Instructions
Objective
Write a C++ assignment program to implement buffer overflows.
Requirements and Specifications
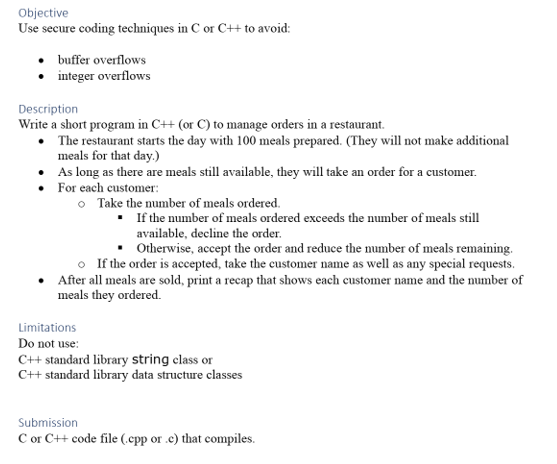
Source Code
#include
#include
using namespace std;
const int MAX_MEAL = 100;
const int MAX_NAME_LEN = 20;
// Holds a customer data and the next customer after it (Linked list)
struct Customer
{
char name[MAX_NAME_LEN];
int numOrders;
Customer *next;
// Initialize a customer
Customer()
{
numOrders = 0;
next = NULL;
}
};
// Make sure to read a positive int that doesn't overflow
// Since this is a simple application, we shouldn't retrieve an input
// that is more than 100 or below 0. This we we protect the app
// from under or overflow
int readNumOrders()
{
while (1)
{
cout << "Enter the number of meals to order for next customer: ";
int value;
// Make sure it's within bounds. When the user enters a very very very
// large number, it will overflow and it will wrap around but we only
// accept values that is within 1 to 100 to make the app still work
// as expected
if (cin >> value)
{
cin.clear();
cin.ignore();
if (value >= 1 && value <= MAX_MEAL)
return value;
}
else
{
cin.clear();
cin.ignore();
}
cout << "Invalid value." << endl;
}
}
// Read a name making sure that the number of characters is within the buffer
// bounds. If an overflow is to happen, we will reject all other incoming characters
void readName(char *name)
{
cout << "Enter customer's name: ";
int i;
bool overflowed = true;
for (i = 0; i < MAX_NAME_LEN - 1; i++)
{
char c = fgetc(stdin);
if (c == '\n')
{
overflowed = false;
break;
}
name[i] = c;
}
name[i] = '\0';
if (overflowed)
{
// Delete all other incoming characters until we get the new line
char c;
while ((c = fgetc(stdin)) != '\n');
}
}
// Entry point of the program
int main()
{
Customer *lastCustomer = NULL;
// Start with 100 meals prepared
int mealsLeft = MAX_MEAL;
// As long as there are meals still available, hey will take an order for a
// customer
while (mealsLeft > 0)
{
cout << "There are " << mealsLeft << " orders left..." << endl;
Customer *customer = new Customer();
customer->numOrders = readNumOrders();
readName(customer->name);
if (customer->numOrders <= 0 || customer->numOrders > mealsLeft)
{
// If the number of meals ordered exceeds the number of meals still
// available, decline the order.
cout << "Invalid number of orders." << endl;
delete customer;
}
else
{
// Otherwise, accept the order and reduce the number of meals remaining.
mealsLeft -= customer->numOrders;
customer->next = lastCustomer;
lastCustomer = customer;
}
}
// After all meals are sold, print a recap that shows each customer name and the number of
// meals they ordered.
cout << endl;
cout << "Orders from latest to oldest:" << endl;
while (lastCustomer != NULL)
{
cout << lastCustomer->name << ": " << lastCustomer->numOrders << endl;
Customer *nextCustomer = lastCustomer->next;
delete lastCustomer;
lastCustomer = nextCustomer;
}
return 0;
}
Related Samples
Explore our C++ programming homework samples to see how we tackle complex assignments with clarity and precision. Our curated examples demonstrate expertise in algorithms, data structures, object-oriented concepts, and more. Trust ProgrammingHomeworkHelp.com for top-notch solutions tailored to your academic needs.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++