct 25 19:15:40 2022
@author: Owner
"""
import csv
from operator import itemgetter
NAME = 0
ELEMENT = 1
WEAPON = 2
RARITY = 3
REGION = 4
MENU = "\nWelcome to Genshin Impact Character Directory\n\
Choose one of below options:\n\
1. Get all available regions\n\
2. Filter characters by a certain criteria\n\
3. Filter characters by element, weapon, and rarity\n\
4. Quit the program\n\
Enter option: "
INVALID_INPUT = "\nInvalid input"
CRITERIA_INPUT = "\nChoose the following criteria\n\
1. Element\n\
2. Weapon\n\
3. Rarity\n\
4. Region\n\
Enter criteria number: "
VALUE_INPUT = "\nEnter value: "
ELEMENT_INPUT = "\nEnter element: "
WEAPON_INPUT = "\nEnter weapon: "
RARITY_INPUT = "\nEnter rarity: "
HEADER_FORMAT = "\n{:20s}{:10s}{:10s}{:<10s}{:25s}"
ROW_FORMAT = "{:20s}{:10s}{:10s}{:<10d}{:25s}"
def open_file():
'''
Prompts the user to input a file name to open
Returns: file pointer
'''
input_file = input("Enter file name: ")
while True:
try:
open_file = open(input_file)
return open_file
except FileNotFoundError:
print("\nError opening file. Please try again.")
input_file = input("Enter file name: ")
def read_file(fp):
'''
Reads the csv file
Value: file
Returns: list of tuples
'''
files = csv.reader(fp)
skip_header = next(files,None)
attributes_list = []
for line in files:
name = str(line[0])
rarity = int(line[1])
element = str(line[2])
weapon = str(line[3])
region = str(line[4]).strip()
region = region if region else None
attributes_tuple = (name , element , weapon , rarity , region)
attributes_list.append(attributes_tuple)
return attributes_list
def get_characters_by_criterion (list_of_tuples, criteria, value):
'''
Retrieve the characters that match a certain criteria
list_of_tuples: --
criteria: the index of a criteria in a character
value: type value
'''
criterion_list = [] #part1
for character in list_of_tuples:
if character[criteria] is None: #part2
continue #skips the following lines of code
elif criteria == RARITY: #part3
if character[criteria] == value and type(character[criteria]) is int and type(value) is int:
criterion_list.append(character)
else: #part4
if (value.lower() == character[criteria].lower()) and type(character[criteria]) is str and type(value) is str:
criterion_list.append(character)
return criterion_list
def get_characters_by_criteria(master_list, element, weapon, rarity):
'''
Returns a list of tuples filtered with parameters
master_list: list of tuples
element: argument for get_characters_by_criterion
weapon: argument for get_characters_by_criterion
rarity: argument for get_characters_by_criterion
'''
criteria_one = get_characters_by_criterion(master_list , ELEMENT , element)
criteria_two = get_characters_by_criterion(criteria_one , WEAPON , weapon)
criteria_three = get_characters_by_criterion(criteria_two , RARITY, rarity)
return criteria_three
def get_region_list (master_list):
'''
Retrieve available regions into a list
Value: tuples
Returns: sorted list
'''
reg_list = []
for line in master_list:
reg = line[4]
if reg != None:
if reg in reg_list:
continue
else:
reg_list.append(reg)
reg_list_sorted = sorted(reg_list)
return reg_list_sorted
def sort_characters (list_of_tuples):
'''
Creates a new sorted list
Value: tuples
Returns: sorted tuples
'''
return sorted(list_of_tuples, key=itemgetter(3) , reverse = True)
def display_characters (list_of_tuples):
'''
Displays the characters with information
Value: tuples
Returns: --
'''
if list_of_tuples == []:
print("\nNothing to print.")
else:
print(HEADER_FORMAT.format("Character", "Element", "Weapon", "Rarity", "Region"))
for line in list_of_tuples:
region = line[4]
if region == None:
print(ROW_FORMAT.format( line[0] , line[1] , line[2] , line[3] , 'N/A' ))
else:
print(ROW_FORMAT.format( line[0] , line[1] , line[2] , line[3] , region ))
def get_option():
'''
Prompt for input
Value: --
Returns: input selected
'''
while True:
menu_input = input(MENU)
try:
menu_input_int = int(menu_input)
if 1 <= menu_input_int <= 4:
return menu_input_int
else:
print("\nNothing to print.")
except ValueError:
print("\nNothing to print.")
def main():
data = read_file(open_file())
menu_input = get_option()
while menu_input != 4:
if menu_input == 1:
print("\nRegions:")
regions = get_region_list(data)
print(", ".join(regions) )
if menu_input == 2:
input_criteria = input(CRITERIA_INPUT)
input_value = ""
try:
input_criteria_int = int(input_criteria)
if not (1 <= input_criteria_int <= 4):
print(INVALID_INPUT)
input_criteria = input(CRITERIA_INPUT)
except ValueError:
print(INVALID_INPUT)
input_criteria = input(CRITERIA_INPUT)
input_value = input(VALUE_INPUT)
if input_criteria_int == RARITY:
try:
input_value = int(input_value)
except:
print(INVALID_INPUT)
menu_input = get_option()
continue
character_criterion = get_characters_by_criterion(data, input_criteria_int, input_value)
character_sort = sort_characters(character_criterion)
display_characters(character_sort)
if menu_input == 3:
element = (input(ELEMENT_INPUT)).upper()
weapon = (input(WEAPON_INPUT)).upper()
rarity = input(RARITY_INPUT)
while True:
try:
rarity = int(rarity)
break
except ValueError:
print(INVALID_INPUT)
rarity = input(RARITY_INPUT)
character_criteria = get_characters_by_criteria(data, element, weapon, rarity)
character_sort = sort_characters(character_criteria) #iii.
display_characters(character_sort)
menu_input = get_option()
# DO NOT CHANGE THESE TWO LINES
#These two lines allow this program to be imported into other code
# such as our function_test code allowing other functions to be run
# and tested without 'main' running. However, when this program is
# run alone, 'main' will execute.
if __name__ == "__main__":
main()
Related Samples
Check out our Python assignment samples: Explore a curated selection covering diverse topics from basic algorithms to advanced data analysis. Each sample provides clear problem-solving strategies to enhance your Python skills and deepen your understanding of programming concepts, perfect for student learning.
Python
Word Count
4091 Words
Writer Name:Walter Parkes
Total Orders:2387
Satisfaction rate:
Python
Word Count
2184 Words
Writer Name:Dr. Jesse Turner
Total Orders:2365
Satisfaction rate:
Python
Word Count
6429 Words
Writer Name:Dr. Olivia Campbell
Total Orders:753
Satisfaction rate:
Python
Word Count
5883 Words
Writer Name:Dr. David Adam
Total Orders:489
Satisfaction rate:
Python
Word Count
5849 Words
Writer Name:Dr. Nicholas Scott
Total Orders:642
Satisfaction rate:
Python
Word Count
6162 Words
Writer Name:Professor Liam Mitchell
Total Orders:932
Satisfaction rate:
Python
Word Count
3640 Words
Writer Name:Professor Daniel Mitchell
Total Orders:465
Satisfaction rate:
Python
Word Count
4343 Words
Writer Name:Prof. Jackson Ng
Total Orders:627
Satisfaction rate:
Python
Word Count
7272 Words
Writer Name:Dr. Jennifer Carter
Total Orders:879
Satisfaction rate:
Python
Word Count
4577 Words
Writer Name:Dr. Sophia Nguyen
Total Orders:900
Satisfaction rate:
Python
Word Count
4145 Words
Writer Name:Dr. Isabella Scott
Total Orders:754
Satisfaction rate:
Python
Word Count
4193 Words
Writer Name:Dr. Isabella Scott
Total Orders:754
Satisfaction rate:
Python
Word Count
4165 Words
Writer Name:Dr. Ashley
Total Orders:685
Satisfaction rate:
Python
Word Count
4176 Words
Writer Name:Prof. Jackson Ng
Total Orders:627
Satisfaction rate:
Python
Word Count
3922 Words
Writer Name:Dr. Chloe Mitchell
Total Orders:957
Satisfaction rate:
Python
Word Count
4091 Words
Writer Name:Glenn R. Maguire
Total Orders:714
Satisfaction rate:
Python
Word Count
4747 Words
Writer Name:Dr. Sophia Nguyen
Total Orders:900
Satisfaction rate:
Python
Word Count
4594 Words
Writer Name:Dr. Samantha Benet
Total Orders:812
Satisfaction rate:
Python
Word Count
6716 Words
Writer Name:Dr. Isabella Scott
Total Orders:754
Satisfaction rate:
Python
Word Count
4347 Words
Writer Name:Prof. James Harper
Total Orders:664
Satisfaction rate: