Instructions
Objective
To complete a Java assignment, you need to write a program to implement linear nodes in the Java language. This task involves creating a program that utilizes linear nodes, a fundamental data structure, in a Java context. By successfully implementing this program, you'll not only enhance your understanding of linear nodes but also demonstrate your proficiency in Java programming. Make sure to apply the concepts you've learned to accomplish this Java assignment effectively.
Requirements and Specifications
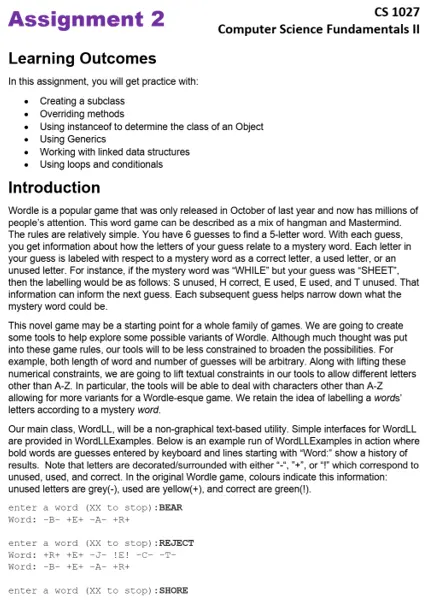
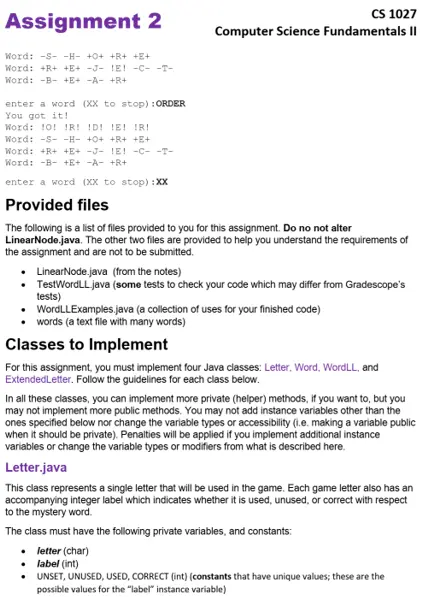
Source Code
EXTENDED LETTER
import java.util.Objects;
public class ExtendedLetter extends Letter {
private static final int SINGLETON = -1;
private String content;
private int family;
private boolean related;
public ExtendedLetter(String s) {
this(s, SINGLETON);
}
public ExtendedLetter(String s, int fam) {
super('a');
content = s;
related = false;
family = fam;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ExtendedLetter that = (ExtendedLetter) o;
if (that.family == family) {
related = true;
}
return content.equals(that.content);
}
@Override
public String toString() {
if (isUnused() && related) {
return "." + content + ".";
}
return decorator() + content + decorator();
}
public static Letter[] fromStrings(String[] content, int[] codes) {
Letter[] result = new Letter[content.length];
for (int i = 0; i
if (codes == null) {
result[i] = new ExtendedLetter(content[i]);
}
else {
result[i] = new ExtendedLetter(content[i], codes[i]);
}
}
return result;
}
}
LETTER
import java.util.Objects;
public class Letter {
private static final int UNSET = 0;
private static final int UNUSED = 1;
private static final int USED = 2;
private static final int CORRECT = 3;
private char letter;
private int label;
public Letter(char c) {
letter = c;
label = UNSET;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Letter letter1 = (Letter) o;
return letter == letter1.letter;
}
public String decorator() {
switch (label) {
case UNSET:
return " ";
case UNUSED:
return "-";
case USED:
return "+";
case CORRECT:
return "!";
default:
throw new IllegalStateException();
}
}
@Override
public String toString() {
return decorator() + letter + decorator();
}
public void setUnused() {
label = UNUSED;
}
public void setUsed() {
label = USED;
}
public void setCorrect() {
label = CORRECT;
}
public boolean isUnused() {
return label == UNUSED;
}
public static Letter[] fromString(String s) {
Letter[] result = new Letter[s.length()];
for (int i = 0; i
result[i] = new Letter(s.charAt(i));
}
return result;
}
}
WORD
public class Word {
private LinearNode firstNode;
public Word(Letter[] letters) {
for (int i = letters.length - 1; i>=0; i--) {
LinearNode newNode = new LinearNode<>(letters[i]);
newNode.setNext(firstNode);
firstNode = newNode;
}
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder("Word: ");
LinearNode current = firstNode;
while(current != null) {
builder.append(current.getElement().toString()).append(" ");
current = current.getNext();
}
return builder.toString();
}
// this is the only method, which is not described strictly in the paper,
// so it is the only one, which must be commented in details.
public boolean labelWord(Word mystery) {
// firstly we set used/unused for all letters in this word
// iterating through all letters in this word
LinearNode current = firstNode;
while(current != null) {
Letter l = current.getElement();
// trying to find current letter 'l' in this word
LinearNode mysteryCurr = mystery.firstNode;
// trying to find letter 'l' in word mystery
while (mysteryCurr != null) {
Letter mysteryL = mysteryCurr.getElement();
if (l.equals(mysteryL)) {
// letter 'l' was found in mystery, setting letter l as used
l.setUsed();
break;
}
mysteryCurr = mysteryCurr.getNext();
}
// if we walked through all the letters in mystery and did not find letter l, setting it as unused
if (mysteryCurr == null) {
l.setUnused();
}
// going to next letter of this word.
// maybe some letters in this word are the same, but we don't care - just making the same
current = current.getNext();
}
// now we need to set letters as 'correct' if they are in a correct place
// we start parallel iterations over both words
current = firstNode;
LinearNode mysteryCurr = mystery.firstNode;
// if there will be no matches, this variable will remain true
boolean equals = true;
// we will iterate until both words are not over
while(current != null && mysteryCurr != null) {
Letter l = current.getElement();
Letter mysteryL = mysteryCurr.getElement();
// comparing current letters for both words. If they match, setting 'correct'
if (l.equals(mysteryL)) {
l.setCorrect();
}
else {
equals = false;
}
// shifting to next letter
current = current.getNext();
mysteryCurr = mysteryCurr.getNext();
}
// if words had different length, setting equals var to false
if (current != null || mysteryCurr != null) {
equals = false;
}
return equals;
}
}
WORD LL
public class WordLL {
private Word mysteryWord;
private LinearNode history;
public WordLL(Word mystery) {
mysteryWord = mystery;
history = null;
}
public boolean tryWord(Word guess) {
// checking if word matches
boolean equals = guess.labelWord(mysteryWord);
// appending new guess to history
LinearNode newWord = new LinearNode<>(guess);
newWord.setNext(history);
history = newWord;
// returning comparing result
return equals;
}
@Override
public String toString() {
LinearNode current = history;
StringBuilder builder = new StringBuilder();
while(current != null) {
builder.append(current.getElement().toString()).append(System.lineSeparator());
current = current.getNext();
}
return builder.toString();
}
}
Similar Samples
Explore our programming homework samples showcasing our expertise in Java, Python, C++, and more. Each example demonstrates our commitment to delivering high-quality solutions tailored to students' needs. Whether it's algorithms, data structures, or web development projects, our samples reflect our proficiency and dedication to academic excellence. See for yourself why students trust us with their programming assignments
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java