Instructions
Objective
Write a python assignment program to implement data reading of body weights of terrestrial mammals.
Requirements and Specifications
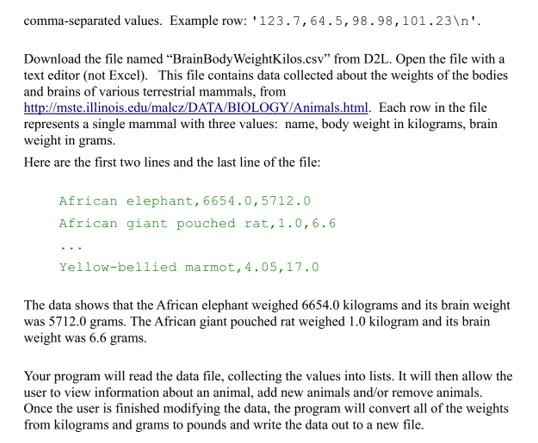
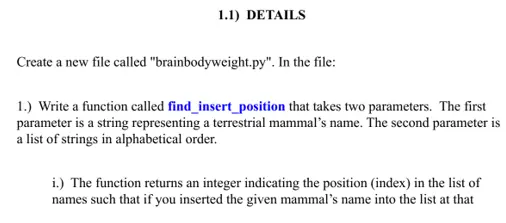
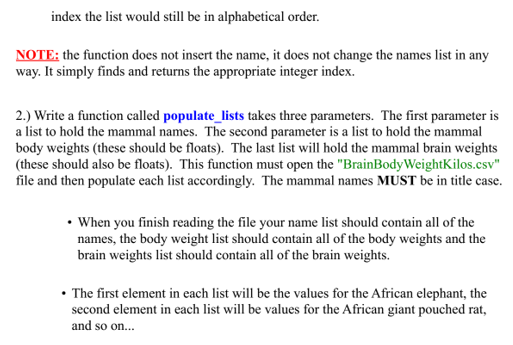
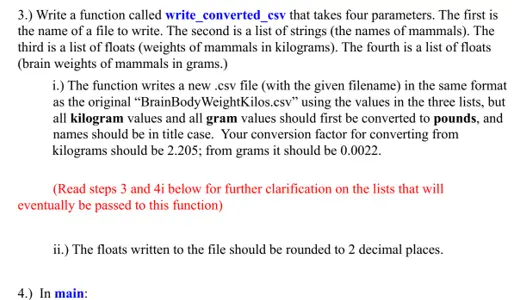
Source Code
def find_insert_position(mammal_name, listofnames):
# If the list is empty, return 0
if len(listofnames) == 0:
return 0
for i, element in enumerate(listofnames):
if element > mammal_name:
return i
# If there are no elements in the list higher than the given name, it means
# that the given name must be inserted at the end
return len(listofnames)
def populate_lists(listofnames, listofbodyweights, listofbrainweights):
# Open file
data = open('BrainBodyWeightKilos.csv', 'r')
# Read lines
lines = data.readlines()
# Loop through lines
for line in lines:
# Strip
line = line.strip()
# Split
row = line.split(',')
# Take the name, convert to title case
name = row[0].lower()
name = name[0].upper() + name[1:]
# Take the body weight and convert to float
body_weight = float(row[1])
# Take Brain weight and convert to float
brain_weight = float(row[2])
# Now, append to lists
# Find index at which they must be inserted
idx = find_insert_position(name, listofnames)
listofnames.insert(idx, name)
listofbodyweights.insert(idx, body_weight)
listofbrainweights.insert(idx, brain_weight)
# Close file
data.close()
def write_converted_csv(filename, listofnames, listofbodyweights, listofbrainweights):
# Define the conversion factor from Kilograms to Pounds
LBS_IN_KG = 2.205
LBS_IN_G = 0.0022
# First, open the file in write mode
file = open(filename, 'w+') # create in case it does not exists
# Get number of records
n = len(listofnames) # all three lists should have the same number of records
# Now, loop through all elements in the lists
for i in range(n):
name = listofnames[i]
body_weight_kg = listofbodyweights[i]
brain_weight_g = listofbrainweights[i]
# Convert body weight from kg to lb
body_weight_lb = body_weight_kg*LBS_IN_KG
# Convert brain weight from grams to lb
brain_weight_lb = brain_weight_g*LBS_IN_G # to lbs
# Now, write to file
file.write('{0},{1:.2f},{2:.2f}\n'.format(name, body_weight_lb, brain_weight_lb))
# Finally, close the file
file.close()
def main():
# Lists to store names and weights
listofnames = list()
listofbodyweights = list()
listofbrainweights = list()
# Populate
populate_lists(listofnames, listofbodyweights, listofbrainweights)
# Now repeatedly ask for animal name
while True:
name = input('Enter animal name (or "q" to quit): ')
# Convert to title case
name = name.lower()
name = name[0].upper() + name[1:]
if name == 'Q':
# Write data to csv
write_converted_csv('BrainBodyWeightPounds.csv', listofnames, listofbodyweights, listofbrainweights)
break
else:
if name in listofnames: # Name is in the list of names
# Find and print
idx = listofnames.index(name)
body_weight = listofbodyweights[idx]
brain_weight = listofbrainweights[idx]
print('{0}: body = {1:.2f}, brain = {2:.2f}'.format(name, body_weight, brain_weight))
delete = input(f'Delete "{name}"? (y|n) ')
if delete == 'y':
del listofnames[idx]
del listofbodyweights[idx]
del listofbrainweights[idx]
else: # Name not in list
print(f'File does not contain "{name}".')
add = input(f'Add "{name}" to file? (y|n) ')
if add == 'y':
# Ask for body weight
body_weight = float(input(f'Enter body weight for "{name}" in kilograms: '))
brain_weight = float(input(f'Enter brain weight for {name} in grams: '))
# Now, insert
idx = find_insert_position(name, listofnames)
listofnames.insert(idx, name)
listofbodyweights.insert(idx, body_weight)
listofbrainweights.insert(idx, brain_weight)
print()
if __name__ == '__main__':
main()
Similar Samples
Explore our programming homework samples to see the high-quality solutions we deliver. Each example showcases our expertise in various programming languages and our commitment to accuracy and detail. Discover how our professional assistance can help you excel in your programming assignments.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python