Instructions
Objective
Write a program that allows users to fork 2 processes and then exit when they complete in C language.
Requirements and Specifications
Process Activity – Programming Language: C
- Create a program (forkchildren.c) that will fork off two children.
- One child will run the “ls -l” command
- The other will run “cat forkchildren.c”
- The main program will wait for the children to finish and then print a note saying it is finished and then it will end.
- No pipe is needed for this assignment, each child is separate.
Screenshots of output
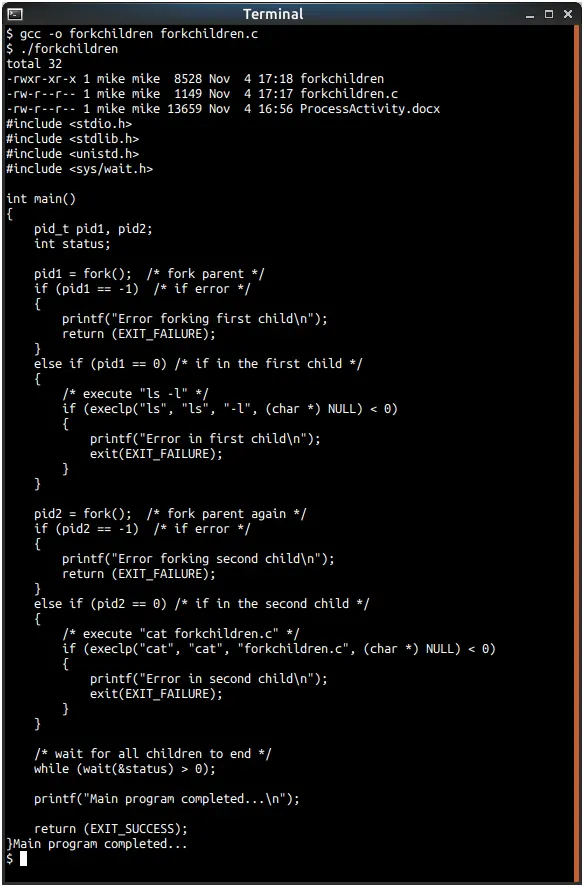
Source Code
#include
#include
#include
#include
int main()
{
pid_t pid1, pid2;
int status;
pid1 = fork(); /* fork parent */
if (pid1 == -1) /* if error */
{
printf("Error forking first child\n");
return (EXIT_FAILURE);
}
else if (pid1 == 0) /* if in the first child */
{
/* execute "ls -l" */
if (execlp("ls", "ls", "-l", (char *) NULL) < 0)
{
printf("Error in first child\n");
exit(EXIT_FAILURE);
}
}
pid2 = fork(); /* fork parent again */
if (pid2 == -1) /* if error */
{
printf("Error forking second child\n");
return (EXIT_FAILURE);
}
else if (pid2 == 0) /* if in the second child */
{
/* execute "cat forkchildren.c" */
if (execlp("cat", "cat", "forkchildren.c", (char *) NULL) < 0)
{
printf("Error in second child\n");
exit(EXIT_FAILURE);
}
}
/* wait for all children to end */
while (wait(&status) > 0);
printf("Main program completed...\n");
return (EXIT_SUCCESS);
}
Similar Samples
At ProgrammingHomeworkHelp.com, we specialize in providing expert assistance for all your programming assignments. Whether you're tackling C++, Java, Python, or any other language, our team of experienced programmers ensures top-quality solutions tailored to your needs. With a commitment to accuracy and timely delivery, we're here to help you excel in your programming endeavors. Explore our services today!"
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C