Instructions
Objective
If you're looking to complete a C++ assignment, one intriguing task could be to write a program that implements binary trees. Binary trees are fundamental data structures in computer science, serving various purposes such as efficient searching, sorting, and organizing hierarchical data. By designing and coding a binary tree in C++, you'll not only gain a deeper understanding of data structures but also strengthen your programming skills. This assignment offers an opportunity to apply core concepts like nodes, traversal methods (such as in-order, pre-order, and post-order), insertion, and deletion operations. So, roll up your sleeves, engage with the intricacies of binary trees, and complete your C++ assignment with confidence.
Requirements and Specifications
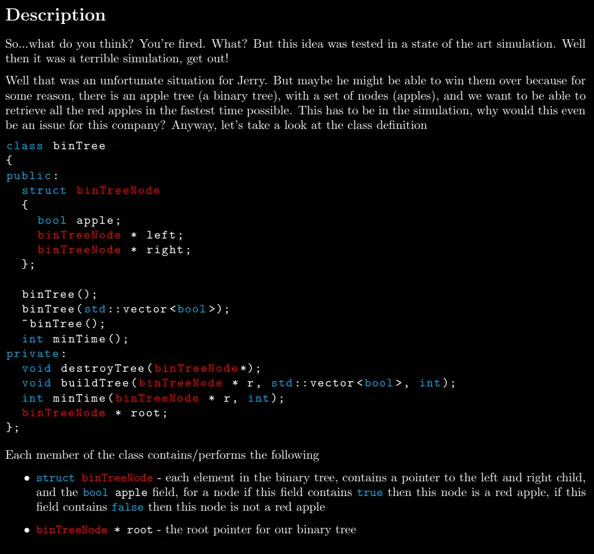
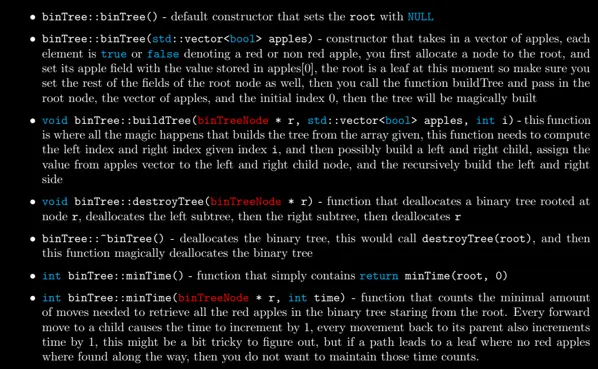
Source Code
#include "binTree.h"
binTree::binTree() {
root = NULL;
}
binTree::binTree(std::vector apples) {
buildTree(root, apples, 0);
}
binTree::~binTree() {
destroyTree(root);
}
void binTree::insert(bool item) {
binTreeNode *newNode = new binTreeNode;
newNode->apple = item;
newNode->left = NULL;
newNode->right = NULL;
binTreeNode *parent = new binTreeNode;
int height = 0;
if(root == NULL) {
root = newNode;
//std::cout << "Root at height 0 " << std::endl;
} else {
binTreeNode* ptr = new binTreeNode;
ptr = root;
while(ptr != NULL) {
parent = ptr;
if(item >= ptr->apple) {
ptr = ptr->right;
} else {
ptr = ptr->left;
}
height++;
}
if(item < parent->apple) {
parent->left = newNode;
//std::cout << "Inserted " << item << " on left at height " << height << std::endl;
} else {
parent->right = newNode;
//std::cout << "Inserted " << item << " on right at height " << height << std::endl;
}
}
}
void binTree::buildTree(binTreeNode * r, std::vector apples, int height) {
// We start inserting and index height+1
for(int i = height; i < apples.size(); i++) {
insert(apples[i]);
}
}
void binTree::destroyTree(binTreeNode *r) {
if(r == NULL) {
return;
}
// Dealocate the left subtree
binTreeNode* node = r->left;
binTreeNode* tmp;
while(node != NULL) {
tmp = node;
node = node->left;
tmp = NULL;
}
// Dealocate the right subtree
node = r->right;
while(node != NULL) {
tmp = node;
node = node->right;
tmp = NULL;
}
}
int binTree::minTime() {
return 2*minTime(root, 0);
}
int binTree::minTime(binTreeNode* r, int time) {
// If there are no more elements to the right, we reached the end
// of the red apples
if(r->right == NULL) {
// If the current element is false, it means there are no red apples, so
// do not count the moves in the branch
if(!r->apple) {
return 0;
}
else {
// return the number of steps count plus one
return time;
}
}
return minTime(r->right, time+1);
}
Similar Samples
Explore our sample programming assignments showcasing our expertise in Python, Java, C++, and more. Our carefully crafted samples illustrate the quality and precision we bring to every project. Whether you're a student or a professional, these examples demonstrate our commitment to delivering top-notch programming solutions tailored to your needs.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++