Instructions
Objective
Write a C# assignment program to implement a 'get info' function in C#. This program should focus on creating a function that retrieves information and demonstrates your understanding of C# programming concepts.
Requirements and Specifications
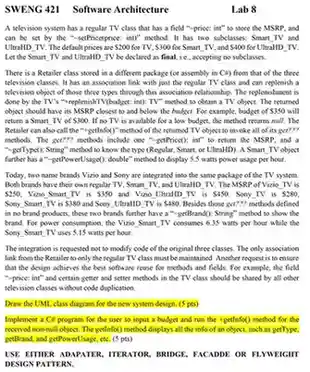
Screenshots
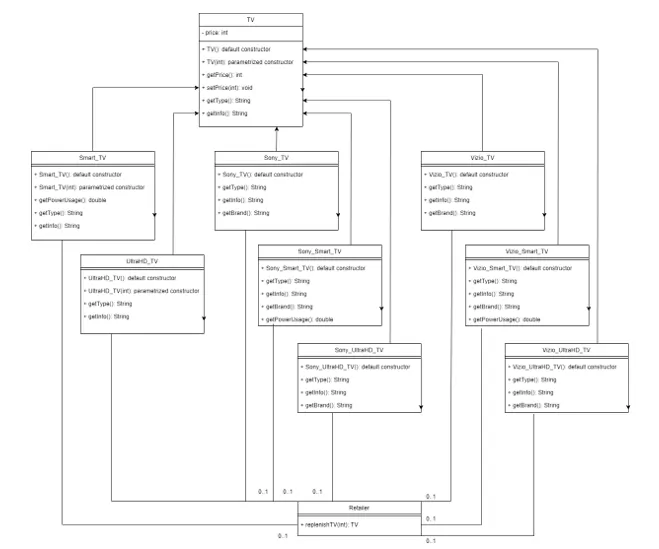
Source Code
PROGRAM
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace AshmeeApp
{
class Program
{
static void Main(string[] args)
{
// Ask user for budget
int budget = 0;
Console.Write("Please enter your budget: $");
budget = Convert.ToInt32(Console.ReadLine());
// Create Retailer object
Retailer retailer = new Retailer();
TV tv = retailer.replenishTV(budget);
if(tv != null) // the tv object returned by Retailer is not null
{
// Display TV info
Console.WriteLine("With a budget of $" + budget + " you can acquire the following TV:");
Console.WriteLine(tv.getInfo());
}
// Execute a Console.Read so console is not closed until user presses a key
Console.Read();
}
}
}
RETAILER
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace AshmeeApp
{
class Retailer
{
public TV replenishTV(int budget)
{
TV tvObject = null;
if (budget >= 200)
tvObject = new TV();
if (budget >= 250)
tvObject = new Vizio_TV();
if (budget >= 280)
tvObject = new Sony_TV();
if (budget >= 300)
tvObject = new Smart_TV();
if (budget >= 350)
tvObject = new Vizio_Smart_TV();
if (budget >= 380)
tvObject = new Sony_Smart_TV();
if (budget >= 400)
tvObject = new UltraHD_TV();
if (budget >= 450)
tvObject = new Vizio_UltraHD_TV();
if (budget >= 480)
tvObject = new Sony_UltraHD_TV();
return tvObject;
}
}
}
Related Samples
At ProgrammingHomeworkHelp.com, we understand the challenges students face with C# assignments. That's why we offer a range of related sample assignments to guide and support your studies. Our expert-crafted samples cover various C# topics, from basic syntax to advanced object-oriented programming concepts, helping you grasp complex ideas and improve your coding skills. Whether you need help with a specific problem or want to understand C# better, our website provides comprehensive resources to assist you in achieving academic success. Let us help you excel in your C# assignments today!
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming