Tip of the day
News
Instructions
Objective
Write a Python assignment program to implement a linked list.
Requirements and Specifications
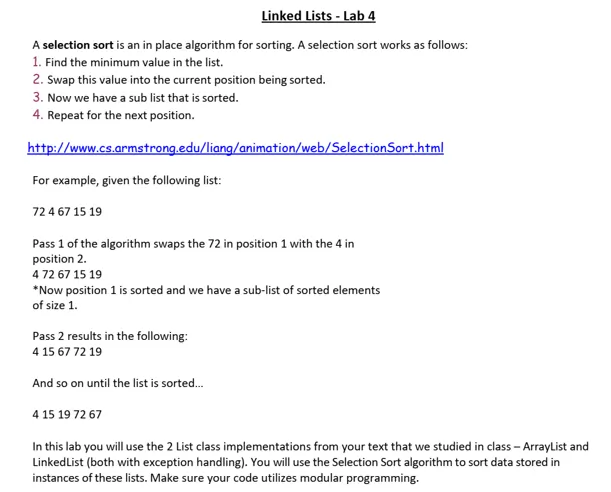
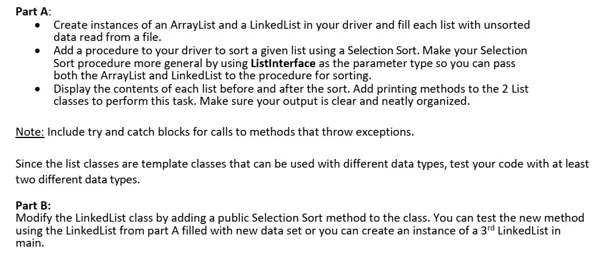
Source Code
// Created by Frank M. Carrano and Timothy M. Henry.
// Copyright (c) 2017 Pearson Education, Hoboken, New Jersey.
/** ADT list: Array-based implementation.
Listing 9-1.
@file ArrayList.h */
#ifndef ARRAY_LIST_
#define ARRAY_LIST_
#include "ListInterface.h"
#include "PrecondViolatedExcep.h"
template
class ArrayList : public ListInterface
{
private:
static const int DEFAULT_CAPACITY = 5; // Small capacity to test for a full list
ItemType items[DEFAULT_CAPACITY+1]; // Array of list items (not using element [0]
int itemCount; // Current count of list items
int maxItems; // Maximum capacity of the list
public:
ArrayList();
// Copy constructor and destructor are supplied by compiler
bool isEmpty() const;
int getLength() const;
bool insert(int newPosition, const ItemType& newEntry);
bool remove(int position);
void clear();
/** @throw PrecondViolatedExcep if position < 1 or
position > getLength(). */
ItemType getEntry(int position) const throw(PrecondViolatedExcep);
/** @throw PrecondViolatedExcep if position < 1 or
position > getLength(). */
void replace(int position, const ItemType& newEntry)
throw(PrecondViolatedExcep);
}; // end ArrayList
#include "ArrayList.cpp"
#endif
Similar Sample
Explore our sample Python assignment to see the high-quality assistance we provide at programminghomeworkhelp.com. Our experts deliver well-documented, error-free solutions tailored to meet your academic needs. Whether you're tackling complex algorithms or data analysis, this sample showcases our commitment to helping you excel in your programming coursework.
Python
Word Count
4091 Words
Writer Name:Walter Parkes
Total Orders:2387
Satisfaction rate:
Python
Word Count
2184 Words
Writer Name:Dr. Jesse Turner
Total Orders:2365
Satisfaction rate:
Python
Word Count
6429 Words
Writer Name:Dr. Olivia Campbell
Total Orders:753
Satisfaction rate:
Python
Word Count
5883 Words
Writer Name:Dr. David Adam
Total Orders:489
Satisfaction rate:
Python
Word Count
5849 Words
Writer Name:Dr. Nicholas Scott
Total Orders:642
Satisfaction rate:
Python
Word Count
6162 Words
Writer Name:Professor Liam Mitchell
Total Orders:932
Satisfaction rate:
Python
Word Count
3640 Words
Writer Name:Professor Daniel Mitchell
Total Orders:465
Satisfaction rate:
Python
Word Count
4343 Words
Writer Name:Prof. Jackson Ng
Total Orders:627
Satisfaction rate:
Python
Word Count
7272 Words
Writer Name:Dr. Jennifer Carter
Total Orders:879
Satisfaction rate:
Python
Word Count
4577 Words
Writer Name:Dr. Sophia Nguyen
Total Orders:900
Satisfaction rate:
Python
Word Count
4145 Words
Writer Name:Dr. Isabella Scott
Total Orders:754
Satisfaction rate:
Python
Word Count
4193 Words
Writer Name:Dr. Isabella Scott
Total Orders:754
Satisfaction rate:
Python
Word Count
4165 Words
Writer Name:Dr. Ashley
Total Orders:685
Satisfaction rate:
Python
Word Count
4176 Words
Writer Name:Prof. Jackson Ng
Total Orders:627
Satisfaction rate:
Python
Word Count
3922 Words
Writer Name:Dr. Chloe Mitchell
Total Orders:957
Satisfaction rate:
Python
Word Count
4091 Words
Writer Name:Glenn R. Maguire
Total Orders:714
Satisfaction rate:
Python
Word Count
4747 Words
Writer Name:Dr. Sophia Nguyen
Total Orders:900
Satisfaction rate:
Python
Word Count
4594 Words
Writer Name:Dr. Samantha Benet
Total Orders:812
Satisfaction rate:
Python
Word Count
6716 Words
Writer Name:Dr. Isabella Scott
Total Orders:754
Satisfaction rate:
Python
Word Count
4347 Words
Writer Name:Prof. James Harper
Total Orders:664
Satisfaction rate: