Instructions
Objective
Write a C++ assignment program to implement geometric shapes.
Requirements and Specifications
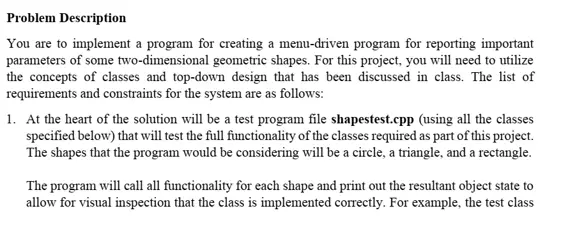
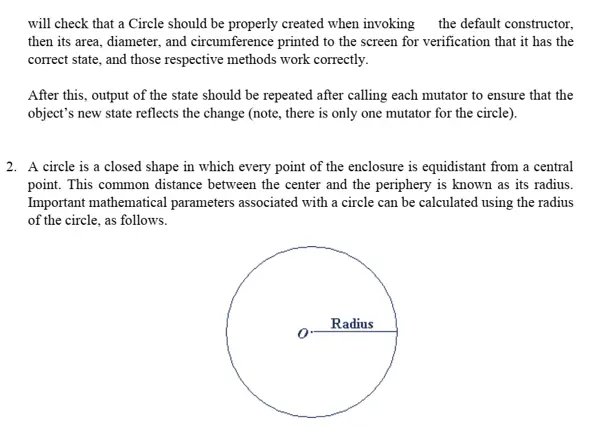
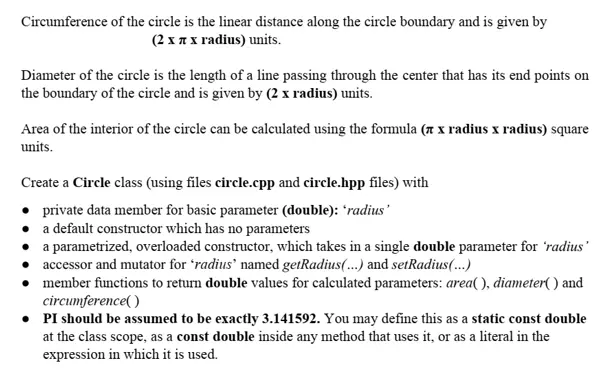
Source Code
CIRCLE
#include "Circle.hpp"
Circle::Circle()
{
radius = 1.0;
}
Circle::Circle(double rad) // parametrized constructor
{
radius = rad;
}
// getters
double Circle::getRadius() {return radius;}
// setters
void Circle::setRadius(double newrad) {radius = newrad;}
// methods
double Circle::area() {return PI*radius*radius;}
double Circle::circunference() {return 2*PI*radius;}
double Circle::diameter() {return 2*radius;}
bool Circle::compareWithRect(Rectangle rect)
{
return rect.area() == area();
}
RECTANGLE
#include "rectangle.hpp"
#include
Rectangle::Rectangle()
{
length = 1.0;
width = 1.0;
}
Rectangle::Rectangle(double l, double w)
{
length = l;
width = w;
}
// getters
double Rectangle::getLength() {return length;}
double Rectangle::getWidth() {return width;}
// setters
void Rectangle::setLength(double newlength) {length = newlength;}
void Rectangle::setWidth(double newwidth) {width = newwidth;}
// methods
double Rectangle::area() {return length*width;}
double Rectangle::diagonal() {return sqrt(pow(length, 2) + pow(width, 2));}
double Rectangle::perimeter() {return 2*(length + width);}
SHAPETEST
#include
#include "circle.cpp"
#include "triangle.cpp"
#include "rectangle.cpp"
using namespace std;
void display_menu()
{
// Function to display all the options from the menu
cout << "1. Create Circle" << endl;
cout << "2. Create Triangle" << endl;
cout << "3. Create Rectangle" << endl;
cout << "4. Quit" << endl;
}
int get_option()
{
// This function asks to user to enter a menu option.
// If the option is not valid, the function will keep prompting the user until a valid option is entered
int option;
while(true) // infinite loop
{
cout << "Please enter an option: ";
cin >> option;
if(option >= 1 && option <= 4) // option is valid
return option;
else // option is invalid
cout << "Invalid option." << endl;
}
}
char get_yes_no()
{
// This function asks to user for Yes (y) or No (N)
char option;
while(true)
{
cout << "Do you want to enter the parameters of the shape (Y) or use default values (N)? (Y/N): ";
cin >> option;
if(option == 'y' ||option == 'Y' ||option == 'n' ||option == 'N')
return option;
else
cout << "Invalid option." << endl;
}
}
double get_double()
{
// This function prompts user for a double value that is positive
double option;
while(true)
{
cin >> option;
if(option > 0)
return option;
else
cout << "Please enter only positive values." << endl;
}
}
int main()
{
bool running = true; // variable used for the while-loop to keep the program running
int option; // store user's numeric option here
char optionchar; // store user's char input here
double radius, base, height, length, width; // double variable to store shapes parameters
while(running)
{
display_menu();
option = get_option();
if(option == 1) // create a circle
{
// Create circle
Circle circle;
// Ask if he/she wants to enter the parameters or use default values
optionchar = get_yes_no();
if(optionchar == 'y' || optionchar == 'Y') // user wants to enter the parameters
{
cout << "Enter radius: ";
radius = get_double();
// Call parametrized constructor
circle = Circle(radius);
//circle.setRadius(radius);
}
// if the option is No, then the circle object created already has default values
// Display all info about the circle
cout << "***** Circle *****" << endl;
cout << "Radius: " << circle.getRadius() << endl;
cout << "Diameter: " << circle.diameter() << endl;
cout << "Area: " << circle.area() << endl;
cout << "Circumference: " << circle.circunference() << endl;
cout << endl;
}
else if(option == 2) // Triangle
{
// Create Triangle
Triangle triangle;
// Ask if he/she wants to enter the parameters or use default values
optionchar = get_yes_no();
if(optionchar == 'y' || optionchar == 'Y') // user wants to enter the parameters
{
cout << "Enter base: ";
base = get_double();
cout << "Enter height: ";
height = get_double();
// Call parametrized constructor
triangle = Triangle(base, height);
//triangle.setBase(base);
//triangle.setHeight(height);
}
// if the option is No, then the circle object created already has default values
// Display all info about the circle
cout << "***** Triangle *****" << endl;
cout << "Base: " << triangle.getBase() << endl;
cout << "Height: " << triangle.getHeight() << endl;
cout << "Area: " << triangle.area() << endl;
cout << endl;
}
else if(option == 3) // Rectangle
{
// Create Rectangle
Rectangle rectangle;
// Ask if he/she wants to enter the parameters or use default values
optionchar = get_yes_no();
if(optionchar == 'y' || optionchar == 'Y') // user wants to enter the parameters
{
cout << "Enter length: ";
length = get_double();
cout << "Enter width: ";
width = get_double();
rectangle = Rectangle(length, width);
}
// if the option is No, then the circle object created already has default values
// Display all info about the circle
cout << "***** Rectangle *****" << endl;
cout << "Length: " << rectangle.getLength() << endl;
cout << "Width: " << rectangle.getWidth() << endl;
cout << "Area: " << rectangle.area() << endl;
cout << "Diagonal: " << rectangle.diagonal() << endl;
cout << "Perimeter: " << rectangle.perimeter() << endl;
cout << endl;
}
else if(option == 4)
{
cout << "Good bye!" << endl;
running = false;
}
}
return 0;
}
TRIANGLE
#include "Triangle.hpp"
Triangle::Triangle()// default constrcutor
{
base = 1.0;
height = 1.0;
}
Triangle::Triangle(double b, double h) // parametrized constructor
{
base = b;
height = h;
}
// getters
double Triangle::getBase() {return base;}
double Triangle::getHeight() {return height;}
// setters
void Triangle::setBase(double newbase) {base = newbase;}
void Triangle::setHeight(double newheight) {height = newheight;}
// methods
double Triangle::area() {return base*height/2.0;}
Related Samples
Explore our free C++ assignment samples for a clear perspective on tackling complex problems. These examples offer detailed solutions and insights, enhancing your understanding and boosting your academic performance.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++