Instructions
Objective
If you require assistance with a Java assignment, here's a program that you might find helpful. The task is to write a Java program that finds specific cards in a deck. To accomplish this, you can create a class representing a card with attributes like suit and value. Then, create a deck as an array or a collection of card objects. Implement a method to search for cards based on given criteria, such as suit or value.
Requirements and Specifications
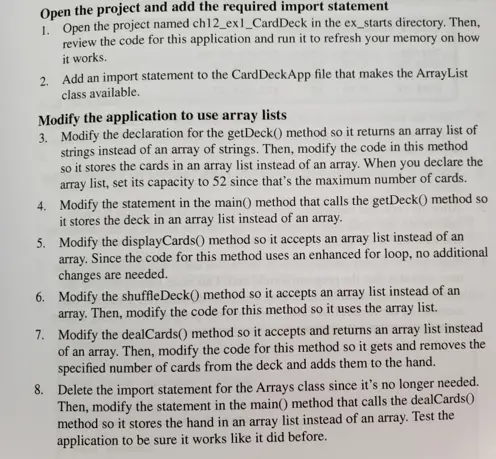
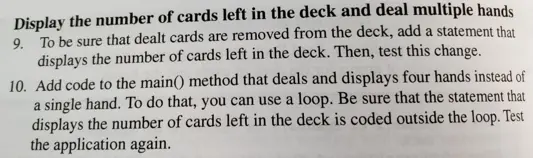
Source Code
import java.util.ArrayList;
public class CardDeckApp {
public static void main(String[] args) {
System.out.println("DECK");
//String[] deck = getDeck();
ArrayList deck = getDeck();
displayCards(deck);
System.out.println("SHUFFLED DECK");
shuffleDeck(deck);
displayCards(deck);
int count = 2;
System.out.println("Dealing 4 hands of " + count + " card each...");
for(int i = 0; i < 4; i++)
{
System.out.println("Hand No. " + (i+1) + ": HAND OF " + count + " CARDS");
//String[] hand = dealCards(deck, count);
ArrayList hand = dealCards(deck, count);
displayCards(hand);
System.out.println("");
}
}
private static ArrayList getDeck() {
String[] suits = {"Spades", "Hearts", "Diamonds", "Clubs"};
String[] ranks = {"Ace", "2", "3", "4", "5", "6", "7",
"8", "9", "10", "Jack", "Queen", "King"};
// Create ArrayList of String
ArrayList deck = new ArrayList (52);
//String[] deck = new String[52];
for (String suit : suits) {
for (String rank : ranks) {
deck.add(rank + " of " + suit);
//deck[i] = rank + " of " + suit;
}
}
return deck;
}
private static void displayCards(ArrayList cards) {
System.out.print("|");
for (String card : cards) {
System.out.print(card + "|");
}
System.out.println();
}
private static void shuffleDeck(ArrayList deck) {
for (int i = 0; i < deck.size(); i++) {
String savedCard = deck.get(i);
int randomIndex = (int) (Math.random() * deck.size()-1);
deck.set(i, deck.get(randomIndex));
//deck[i] = deck[randomIndex];
//deck[randomIndex] = savedCard;
deck.set(randomIndex, savedCard);
}
}
private static ArrayList dealCards(ArrayList deck, int count) {
//String[] hand = Arrays.copyOfRange(deck, 0, count);
ArrayList hand = new ArrayList (count); // create empty
// Now, add the first 'count' elements to hand and remove them from deck
int i = 0;
while(i < count) {
String card = deck.remove(0);
hand.add(card);
i++;
}
// Display number of cards left in deck
System.out.println(deck.size() + " cards left in deck after dealing hand of " + count + " cards.");
return hand;
}
}
Similar Samples
Explore our diverse range of programming samples at ProgrammingHomeworkHelp.com. Our carefully curated examples cover various languages and topics, showcasing our proficiency in solving complex programming challenges. These samples serve as educational resources and demonstrate our commitment to delivering high-quality solutions tailored to your academic or professional needs.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java