Instructions
Objective
Write a JAVA assignment program to implement arrays to solve problems.
Requirements and Specifications
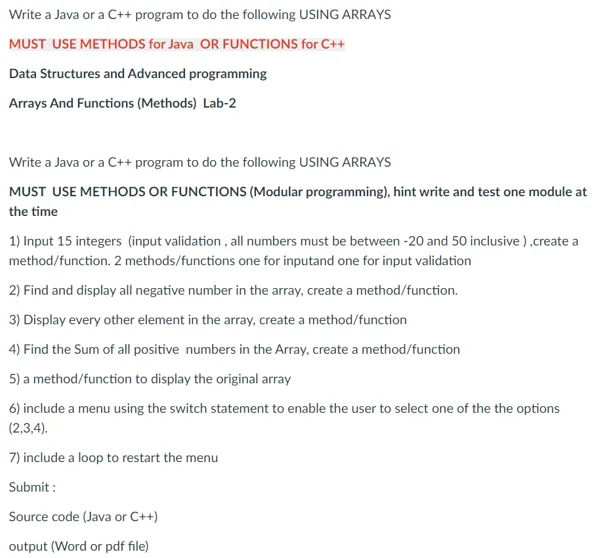
Source Code
#include
using namespace std;
bool check(int v) {
return (-20 <= v) && (v <= 50);
}
void read15Ints(int* arr, int n) {
int counter = 0;
int val;
while(counter < n) {
cin >> val;
if (check(val)) {
arr[counter] = val;
counter++;
}
else {
cout << "Input value is out of bounds" << endl;
}
}
}
void showAllBySign(int* arr, int n, bool negative) {
cout << (negative ? "Negatives:" : "Non-negatives:");
for (int i = 0; i
if (arr[i] < 0 && negative || arr[i] >= 0 && !negative) {
cout << " " << arr[i];
}
}
cout << endl;
}
void showPositiveSum(int* arr, int n) {
cout << "Positive sum:";
int sum = 0;
for (int i = 0; i
if (arr[i] > 0) {
sum += arr[i];
}
}
cout << sum << endl;
}
void showArray(int* arr, int n) {
cout << "Array:";
for (int i = 0; i
cout << " " << arr[i];
}
cout << endl;
}
int main() {
int arr[15], n = 15;
bool isOver = false;
read15Ints(arr, n);
while (!isOver) {
int choice = 0;
cout << "Menu:" << endl;
cout << "1. Show all negatives" << endl;
cout << "2. Show all non-negatives" << endl;
cout << "3. Show positive sum" << endl;
cout << "4. Show original array" << endl;
cout << "5. Exit" << endl;
cout << "Your choice: ";
cin >> choice;
switch(choice) {
case 1:
showAllBySign(arr, n, true);
break;
case 2:
showAllBySign(arr, n, false);
break;
case 3:
showPositiveSum(arr, n);
break;
case 4:
showArray(arr, n);
break;
case 5:
isOver = true;
break;
default:
cout << "Invalid input" << endl;
break;
}
cout << endl;
}
cout << "Good Bye" << endl;
}
Related Samples
Explore our Java programming homework samples to see how we tackle complex concepts with clarity and precision. Our curated examples showcase our expertise in Java programming, offering insights into problem-solving, code optimization, and more. Whether you're studying arrays, inheritance, or GUIs, these samples demonstrate our commitment to delivering high-quality solutions tailored to your academic needs.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java