Instructions
Objective
Write a java assignment to implement GUI.
Requirements and Specifications
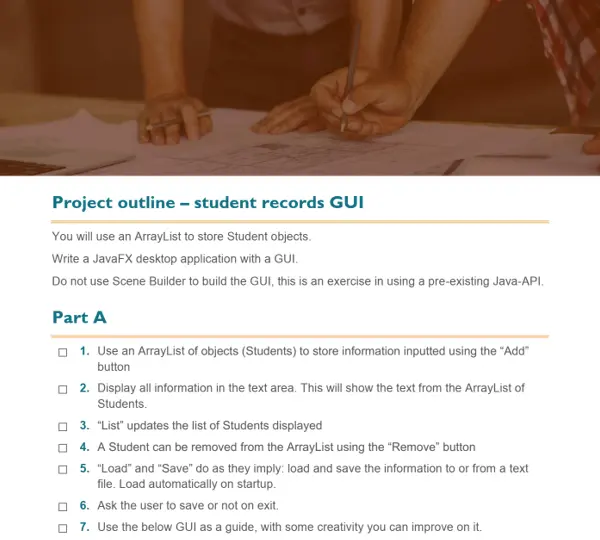
Source Code
APP
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class App extends Application {
public static void main(String[] args) throws Exception {
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
FXMLLoader loader = new FXMLLoader(getClass().getResource("layout.fxml"));
Parent root = loader.load();
Scene scene = new Scene(root, 450, 500);
primaryStage.setTitle("Students App");
primaryStage.setScene(scene);
primaryStage.show();
}
}
STUDENT
import java.util.ArrayList;
import java.util.Dictionary;
import java.util.List;
public class Student {
private String name;
private int StudentID;
private String dob;
private List modules;
/**
* Overloaded constructor.
* @param name String
* @param StudentID int
* @param dob String
*/
public Student(String name, int studentID, String dob) {
this.name = name;
this.StudentID = studentID;
this.dob = dob;
this.modules = new ArrayList();
}
/**
* Getter method for name
* @return String
*/
public String getName() {
return this.name;
}
/**
* Getter method for Student ID
* @return int
*/
public int getStudentID() {
return this.StudentID;
}
/**
* Getter method for Date of birth
* @return String
*/
public String getDoB() {
return this.dob;
}
/**
* Getter method for ModuleRecord list
* @return List of ModuleRecord objects
*/
public List getModules() {
return this.modules;
}
/**
* Method to add a new module to student's records
* @param module ModuleRecord object
*/
public void addModule(ModuleRecord module) {
this.modules.add(module);
}
/**
* Return a String representation of the Student object
* @return String
*/
@Override
public String toString() {
return String.format("Student {name='%s', studentID='%d', dateOfBirth='%s'}", getName(), getStudentID(), getDoB());
}
}
Similar Samples
Browse through our diverse collection of programming homework samples at ProgrammingHomeworkHelp.com. Our examples showcase expertise in various languages such as Python, Java, and C++. These samples are crafted to illustrate our proficiency in delivering clear, well-structured solutions tailored to academic requirements. Explore them to see how we can assist you with your programming challenges effectively.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java