Instructions
Objective
Write a C++ assignment program to implement internet service provider.
Requirements and Specifications
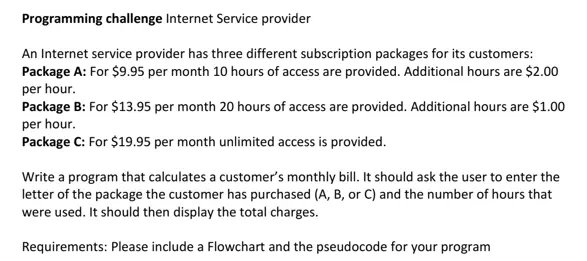
Source Code
#include
#include
using namespace std;
int main() {
// Ask for letter package
string package_name;
while(true) {
cout << "Enter package: ";
cin >> package_name;
if(package_name.compare("A") == 0 || package_name.compare("a") == 0 ||
package_name.compare("B") == 0 || package_name.compare("b") == 0 ||
package_name.compare("C") == 0 || package_name.compare("c") == 0) {
break;
}
else {
cout << "Please enter a valid package name (A, B or C)" << endl;
}
}
// Now, ask for hours
int hours;
while(true) {
cout << "Enter number of hours: ";
cin >> hours;
if(hours > 0) {
break;
}
else {
cout << "Please enter a positive number of hours." << endl;
}
}
// Now, calculate
double monthly_cost;
double hour_cost;
double total_cost;
int max_hours;
if(package_name.compare("A") == 0 || package_name.compare("a") == 0) {
monthly_cost = 9.95;
hour_cost = 2.00;
max_hours = 10;
total_cost = monthly_cost;
// Calculate
if(hours > max_hours) {
total_cost = monthly_cost + (hours - max_hours)*hour_cost;
}
}
else if(package_name.compare("B") == 0 || package_name.compare("b") == 0) {
monthly_cost = 13.95;
hour_cost = 1.00;
max_hours = 20;
total_cost = monthly_cost;
// Calculate
if(hours > 120) {
total_cost = monthly_cost + (hours - max_hours)*hour_cost;
}
}
else // it is package C
{
monthly_cost = 19.95;
hour_cost = 0.00;
total_cost = monthly_cost;
}
// Finally, display
cout << "Your total bill for this month is: $" << total_cost << endl;
}
Similar Samples
"Explore our curated samples at ProgrammingHomeworkHelp.com to witness our expertise in diverse programming assignments. These examples demonstrate our commitment to delivering precise and efficient solutions tailored to your academic or professional requirements. Dive into our samples to experience how we can assist you in mastering programming challenges effectively.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++