Instructions
Objective
If you're looking to create a rocket computational system using Python, you're about to embark on an exciting coding journey! Python's versatility and user-friendly nature make it the perfect choice for this project. However, we understand that tackling such an assignment might be challenging at times. If you ever find yourself in need of assistance with your Python assignment, don't hesitate to seek help. There are plenty of resources and experts available online who can guide you through the process, providing valuable insights and ensuring your success. So, embrace the challenge, explore the possibilities, and remember, help is just a click away!
Requirements and Specifications
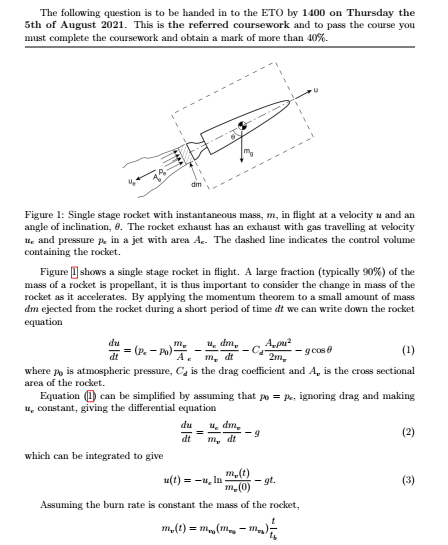
Source Code
QUESTION 1
import numpy as np
from scipy.integrate import solve_ivp
from matplotlib import pyplot as plt
# Constants
Grav = 9.81
RocketMass = 1000 # kg
JetArea = 0.1**2*np.pi
JetVel = 1500.0 #m/s
def rocket(t, state):
h,u,m = state
if m < RocketMass:
DeltaM = 0.0
else:
DeltaM = -JetArea*JetVel
# acceleration
DeltaV = -JetVel/m*DeltaM-Grav
return [u, DeltaV, DeltaM] # dhdt, dudt, dmdt
# event for when rocket crases back to eart
def hit_ground(t,y): return y[0]
hit_ground.terminal = True
hit_ground.direction = -1
# event for burnout
def burnout(t,y): return y[2] - RocketMass
burnout.terminal = False
burnout.direction = -1
# event for apogee
def apogee(t, y): return y[1]
apogee.terminal = False
apogee.direction = -1
# Launch a rocket with 1500kg of fuel and see what happens
sol = solve_ivp(rocket,
[0, 3600], # 1 hour of flight maximum)
[0.0, 0.0, RocketMass + 1500.0], # initial conditions
method = 'LSODA', # stiff ODE solver
dense_output = True,
events = (burnout, apogee, hit_ground))
# Interpret results
print('Burn out at t={:.2f}s, maximum velocity is {:.2f} m/s'.format(sol.t_events[0][0], sol.y_events[0][0][1]))
print('Apogee at t={:.2f}s, maximum altitude is {:.2f} km'.format(sol.t_events[1][0], sol.y_events[1][0][0]/1000))
print('Impact at t={:.2f}s'.format(sol.t_events[2][0]))
# Plot a graph
t = np.linspace(0.0, sol.t_events[2][0], 500)
h = sol.sol(t)
u = h[1]
m = h[2]
plt.plot(t, h[0])
plt.ylabel('Altitude (km)')
plt.xlabel('Time (s)')
plt.axvline(sol.t_events[0][0], color = 'red')
plt.show()
# Now, plot the velocity using the integrated function
mv0 = RocketMass + 1500.0 # mass at ignition
mvb = RocketMass # mass at burnout
tb = sol.t_events[0][0] # time at which all the propellant has been used
U = -JetVel*np.log(m/m[0]) -Grav*t
plt.figure()
plt.plot(t, u, label = 'From Eq. (1)')
plt.plot(t, U, label = 'From Eq. (3)')
plt.legend()
plt.grid(True)
plt.show()
QUESTION 2
import numpy as np
from scipy.integrate import solve_ivp
from matplotlib import pyplot as plt
# Constants
Grav = 9.81
RocketMass = 1000 # kg
JetArea = 0.1**2*np.pi
JetVel = 1500.0 #m/s
Cd = 0.75
p = 1.225 # kg/m^3
d = 31.0/100.0 # Diameter of the cross-sectional area [cm]
A = np.pi*(d/2.0)**2 # cross sectional area
def rocket(t, state):
h,u,m = state
if m < RocketMass:
DeltaM = 0.0
else:
DeltaM = -JetArea*JetVel
# acceleration, here goes the drag force
Fd = (A/2)*Cd*p*u**2
DeltaV = -JetVel/m*DeltaM-Grav - Fd/m
if h < 0.0:
DeltaV = 0.0
return [u, DeltaV, DeltaM] # dhdt, dudt, dmdt
# event for when rocket crases back to eart
def hit_ground(t,y): return y[0]
hit_ground.terminal = True
hit_ground.direction = -1
# event for burnout
def burnout(t,y): return y[2] - RocketMass
burnout.terminal = False
burnout.direction = -1
# event for apogee
def apogee(t, y): return y[1]
apogee.terminal = False
apogee.direction = -1
# Launch a rocket with 1500kg of fuel and see what happens
sol = solve_ivp(rocket,
[0, 3600], # 1 hour of flight maximum)
[0.0, 0.0, RocketMass + 1500.0], # initial conditions
method = 'LSODA', # stiff ODE solver
dense_output = True,
events = (burnout, apogee, hit_ground))
# Interpret results
print('Burn out at t={:.2f}s, maximum velocity is {:.2f} m/s'.format(sol.t_events[0][0], sol.y_events[0][0][1]))
print('Apogee at t={:.2f}s, maximum altitude is {:.2f} km'.format(sol.t_events[1][0], sol.y_events[1][0][0]/1000))
print('Impact at t={:.2f}s'.format(sol.t_events[2][0]))
# Plot a graph
t = np.linspace(0.0, sol.t_events[2][0], 500)
h = sol.sol(t)
plt.plot(t, h[0])
plt.ylabel('Altitude (km)')
plt.xlabel('Time (s)')
plt.axvline(sol.t_events[0][0], color = 'red')
plt.show()
QUESTION 3
import numpy as np
from scipy.integrate import solve_ivp
from matplotlib import pyplot as plt
import time
# Colors for coloured text
TGREEN = '\033[32m' # Green Text
TRED = "\u001b[31m"
TAMBER = "\u001b[33m"
ENDC = '\033[m' # reset to the defaults
# Constants
Grav = 9.81
RocketMass = 1000 # kg
JetArea = 0.1**2*np.pi
JetVel = 1500.0 #m/s
Cd = 0.75
p = 1.225 # kg/m^3
d = 31.0/100.0 # Diameter of the cross-sectional area [cm]
A = np.pi*(d/2.0)**2 # cross sectional area
def rocket(t, state):
h,u,m = state
if m < RocketMass:
DeltaM = 0.0
else:
DeltaM = -JetArea*JetVel
# acceleration, here goes the drag force
Fd = (A/2)*Cd*p*u**2
DeltaV = -JetVel/m*DeltaM-Grav - Fd/m
if h < 0.0:
DeltaV = 0.0
return [u, DeltaV, DeltaM] # dhdt, dudt, dmdt
# event for when rocket crases back to eart
def hit_ground(t,y): return y[0]
hit_ground.terminal = True
hit_ground.direction = -1
# event for burnout
def burnout(t,y): return y[2] - RocketMass
burnout.terminal = False
burnout.direction = -1
# event for apogee
def apogee(t, y): return y[1]
apogee.terminal = False
apogee.direction = -1
# Launch a rocket with 1500kg of fuel and see what happens
sol = solve_ivp(rocket,
[0, 3600], # 1 hour of flight maximum)
[0.0, 0.0, RocketMass + 1500.0], # initial conditions
method = 'LSODA', # stiff ODE solver
dense_output = True,
events = (burnout, apogee, hit_ground))
# Interpret results
#print('Burn out at t={:.2f}s, maximum velocity is {:.2f} m/s'.format(sol.t_events[0][0], sol.y_events[0][0][1]))
#print('Apogee at t={:.2f}s, maximum altitude is {:.2f} km'.format(sol.t_events[1][0], sol.y_events[1][0][0]/1000))
#print('Impact at t={:.2f}s'.format(sol.t_events[2][0]))
# Time vector
t = np.linspace(0.0, sol.t_events[2][0], 500)
# Variables
y = sol.sol(t)
h = y[0]
v = y[1]
m = y[2]
tb = sol.t_events[0][0]
vmax = sol.y_events[0][0][1]
tapg = sol.t_events[1][0]
hmax = sol.y_events[1][0][0]/1000.0
timpact = sol.t_events[2][0]
# Initialize countdown
for i in range(0, 10):
print("T - {} seconds".format(10-i))
time.sleep(1)
print("Lit off!")
time_counter = 5.0
dt = t[1]-t[0]
burnout_printed = False
impact_printed = False
apogee_printed = False
TEXT_COLOR = TGREEN
tsim = 0
for i in range(len(t)):
time_counter += dt
tsim += dt
if tsim >= tb and not burnout_printed:
TEXT_COLOR = TAMBER
print(TEXT_COLOR + "T + {:.0f} seconds, burnout occurred, maximum velocity is {:.2f} m/s".format(tb, vmax), ENDC)
burnout_printed = True
if tsim >= tapg and not apogee_printed:
print(TEXT_COLOR + 'T + {:.0f} seconds, apogee occurred, maximum altitude is {:.2f} km'.format(tapg, hmax), ENDC)
apogee_printed = True
if tsim >= timpact and not impact_printed:
print(TEXT_COLOR + 'T + {:.0f} seconds, impact occured'.format(timpact), ENDC)
impact_printed = True
if v[i] < 0: # rocket is descending
TEXT_COLOR = TRED
if time_counter >= 5.0:
# Print
fuel_remaining = (m[i]-RocketMass)/(RocketMass + 1500.0) * 100.0
if fuel_remaining < 0:
fuel_remaining = 0.0
print(TEXT_COLOR + "T + {:.0f} seconds, velocity is {:.2f} m/s, altitude is {:.2f} km, remaining fuel is {:.2f}%".format(
t[i],
v[i],
h[i]/1000.0,
fuel_remaining
), ENDC)
time_counter = 0
time.sleep(1)
QUESTION 4
import numpy as np
from scipy.integrate import solve_ivp
from matplotlib import pyplot as plt
import time
# Colors for coloured text
TGREEN = '\033[32m' # Green Text
TRED = "\u001b[31m"
TAMBER = "\u001b[33m"
ENDC = '\033[m' # reset to the defaults
# Constants
Grav = 9.81
RocketMass = 1000 # kg
JetArea = 0.1**2*np.pi
JetVel = 1500.0 #m/s
Cd = 0.75
p = 1.225 # kg/m^3
d = 31.0/100.0 # Diameter of the cross-sectional area [cm]
A = np.pi*(d/2.0)**2 # cross sectional area
# Initial conditions of launch
h0 = 83 # in meters. This is the height of the launch tower
fuel_mass = 5000.0 # fuel mass
def rocket(t, state):
h,u,m = state
if m < RocketMass:
DeltaM = 0.0
else:
DeltaM = -JetArea*JetVel
# acceleration, here goes the drag force
Fd = (A/2)*Cd*p*u**2
DeltaV = -JetVel/m*DeltaM-Grav - Fd/m
if h < 0.0:
DeltaV = 0.0
return [u, DeltaV, DeltaM] # dhdt, dudt, dmdt
# event for when rocket crases back to eart
def hit_ground(t,y): return y[0]
hit_ground.terminal = True
hit_ground.direction = -1
# event for burnout
def burnout(t,y): return y[2] - RocketMass
burnout.terminal = False
burnout.direction = -1
# event for apogee
def apogee(t, y): return y[1]
apogee.terminal = False
apogee.direction = -1
# Launch a rocket with 1500kg of fuel and see what happens
sol = solve_ivp(rocket,
[0, 3600], # 1 hour of flight maximum)
[h0, 0.0, RocketMass + fuel_mass], # initial conditions
method = 'LSODA', # stiff ODE solver
dense_output = True,
events = (burnout, apogee, hit_ground))
# Interpret results
#print('Burn out at t={:.2f}s, maximum velocity is {:.2f} m/s'.format(sol.t_events[0][0], sol.y_events[0][0][1]))
#print('Apogee at t={:.2f}s, maximum altitude is {:.2f} km'.format(sol.t_events[1][0], sol.y_events[1][0][0]/1000))
#print('Impact at t={:.2f}s'.format(sol.t_events[2][0]))
# Time vector
t = np.linspace(0.0, sol.t_events[2][0], 500)
# Variables
y = sol.sol(t)
h = y[0]
v = y[1]
m = y[2]
tb = sol.t_events[0][0]
vmax = sol.y_events[0][0][1]
tapg = sol.t_events[1][0]
hmax = sol.y_events[1][0][0]/1000.0
timpact = sol.t_events[2][0]
# Initialize countdown
for i in range(0, 10):
print("T - {} seconds".format(10-i))
time.sleep(1)
print("Lit off!")
time_counter = 5.0
dt = t[1]-t[0]
burnout_printed = False
impact_printed = False
apogee_printed = False
TEXT_COLOR = TGREEN
tsim = 0
for i in range(len(t)):
time_counter += dt
tsim += dt
if tsim >= tb and not burnout_printed:
TEXT_COLOR = TAMBER
print(TEXT_COLOR + "T + {:.0f} seconds, burnout occurred, maximum velocity is {:.2f} m/s".format(tb, vmax), ENDC)
burnout_printed = True
if tsim >= tapg and not apogee_printed:
print(TEXT_COLOR + 'T + {:.0f} seconds, apogee occurred, maximum altitude is {:.2f} km'.format(tapg, hmax), ENDC)
apogee_printed = True
if tsim >= timpact and not impact_printed:
print(TEXT_COLOR + 'T + {:.0f} seconds, impact occured'.format(timpact), ENDC)
impact_printed = True
if v[i] < 0: # rocket is descending
TEXT_COLOR = TRED
if time_counter >= 5.0:
# Print
fuel_remaining = (m[i]-RocketMass)/(RocketMass + fuel_mass) * 100.0
if fuel_remaining < 0:
fuel_remaining = 0.0
print(TEXT_COLOR + "T + {:.0f} seconds, velocity is {:.2f} m/s, altitude is {:.2f} km, remaining fuel is {:.2f}%".format(
t[i],
v[i],
h[i]/1000.0,
fuel_remaining
), ENDC)
time_counter = 0
time.sleep(0.5)
Related Samples
Access our free Python assignment samples here to enhance your coding skills. These samples cover a range of topics and provide practical examples to help you understand key concepts and improve your programming abilities. Perfect for students and beginners looking to learn and practice Python.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python