Instructions
Objective
Write a java homework, to create student management system.
Requirements and Specifications
.webp)
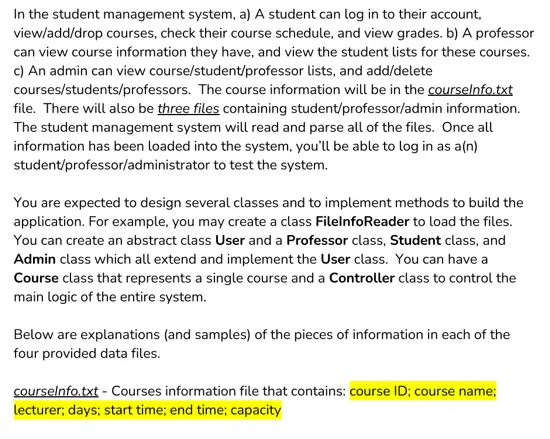
Source Code
ADMIN
public class Admin extends User {
private String AID;
public Admin(String AID, String name, String username, String password) {
this.AID = AID;
this.name = name;
this.username = username;
this.password = password;
}
public boolean checkCredentials(String username, String password) {
// CHeck that the given username and password are equal to this user's username and password
return this.username.compareTo(username) == 0 && this.password.compareTo(password) == 0;
}
public String getID() {return AID;}
@Override
String getUsername() {
// TODO Auto-generated method stub
return username;
}
@Override
String getPassword() {
// TODO Auto-generated method stub
return password;
}
@Override
String getName() {
// TODO Auto-generated method stub
return name;
}
}
APPROVED COURSE
public class ApprovedCourse {
private String CID;
private String grade;
public ApprovedCourse(String CID, String grade) {
this.CID = CID;
this.grade = grade;
}
public String getCID() {
return this.CID;
}
public void setCID(String CID) {
this.CID = CID;
}
public String getGrade() {
return this.grade;
}
public void setGrade(String grade) {
this.grade = grade;
}
}
CONTROLLER
import java.util.List;
import java.util.Scanner;
public class Controller {
public static void main(String[] args) throws Exception {
// Create Scanner object to get user input
Scanner sc = new Scanner(System.in);
// Reader
FileInfoReader reader = new FileInfoReader();
// Read professors Data
List professors = reader.readProfessorData("profInfo.txt");
// Read Admins Data
List admins = reader.readAdminData("adminInfo.txt");
// Read courses
List courses = reader.readCoursesData("courseInfo.txt", professors);
// Read Students Data
List students = reader.readStudentsData("studentInfo.txt", courses);
System.out.println("A total of " + students.size() + " students read.");
System.out.println("A total of " + professors.size() + " professors read.");
System.out.println("A total of " + admins.size() + " admins read.");
System.out.println("A total of " + courses.size() + " courses read.");
// Now, create a User variable that will store the current logged user
User current_user = null;
// Helper variables
String username, password;
String course_id, course_name, course_days, course_startTime, course_endTime, course_capacity_str, course_lecturer_id;
int course_capacity;
String lecturer_id, lecturer_name, lecturer_username, lecturer_password;
String student_id, student_name, student_username, student_password;
lecturer_id = "";
lecturer_name = "";
lecturer_username = "";
lecturer_password = "";
student_id = "";
student_name = "";
student_username = "";
student_password = "";
course_id = "";
course_name = "";
course_days = "";
course_startTime = "";
course_endTime = "";
course_capacity_str = "";
course_lecturer_id = "";
// Start program
boolean running = true;
int option;
while(running) {
// Display menu and get option
option = menu(sc, current_user);
// Process options if the user is not logged in
if(current_user == null)
{
if(option == 1) // login as student
{
username = input(sc, "Please enter your username, or type 'q' to quit: ");
if(username.compareTo("q") != 0) {
password = input(sc, "Please enter your password, or type 'q' to quit: ");
if(password.compareTo("q") != 0) {
// Check if there is an user with these credentials
for(Student student: students) {
if(student.checkCredentials(username, password)) {
current_user = student;
break;
}
}
if(current_user == null) // if current user is still null, this means there is no student with that usernamer and passwprd
{
System.out.println("Username or password incorrect.");
}
}
}
}
else if(option == 2) // login as professor
{
username = input(sc, "Please enter your username, or type 'q' to quit: ");
if(username.compareTo("q") != 0) {
password = input(sc, "Please enter your password, or type 'q' to quit: ");
if(password.compareTo("q") != 0) {
// Check if there is an user with these credentials
for(Professor professor: professors) {
if(professor.checkCredentials(username, password)) {
current_user = professor;
break;
}
}
if(current_user == null) // if current user is still null, this means there is no student with that usernamer and passwprd
{
System.out.println("Username or password incorrect.");
}
}
}
}
else if(option == 3) // login as admin
{
username = input(sc, "Please enter your username, or type 'q' to quit: ");
if(username.compareTo("q") != 0) {
password = input(sc, "Please enter your password, or type 'q' to quit: ");
if(password.compareTo("q") != 0) {
// Check if there is an user with these credentials
for(Admin admin: admins) {
if(admin.checkCredentials(username, password)) {
current_user = admin;
break;
}
}
if(current_user == null) // if current user is still null, this means there is no admin with that usernamer and passwprd
{
System.out.println("Username or password incorrect.");
}
}
}
}
else if(option == 4) // quit
{
running = false;
}
}
else if(current_user instanceof Student) // user is student
{
if(option == 1) // view all courses
{
for(Course course: courses) {
System.out.println(course.toString());
}
}
else if(option == 2) // add courses to your list
{
course_id = input(sc, "Please select the course ID you want to add to your list, eg. 'CIT590'.\nOr enter 'q' to return to the previous menu: ");
if(course_id.toLowerCase().compareTo("q") != 0)
{
// Check if the course exists
Course course = null;
for(Course c: courses) {
if(c.getID().compareTo(course_id) == 0) {
course = c;
break;
}
}
if(course != null) // course exists
{
// check if it is already added into student's courses
if(!((Student)current_user).isEnrolledIn(course_id)) {
// Check if the times overlaps
if(!((Student)current_user).checkCourseOverlaps(course.getStartTime(), course.getEndTime(), course.getDays())){
// Enroll student in course
// Add student to course
course.addStudent((Student)current_user);
((Student)current_user).enrollInCourse(course);
}
else {
System.out.println("Sorry, but the time of this course overlaps with the time of other of your courses.");
}
}
else {
System.out.println("You are already enrolled in this course.");
}
}
else {
System.out.println("There is no course with this ID.");
}
}
}
else if(option == 3) // display enrolled course
{
((Student)current_user).printEnrolledCourses();
}
else if(option == 4) { // drop course
// First, display courses
System.out.println("The courses in your lit:");
for(Course course: ((Student)current_user).getEnrolledCourses()) {
System.out.println(course.toString());
}
course_id = input(sc, "Please enter the ID of the course which you want to drop, eg. 'CIT591'.\nOr enter 'q' to return to the previous menu\n");
if(((Student)current_user).dropCourse(course_id)) {
System.out.println("Course dropped successfully");
}
else {
System.out.println("You are not enrolled in that course.");
}
}
else if(option == 5) // view grades
{
((Student)current_user).printApprovedCourses();
}
else if(option == 6) // return to loigin menu
{
current_user = null;
}
}
else if(current_user instanceof Professor) // user is a professor
{
if(option == 1) { // View given courses
// Loop through courses and print courses where the lecturer is current user
System.out.println("----------The course list----------");
for(Course course: courses) {
if(course.getLecturer().getID() == ((Professor)current_user).getID()){
System.out.println(course.toString());
}
}
}
else if(option == 2) // View student list of the given course
{
for(Course course: courses) {
if(course.getLecturer().getID() == ((Professor)current_user).getID()){
System.out.println(String.format("Students in your course %s %s:", course.getID(), course.getName()));
for(Student student: course.getStudents())
{
System.out.println(student.toString());
}
System.out.println("");
}
}
}
else if(option == 3) // return to login menu
{
current_user = null;
}
}
else if(current_user instanceof Admin) // user is an admin
{
if(option == 1) // view all courses
{
System.out.println("----------The Course List----------");
for(Course course: courses) {
System.out.println(course.toString());
}
}
else if(option == 2) { // add a new course
int talk_state = 1;
String input_str;
while(talk_state != 8) {
if(talk_state == 1) {
course_id = input(sc, "Please enter the course ID, or type 'q' to end.\n");
input_str = course_id;
if(input_str.compareTo("q") == 0) {
break;
}
else{
talk_state++;
}
}
if(talk_state == 2) {
course_name = input(sc, "Please enter the course name, or type 'q' to end.\n");
input_str = course_name;
if(input_str.compareTo("q") == 0) {
break;
}
else{
talk_state++;
}
}
if(talk_state == 3) {
course_startTime = input(sc, "Please enter the course start time, or type 'q' to end. eg. '19:00'\n");
input_str = course_startTime;
if(input_str.compareTo("q") == 0) {
break;
}
else{
talk_state++;
}
}
if(talk_state == 4) {
course_endTime = input(sc, "Please enter the course end time, or type 'q' to end. eg. '20:00'\n");
input_str = course_endTime;
if(input_str.compareTo("q") == 0) {
break;
}
else{
talk_state++;
}
}
if(talk_state == 5) {
course_days = input(sc, "Please enter the course date, or type 'q' to end. eg. 'MW'\n");
input_str = course_days;
if(input_str.compareTo("q") == 0) {
break;
}
else{
talk_state++;
}
}
if(talk_state == 6) {
course_capacity_str = input(sc, "Please enter the course capacity, or type 'q' to end. eg. '72'\n");
input_str = course_capacity_str;
if(input_str.compareTo("q") == 0) {
break;
}
else{
talk_state++;
}
}
if(talk_state == 7) {
course_lecturer_id = input(sc, "Please enter the course lecturer's id, or type 'q' to end. eg. '001'\n");
input_str = course_lecturer_id;
if(input_str.compareTo("q") == 0) {
break;
}
else{
talk_state++;
}
}
}
if(talk_state == 8)
{
// check if course exists
Course course = null;
for(Course c: courses) {
if(c.getID().compareTo(course_id) == 0) {
course = c;
break;
}
}
if(course == null) // course not found, so we proceed
{
// check that lecturer exists
Professor lecturer = null;
for(Professor prof: professors) {
if(prof.getID().compareTo(course_lecturer_id) == 0) {
lecturer = prof;
break;
}
}
if(lecturer != null) // lecturer found, so we proceed
{
// Check taht the times of this new course does not overlaps with the time from other courses
boolean overlaps = false;
for(Course c: courses) {
if(c.getLecturer().getID() == lecturer.getID() && c.overlaps(course_startTime, course_endTime, course_days))
{
overlaps = true;
break;
}
}
if(!overlaps)
{
// Create course object
Course newCourse = new Course(course_id, course_name, lecturer, course_days, course_startTime, course_endTime, Integer.valueOf(course_capacity_str));
courses.add(newCourse);
System.out.println("Successfully added the course: " + newCourse.toString());
}
else{
System.out.println("Sorry, the times for this course are in conflict with the time for another course of this lecturer.");
}
}
else{
System.out.println("Sorry, a lecturer with that id does not exists in the database.");
}
}
else {
System.out.println("Sorry, a Course with that ID already exists.");
}
}
}
else if(option == 3) {// delete a course
course_id = input(sc, "Enter course id, or 'q' to end.\n");
// Check if course exists
Course course = null;
int idx = 0;
for(Course c: courses) {
if(c.getID().compareTo(course_id) == 0) {
course = c;
break;
}
idx++;
}
if(course != null) // course exist
{
// Delete
courses.remove(idx);
System.out.println("Successfully deleted the course: " + course.toString());
}
}
else if(option == 4) { // add new professor
// get id
lecturer_id = input(sc, "Please enter lecturer ID, or type 'q' to quit.\n");
if(lecturer_id.compareTo("q") != 0) {
// ask for name
lecturer_name = input(sc, "Please enter lecturer name, or type 'q' to quit.\n");
if(lecturer_name.compareTo("q") != 0) {
// ask for username
lecturer_username = input(sc, "Please enter lecturer username, or 'q' to quit.\n");
if(lecturer_username.compareTo("q") != 0) {
// password
lecturer_password = input(sc, "Please enter lecturer password, or 'q' to quit.\n");
if(lecturer_password.compareTo("q") != 0) {
// check if the lecturer id exists
boolean ok_id = true;
boolean ok_user = true;
for(Professor prf: professors) {
if(prf.getID().compareTo(lecturer_id) == 0) {
ok_id = false;
}
if(prf.getUsername().compareTo(lecturer_username) == 0) {
ok_user = false;
}
}
if(!ok_id) {
System.out.println("Sorry, a Professor with this ID already exists.");
}
else if(!ok_user) {
System.out.println("Sorry, this username is already registered.");
}
else { // everything is ok
// Create Professor object
Professor professor = new Professor(lecturer_id, lecturer_name, lecturer_username, lecturer_password);
professors.add(professor);
System.out.println("The following Professor has been registered successfully: " + professor.toString());
}
}
}
}
}
}
else if(option == 5) { // Delete professor
lecturer_id = input(sc, "Enter Professor ID\n");
int i = 0;
Professor prof = null;
for(Professor prf: professors) {
if(prf.getID().compareTo(lecturer_id) == 0) {
prof = prf;
break;
}
i++;
}
if(prof != null) {
professors.remove(i);
System.out.println("The following Professor has been removed successfully: " + prof.toString());
}
else {
System.out.println("Sorry, there is no Professor with that ID.");
}
}
else if(option == 6) { // add student
// get id
student_id = input(sc, "Please enter Student ID, or type 'q' to quit.\n");
if(student_id.compareTo("q") != 0) {
// ask for name
student_name = input(sc, "Please enter student name, or type 'q' to quit.\n");
if(student_name.compareTo("q") != 0) {
// ask for username
student_username = input(sc, "Please enter student username, or 'q' to quit.\n");
if(student_username.compareTo("q") != 0) {
// password
student_password = input(sc, "Please enter student password, or 'q' to quit.\n");
if(student_password.compareTo("q") != 0) {
// check if the student id exists
boolean ok_id = true;
boolean ok_user = true;
for(Student st: students) {
if(st.getID().compareTo(student_id) == 0) {
ok_id = false;
}
if(st.getUsername().compareTo(student_username) == 0) {
ok_user = false;
}
}
if(!ok_id) {
System.out.println("Sorry, a Student with this ID already exists.");
}
else if(!ok_user) {
System.out.println("Sorry, this username is already registered.");
}
else { // everything is ok
// Create Professor object
Student student = new Student(student_id, student_name, student_username, student_password);
students.add(student);
System.out.println("The following Student has been registered successfully: " + student.toString());
}
}
}
}
}
}
else if(option == 7) { // remove student
student_id = input(sc, "Enter Student ID\n");
int i = 0;
Student student = null;
for(Student st: students) {
if(st.getID().compareTo(student_id) == 0) {
student = st;
break;
}
i++;
}
if(student != null) {
students.remove(i);
System.out.println("The following Student has been removed successfully: " + student.toString());
}
else {
System.out.println("Sorry, there is no Student with that ID.");
}
}
else if(option == 8) { // return to login menu
current_user = null;
}
}
}
System.out.println("");
}
public static int getOption(Scanner sc, String message, int lb, int ub) {
/*
This function displays 'message' and requests an integer from user
such that lb <= option <= ub
If the user enters an invalid option, this function displays the error message and reprompts
the user
*/
int option;
while(true)
{
try {
System.out.print(message);
option = Integer.valueOf(sc.nextLine());
if(option >= lb && option <= ub) {
return option;
}
else {
System.out.println("Please enter a value between " + lb + " and " + ub + ".");
}
}
catch(Exception ex ){
// If we get an exception, it is because user entered an non numeric value
System.out.println("Please enter a valid numeric option.");
}
}
}
public static int menu(Scanner sc, User current_user) {
/*
Display a menu depending of the type of user and if the user is logged in (not null)
Then, request for a menu option and return it
*/
if(current_user == null) // not logged int
{
System.out.println("--------------------------");
System.out.println("Students management System");
System.out.println("--------------------------");
System.out.println(" 1 -- Login as a student");
System.out.println(" 2-- Login as a professor");
System.out.println(" 3 -- Login as a admin");
System.out.println(" 4 -- Quit the system");
return getOption(sc, "Please enter your option, eg. '1': ", 1, 4);
}
else { // user is logged int. Depending of the type of user, display a different menu
if(current_user instanceof Student) // user is a student
{
// Display student menu
System.out.println("--------------------------");
System.out.println("Welcome, " + current_user.getName());
System.out.println("--------------------------");
System.out.println(" 1 -- View all courses");
System.out.println(" 2 -- Add courses to your list");
System.out.println(" 3 -- View enrolled courses");
System.out.println(" 4 -- Drop courses in your list");
System.out.println(" 5 -- View graded");
System.out.println(" 6 -- Return to previous menu");
return getOption(sc, "Please enter your option, eg. '1': ", 1, 6);
}
else if(current_user instanceof Professor) // current user is a professor
{
// Display professor menu
System.out.println("--------------------------");
System.out.println("Welcome, " + current_user.getName());
System.out.println("--------------------------");
System.out.println(" 1 -- View given courses");
System.out.println(" 2 -- View student list of the given course");
System.out.println(" 3 -- Return to the previous menu");
return getOption(sc, "Please enter your option, eg. '1': ", 1, 6);
}
else /// user is admin
{
// display admin menu
// Display professor menu
System.out.println("--------------------------");
System.out.println("Welcome, " + current_user.getName());
System.out.println("--------------------------");
System.out.println(" 1 -- View all courses");
System.out.println(" 2 -- Add new courses");
System.out.println(" 3 -- Delete courses");
System.out.println(" 4 -- Add new professor");
System.out.println(" 5 -- Delete professor");
System.out.println(" 6 -- Add new student");
System.out.println(" 7 -- Delete student");
System.out.println(" 8 -- Return to previous menu");
return getOption(sc, "Please enter your option, eg. '1': ", 1, 8);
}
}
}
public static String input(Scanner sc, String message)
{
// Function to display message and get user input
System.out.print(message);
return sc.next();
}
}
COURSE
import java.util.ArrayList;
import java.util.List;
public class Course {
private String ID;
private String name;
private Professor lecturer;
private String days;
private String startTime;
private String endTime;
private int capacity;
private List students;
private String grade;
public Course(String course_id, String name, String grade) {
this.ID = course_id;
this.name = name;
this.grade =grade;
}
// Constructor
public Course(String ID, String name, Professor lecturer, String days, String startTime, String endTime, int capacity){
this.ID = ID;
this.name = name;
this.lecturer = lecturer;
this.days = days;
this.startTime = startTime;
this.endTime = endTime;
this.capacity = capacity;
this.students = new ArrayList();
this.grade = "";
}
public void addStudent(Student student) {
this.students.add(student);
}
public List getStudents() {return students;}
public int getStartHour() {
// return the start hour of the course
return Integer.valueOf(getStartTime().split(":")[0]);
}
public int getStartMin() {
// return the start minutes of the course
return Integer.valueOf(getStartTime().split(":")[1]);
}
public int getEndHour() {
// return the end hour of the course
return Integer.valueOf(getEndTime().split(":")[0]);
}
public int getEndMin() {
// return the end minutes of the course
return Integer.valueOf(getEndTime().split(":")[1]);
}
public int getStartTime24() {
// Returns the start time in 24hrs format
return getStartHour()*100 + getStartMin();
}
public int getEndTime24() {
// Returns the end time in 24hrs format
return getEndHour()*100 + getEndMin();
}
public boolean overlaps(String startTime, String endTime, String days) {
int startHour = Integer.valueOf(startTime.split(":")[0]);
int startMin = Integer.valueOf(startTime.split(":")[1]);
int endHour = Integer.valueOf(endTime.split(":")[0]);
int endMin = Integer.valueOf(endTime.split(":")[1]);
int startTime24 = startHour*100 + startMin;
int endTime24 = endHour*100 + endMin;
if(startTime24 >= getStartTime24() && startTime24 <= getEndTime24() && (getDays().indexOf(days) != -1 || days.indexOf(getDays()) != -1)){
return true;
}
else if(endTime24 >= getStartTime24() && endTime24 <= getEndTime24() && (getDays().indexOf(days) != -1 || days.indexOf(getDays()) != -1)) {
return true;
}
return false;
}
// Getters and setters
public String getID() {
return this.ID;
}
public String getName() {
return this.name;
}
public void setName(String name) {
this.name = name;
}
public Professor getLecturer() {
return this.lecturer;
}
public void setLecturer(Professor lecturer) {
this.lecturer = lecturer;
}
public String getDays() {
return this.days;
}
public void setDays(String days) {
this.days = days;
}
public String getStartTime() {
return this.startTime;
}
public void setStartTime(String startTime) {
this.startTime = startTime;
}
public String getEndTime() {
return this.endTime;
}
public void setEndTime(String endTime) {
this.endTime = endTime;
}
public int getCapacity() {
return this.capacity;
}
public void setCapacity(int capacity) {
this.capacity = capacity;
}
public boolean isFinished() {
return grade.length() > 0;
}
public void setGrade(String g) {grade = g;}
public String getGrade() {return grade;}
@Override
public String toString() {
if(!isFinished()) {
return String.format("%s|%s, %s-%s on %s, with course capacity: %d, students: %s, lecturer: %s", getID(), getName(), getStartTime(), getEndTime(), getDays(), getCapacity(), getStudents().size(), lecturer.toString());
}
else {
return String.format("Grade of %s %s: %s", getID(), getName(), getGrade());
}
}
}
FILE INFO READER
import java.io.BufferedReader;
import java.io.FileReader;
import java.util.ArrayList;
import java.util.List;
public class FileInfoReader
{
// Method to read Students
public List readStudentsData(String file_name, List courses)
{
List students = new ArrayList();
String username, password, name, SID;
String course_id, grade;
try{
// Read file
BufferedReader reader = new BufferedReader(new FileReader(file_name));
String line;
while((line = reader.readLine()) != null){
// First, split the line by ;
String[] row = line.split(";");
// The first element is the SID
SID = row[0].strip();
// Second element is name
name = row[1].strip();
// Third element is usernae
username = row[2].strip();
// Fourth element is the password
password = row[3].strip();
// Create Student object
Student student = new Student(SID, name, username, password);
// The last element is a list of courses that we need to split by comma
String approved_courses[] = row[4].split(",");
// Now, each element in courses must be split by :
for(int i = 0; i < approved_courses.length; i++)
{
String course_info[] = approved_courses[i].split(":");
course_id = course_info[0].strip();
grade = course_info[1].strip();
// Get course object
Course course = null;
for(Course c: courses) {
if(c.getID().compareTo(course_id) == 0) {
course = c;
break;
}
}
// add course to user
student.enrollInCourse(new Course(course_id, course.getName(), grade));
}
// Now, add the student to the list of students
students.add(student);
}
// close reader
reader.close();
}
catch(Exception ex){
// If we get an exception it is probably because we could not open the file
System.out.println("File " + file_name + " could not be opened.");
System.out.println(ex.getMessage());
}
return students;
}
// Method to read Professors
public List readProfessorData(String file_name)
{
List professors = new ArrayList();
String username, password, name, PID;
try{
// Read file
BufferedReader reader = new BufferedReader(new FileReader(file_name));
String line;
while((line = reader.readLine()) != null){
// First, split the line by ;
String[] row = line.split(";");
// The first element is the name
name = row[0].strip();
// The second element is the PID
PID = row[1].strip();
// Third element is usernae
username = row[2].strip();
// Fourth element is the password
password = row[3].strip();
// Create Professor object
Professor professor = new Professor(PID, name, username, password);
// Now, add the list
professors.add(professor);
}
// close reader
reader.close();
}
catch(Exception ex){
// If we get an exception it is probably because we could not open the file
System.out.println("File " + file_name + " could not be opened.");
}
return professors;
}
// Method to read Admins
public List readAdminData(String file_name)
{
List admins = new ArrayList();
String username, password, name, AID;
try{
// Read file
BufferedReader reader = new BufferedReader(new FileReader(file_name));
String line;
while((line = reader.readLine()) != null){
// First, split the line by ;
String[] row = line.split(";");
// The first element is the AID
AID = row[0].strip();
// The second element is the name
name = row[1].strip();
// Third element is usernae
username = row[2].strip();
// Fourth element is the password
password = row[3].strip();
// Create Admin
Admin admin = new Admin(AID, name, username, password);
// Now, add the list
admins.add(admin);
}
// close reader
reader.close();
}
catch(Exception ex){
// If we get an exception it is probably because we could not open the file
System.out.println("File " + file_name + " could not be opened.");
}
return admins;
}
// Method to read Courses
public List readCoursesData(String file_name, List professors)
{
List courses = new ArrayList();
String ID, name, days, startTime, endTime;
int capacity;
Professor lecturer = null;
String lecturer_name;
try{
// Read file
BufferedReader reader = new BufferedReader(new FileReader(file_name));
String line;
while((line = reader.readLine()) != null){
// First, split the line by ;
String[] row = line.split(";");
// The first element is the ID
ID = row[0].strip();
// The second element is the name
name = row[1].strip();
// Third element is the lecturer name
lecturer_name = row[2].strip();
// Find lecturer with this name
for(Professor p: professors) {
if(p.getName().compareTo(lecturer_name) == 0) {
lecturer = p;
break;
}
}
// Fourth element is days
days = row[3].strip();
// Fifth is startTIme
startTime = row[4].strip();
//Sisxth is endTime
endTime = row[5].strip();
// Last is capacity
capacity = Integer.valueOf(row[6].strip());
// Create Course
Course course = new Course(ID, name, lecturer, days, startTime, endTime, capacity);
// Now, add the list
courses.add(course);
}
// close reader
reader.close();
}
catch(Exception ex){
// If we get an exception it is probably because we could not open the file
System.out.println("File " + file_name + " could not be opened.");
}
return courses;
}
}
PROFESSOR
public class Professor extends User {
private String PID;
public Professor(String PID, String name, String username, String password) {
this.PID = PID;
this.name = name;
this.username = username;
this.password = password;
}
public boolean checkCredentials(String username, String password) {
// CHeck that the given username and password are equal to this user's username and password
return this.username.compareTo(username) == 0 && this.password.compareTo(password) == 0;
}
public String getID() {return PID;}
@Override
public String toString() {return "Professor " + getName();}
@Override
String getUsername() {
// TODO Auto-generated method stub
return username;
}
@Override
String getPassword() {
// TODO Auto-generated method stub
return password;
}
@Override
String getName() {
// TODO Auto-generated method stub
return name;
}
}
STUDENT
import java.util.ArrayList;
import java.util.List;
public class Student extends User {
private String SID;
private List enrolled_courses;
public Student(String SID, String name, String username, String password) {
this.username = username;
this.password = password;
this.SID = SID;
this.name = name;
enrolled_courses = new ArrayList();
}
public void enrollInCourse(Course course) {
this.enrolled_courses.add(course);
}
public boolean isEnrolledIn(String course_id){
/*
Returns true is the student is enrolled in the given course
*/
for(Course c: enrolled_courses){
if(c.getID().compareTo(course_id) == 0) {
return true;
}
}
return false;
}
public boolean checkCourseOverlaps(String startTime, String endTime, String days)
{
if(enrolled_courses.size() == 0) {
return false;
}
int startHour = Integer.valueOf(startTime.split(":")[0]);
int startMin = Integer.valueOf(startTime.split(":")[1]);
int endHour = Integer.valueOf(endTime.split(":")[0]);
int endMin = Integer.valueOf(endTime.split(":")[1]);
int startTime24 = startHour*100 + startMin;
int endTime24 = endHour*100 + endMin;
for(Course c: enrolled_courses) {
if(startTime24 >= c.getStartTime24() && startTime24 <= c.getEndTime24() && (c.getDays().indexOf(days) != -1 || days.indexOf(c.getDays()) != -1)){
return true;
}
else if(endTime24 >= c.getStartTime24() && endTime24 <= c.getEndTime24() && (c.getDays().indexOf(days) != -1 || days.indexOf(c.getDays()) != -1)) {
return true;
}
}
return false;
}
public boolean checkCredentials(String username, String password) {
// CHeck that the given username and password are equal to this user's username and password
return this.username.compareTo(username) == 0 && this.password.compareTo(password) == 0;
}
public String getID() {return SID;}
public List getEnrolledCourses() {return enrolled_courses;}
public boolean dropCourse(String course_id) {
int i = 0;
for(Course c: getEnrolledCourses()) {
if(c.getID().compareTo(course_id) == 0) {
enrolled_courses.remove(i);
return true;
}
i++;
}
return false;
}
public void printEnrolledCourses() {
if(enrolled_courses.size() > 0) {
for(Course course: enrolled_courses) {
if(!course.isFinished()) {
System.out.println(course.toString());
}
}
}
else{
System.out.println("You are not enrolled in any course.");
}
}
public void printApprovedCourses() {
int approved = 0;
for(Course course: enrolled_courses) {
if(course.isFinished()) {
System.out.println(course.toString());
approved++;
}
}
if(approved == 0) {
System.out.println("You have to approved any course yet.");
}
}
@Override
String getUsername() {
// TODO Auto-generated method stub
return username;
}
@Override
String getPassword() {
// TODO Auto-generated method stub
return password;
}
@Override
String getName() {
// TODO Auto-generated method stub
return name;
}
@Override
public String toString() {
return String.format("%s %s", getID(), getName());
}
}
USER
public abstract class User {
String username;
String password;
String name;
abstract String getUsername();
abstract String getPassword();
abstract String getName();
}
Similar Samples
Visit ProgrammingHomeworkHelp.com to explore our comprehensive samples across Java, Python, C++, and more. Our examples highlight our proficiency in solving diverse programming problems with clarity and precision. Each sample serves as a testament to our commitment to delivering top-notch academic assistance in programming assignments.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java