Instructions
Requirements and Specifications
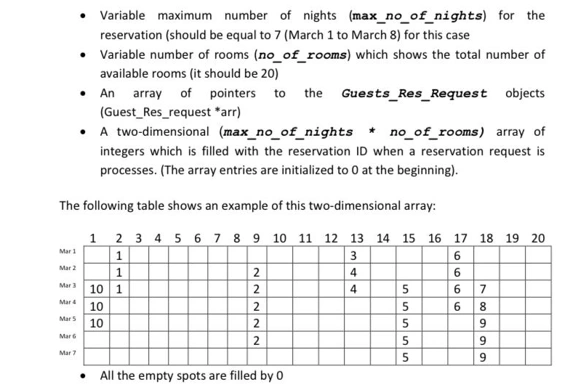
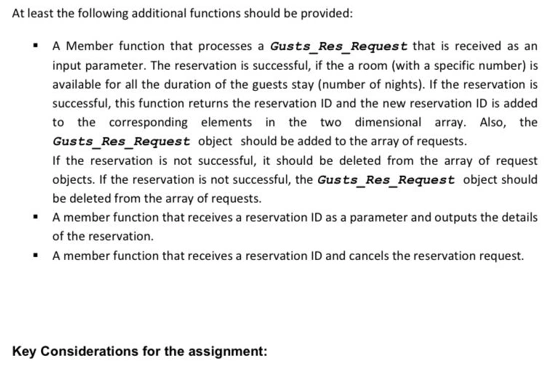
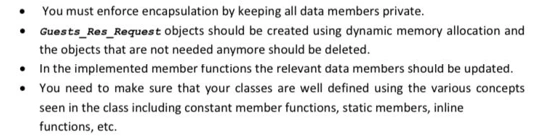
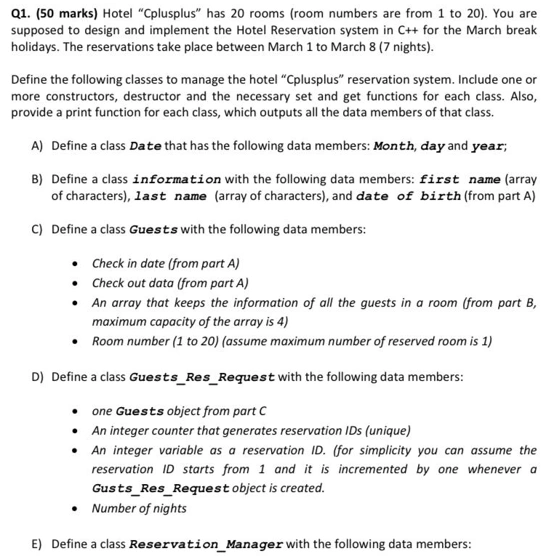
Source Code
GUEST
#include <Date.h>
#include <Information.h>
class Guests
{
private:
Date check_in;
Date check_out;
Information guests_in_room[4];
int room_number;
int n_guests;
public:
// constructors
Guests() {};
Guests(Date in, Date out, int room_n) {
heck_in = in;
check_out = out;
room_number = room_n;
n_guests;
}
void addPerson(Information inf) {
guests_in_room[n_guests++] = inf;
}
// getters and setters
Date getCheckInDate() { return check_in; }
Date getCheckOutDate() { return check_out; }
int getRoomNumber() { return room_number; }
void print() {
for (int i = 0; i < n_guests; i++) {
std::cout << "Person No. " << (i + 1) << ": ";
guests_in_room[i].print();
}
}
};
RESERVATION MANAGER
#include <Guests_Res_Request.h>
class Reservation_Manager
{
private:
int max_no_of_nights;
int no_of_rooms;
Guests_Res_Request* arr;
int requests;
int* reservation_ids;
public:
Reservation_Manager() {};
Reservation_Manager(int max_nights, int n_rooms) {
requests = 0;
max_no_of_nights = max_nights;
no_of_rooms = n_rooms;
reservation_ids = (int*)malloc((max_no_of_nights * no_of_rooms) * sizeof(int));
// initialize with zeros
for (int i = 0; i < max_no_of_nights; i++) {
for (int j = 0; j < no_of_rooms; j++) {
*(reservation_ids + max_no_of_nights * i + j) = 0;
}
}
}
void addGuestRequest(Guests_Res_Request request, int reserv_id) {
bool isAvailable = true;
// check that room is available
Guests guest = request.getGuests();
Date check_in = guest.getCheckInDate();
Date check_out = guest.getCheckOutDate();
// get row id
int start_row_id = check_in.getMonth() * 30 + check_in.getDay();
int end_row_id = check_out.getMonth() * 30 + check_in.getDay();
// Now check if there is a room available for this time
int n_room = -1;
for (int j = 0; j < no_of_rooms; j++) {
isAvailable = true;
for (int i = start_row_id; i <= end_row_id; i++) {
if (*(reservation_ids + max_no_of_nights * i + j) != 0) {
isAvailable = false;
break;
}
}
if (isAvailable) {
n_room = j;
break;
}
}
if (n_room != -1) // a room was found
{
for (int i = start_row_id; i <= end_row_id; i++) {
*(reservation_ids+max_no_of_nights*i+n_room) = request.getReservationId();
arr[requests++] = request;
}
}
}
void printReservationDetails(int reserv_id) {
int request_id = -1;
for (int i = 0; i < requests; i++) {
if (arr[i].getReservationId() == reserv_id) {
Guests_Res_Request r = arr[i];
r.print();
return;
}
}
// if we reach this line it is because no reservation found
std::cout << "Reservation with id " << reserv_id << " not found." << std::endl;
}
void removeReservation(int reserv_id) {
for (int j = 0; j < no_of_rooms; j++) {
for (int i = 0; i < max_no_of_nights; i++) {
if (*(reservation_ids + max_no_of_nights * i + j) == reserv_id) {
*(reservation_ids + max_no_of_nights * i + j) = 0;
}
}
}
}
};
Related Samples
Explore our C++ Assignment Samples featuring meticulously crafted solutions for diverse programming tasks. From fundamental concepts to intricate algorithms, each sample showcases our commitment to clarity, efficiency, and precise coding techniques. Empower your learning and excel in C++ programming with our insightful examples and expert guidance.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++