Instructions
Requirements and Specifications
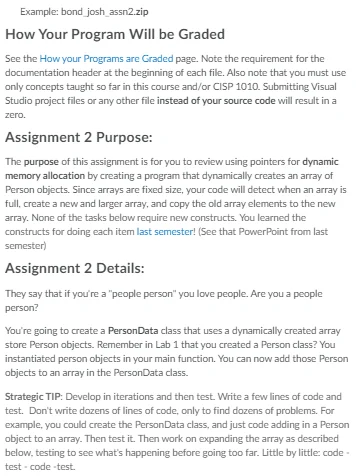
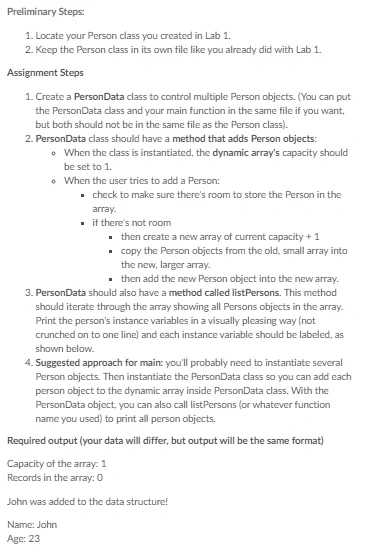
Source Code
#pragma once
#include <string.h>
using namespace std;
class Person {
private:
string myName;
int myAge;
bool superHero;
double myLastMeal;
public:
bool super;
// CREATING GETTERS AND SETTERS//
void setname(string name) {
this->myName = name;
}
string getname() {
return myName;
//Some Constructors//
}
void setage(int age) {
this->myAge = age;
}
int getAge() {
return myAge;
}
void setSuper(int super) {
this->superHero = super;
}
bool getSuper() {
return superHero;
}
void setMeal(double meal) {
this->myLastMeal = meal;
}
double getMeal() {
return myLastMeal;
}
string changeName() {
string name2 = "Harold";
this->myName = name2;
return myName;
}
int changeAge() {
this->myAge = 42;
return myAge;
}
double changeMeal() {
this->myLastMeal = 371.48;
return myLastMeal;
}
bool changeSuper() {
if (superHero == 1) {
this ->superHero = 0;
}
if (superHero == 0) {
this ->superHero = 1;
}
return superHero;
}
};
Related Samples
Delve into our C++ Assignments samples, offering insights into topics such as classes, inheritance, pointers, and algorithms. These meticulously crafted solutions serve as invaluable resources for students navigating C++ programming challenges, fostering comprehension and boosting academic success.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++