Instructions
Objective
Write a C++ assignment program to implement 2D array.
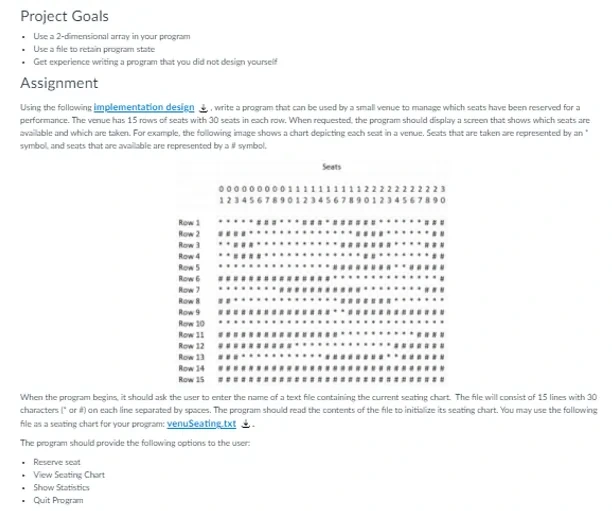
#include
#include
#include
#include
#include
using namespace std;
const int N_ROWS = 15;
const int N_SEATS = 30;
const int MENU_CHOICE_RESERVE = 1;
const int MENU_CHOICE_DISPLAY = 2;
const int MENU_CHOICE_SHOW_STATS = 3;
const int MENU_CHOICE_QUIT = 4;
const char SEAT_AVAILABLE = '#';
const char SEAT_RESERVED = '*';
void saveReservationState(string stateFileName, char reservations[N_ROWS][N_SEATS]);
bool loadReservationState(string stateFileName, char reservations[N_ROWS][N_SEATS]);
int getMenuChoice();
void processChoice(int choice, char reservations[N_ROWS][N_SEATS]);
bool makeReservation(char reservations[N_ROWS][N_SEATS]);
bool getSeat(int &row, int &seat);
void displayReservationState(char reservations[N_ROWS][N_SEATS]);
void calculateReservationStatistics(char reservations[N_ROWS][N_SEATS]);
int main()
{
char reservations[N_ROWS][N_SEATS];
cout << "Enter reservations state filename: ";
string stateFileName;
getline(cin, stateFileName);
if (!loadReservationState(stateFileName, reservations))
{
cout << "The reservations state file is invalid.\n";
return 1;
}
int menuChoice;
do
{
menuChoice = getMenuChoice();
if (menuChoice != MENU_CHOICE_QUIT)
{
processChoice(menuChoice, reservations);
}
} while (menuChoice != MENU_CHOICE_QUIT);
saveReservationState(stateFileName, reservations);
return 0;
}
void saveReservationState(string stateFileName, char reservations[N_ROWS][N_SEATS])
{
ofstream file(stateFileName.c_str());
for (int i = 0; i < N_ROWS; i++)
{
for (int j = 0; j < N_SEATS; j++)
{
file << reservations[i][j] << " ";
}
file << "\n";
}
file.close();
}
bool loadReservationState(string stateFileName, char reservations[N_ROWS][N_SEATS])
{
ifstream file(stateFileName.c_str());
if (!file.is_open())
{
return false;
}
for (int i = 0; i < N_ROWS; i++)
{
for (int j = 0; j < N_SEATS; j++)
{
char seat;
if (file >> seat && (seat == SEAT_RESERVED || seat == SEAT_AVAILABLE))
{
reservations[i][j] = seat;
}
else
{
file.close();
return false;
}
}
}
file.close();
return true;
}
int getMenuChoice()
{
cout << "1. Reserve Seats\n"
"2. View Seating Chart\n"
"3. Show Statistics\n"
"4. Quit\n"
"Choice: ";
int menuChoice;
cin >> menuChoice;
return menuChoice;
}
void processChoice(int choice, char reservations[N_ROWS][N_SEATS])
{
if (choice == MENU_CHOICE_RESERVE)
{
makeReservation(reservations);
}
else if (choice == MENU_CHOICE_DISPLAY)
{
displayReservationState(reservations);
}
else if (choice == MENU_CHOICE_SHOW_STATS)
{
calculateReservationStatistics(reservations);
}
else
{
cout << "Unknown choice\n";
}
}
bool makeReservation(char reservations[N_ROWS][N_SEATS])
{
displayReservationState(reservations);
cout << "How many seats do you want to reserve? ";
int requiredSeats;
cin >> requiredSeats;
for (int i = 0; i < requiredSeats;)
{
int rowNumber;
int seatNumber;
if (getSeat(rowNumber, seatNumber))
{
if (reservations[rowNumber][seatNumber] == SEAT_AVAILABLE)
{
reservations[rowNumber][seatNumber] = SEAT_RESERVED;
i++;
}
else
{
cout << "Seat is currently taken.\n";
}
}
}
return true;
}
bool getSeat(int &row, int &seat)
{
cout << "Enter row # and seat # of the seat to reserve: ";
int rowNumber;
int seatNumber;
cin >> rowNumber >> seatNumber;
if (rowNumber >= 1 && rowNumber <= N_ROWS
&& seatNumber >= 1 && seatNumber <= N_SEATS)
{
row = rowNumber - 1;
seat = seatNumber - 1;
return true;
}
cout << "Seat out of range.\n";
return false;
}
void displayReservationState(char reservations[N_ROWS][N_SEATS])
{
cout << "Seats" << endl;
cout << " ";
for (int i = 1; i <= N_SEATS; i++)
{
cout << (i / 10) << " ";
}
cout << endl;
cout << " ";
for (int i = 1; i <= N_SEATS; i++)
{
cout << (i % 10) << " ";
}
cout << endl;
for (int i = 0; i < N_ROWS; i++)
{
cout << "Row " << (i + 1);
if (i + 1< 10)
{
cout << " ";
}
cout << " ";
for (int j = 0; j < N_SEATS; j++)
{
cout << reservations[i][j] << " ";
}
cout << "\n";
}
}
void calculateReservationStatistics(char reservations[N_ROWS][N_SEATS])
{
int reserved = 0;
for (int i = 0; i < N_ROWS; i++)
{
for (int j = 0; j < N_SEATS; j++)
{
if (reservations[i][j] == SEAT_RESERVED)
{
reserved++;
}
}
}
cout << "Total Seats Reserved: " << reserved << "\n"
"Total Seats Available: " << (N_ROWS * N_SEATS - reserved) << "\n"
"Number of Seats available by row:\n";
for (int i = 0; i < N_ROWS; i++)
{
reserved = 0;
for (int j = 0; j < N_SEATS; j++)
{
if (reservations[i][j] == SEAT_RESERVED)
{
reserved++;
}
}
cout << "\tRow " << (i + 1);
if (i + 1< 10)
{
cout << " ";
}
cout << ": " << (N_SEATS - reserved) << "\n";
}
}
Similar Samples
Our samples are designed to showcase our expertise in solving assignments, providing you with a clear understanding of the quality and depth of our work. Explore our C++ samples to see how we handle complex programming tasks with precision and efficiency.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++