Instructions
Requirements and Specifications
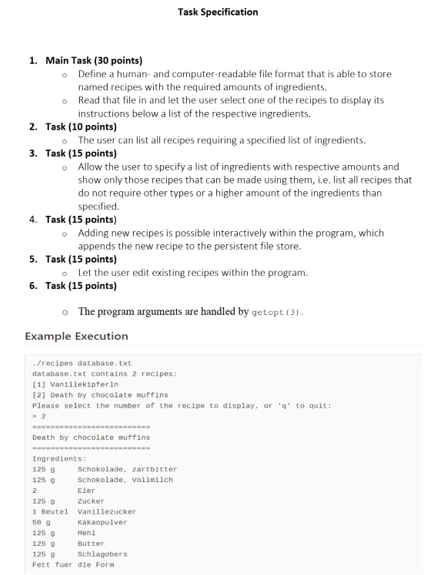
Source Code
/* Ex 3 Queries from a Business Purpose */
/* In Ex 1 you inserted data into the IT Asset Management (ITAM) schema tables
of user jaherna42. In Ex 2 you wrote wrote select command with explicit join
syntax. The tasks for this exercise refer to the ITAM tables of user jaherna42
schema on the class server.
Before a task is presented to you here, an example from the Murach book is
given that is somewhat similar (structurally) to the query you need to write
for the task. The example from Murach refers to the AP tables of user ap or
user jdoe22. The task that follows the example refers to the ITAM business
process and schema.
The deliverable for this exercise is a Word document named
ex3_lastname_firstname_section#.docx. Put your name and the exercise number
(Ex 3) as a heading in a Word document as you begin the exercise.*/
/*Here in the exercise instructions, you are prompted to (1) complete tasks,
(2) take screen shots that document your hands-on work and insert them into
the deliverable document, and/or (3) write something in the deliverable
document. Use a tool to capture just the portion of the screen you need to
document your work.
Whenever you capture work with a screenshot, include your name in the
screenshot. This guideline applies to any screenshots you take to document your
hands-on work throughout the semester. You may not get credit for something if
you do not include your name as requested.*/
/*Connect to the class server using your assigned username.*/
/* For each task, do not get data from the it_asset_inv_summary table
unless the task specifically asks you to do so. */
/*Example 1: Murach Chapter 4 #3 tells you to write a SELECT statement that
returns three columns from two different tables. A business reason that a
manager might state for the query is, "I need a list of vendors and a
description of what type of product they normally sell us. By that I mean, you
know, the description of how the things we usually buy from them are categorized
in our accounting system." */
select v.vendor_name, gla.account_description
from jdoe22.vendors v join jdoe22.general_ledger_accounts gla
on v.default_account_number=gla.account_number;
/* Task 1. Your manager says, "I need a list of all our employees and their cell
mobile phone numbers. Put the list in alphabetical order by their last name
followed by their first name initial. Then put their cell number and, last, put
which department they work in. At the top of each column put Employee Name, Cell
No., and Department."
In addition to your name and your query, your screenshot should include the
All Rows Fetched: n in x.xxx seconds response from Oracle C-language Developer. Make
sure n is the total number of rows fetched by scrolling to the end of the
returned data. Include about 5 records of returned data (does not have to be
exactly 5). */
--Task 1 Employee List
--Type Your Name Here
/* Task 2. Your manager says, "Get me descriptions of different IT assets we have
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
// Create a struct that will help us store recipes
struct Recipe
{
char title[100];
char ingredients[100][100];
char instructions[100][100];
int n_ingredients;
int n_instructions;
};
int main(int argc, char** argv) {
char *filename; // store filename here
char line[200]; // store lines read from file
FILE *fin;
int data;
// Create array to store recipes
struct Recipe recipes[100]; // up to 100 recipes
struct Recipe recipe; // store each recipe read from file
recipe.n_ingredients = 0;
recipe.n_instructions = 0;
int n = 0;
// Get filename
int opt;
while ((opt = getopt(argc, argv, "f:")) != -1)
{
switch (opt)
{
case 'f':
filename = optarg;
break;
}
}
//strncpy(filename, argv[1], strlen(argv[1]));
fin = fopen(filename, "r");
while(fgets(line, 200, fin)) {
//printf("LINE %s\n", line);
//fscanf(fin, "%s", line);
if(line[0] == '+') // it is a title
{
if(strlen(recipe.title) > 0) { // cuyrrent recipe is not null
recipes[n] = recipe;
n++;
recipe.n_ingredients = 0;
recipe.n_instructions = 0;
memset(&recipe.title[0], 0, sizeof(recipe.title));
}
char* title = line+1;
strncpy(recipe.title, title, strlen(title)-1);
}
else if(line[0] == '*') // an ingredient
{
char* ing = line+1;
strncpy(recipe.ingredients[recipe.n_ingredients], ing, strlen(ing)-1);
recipe.n_ingredients++;
}
else if(line[0] == '-')
{
char* inst = line+1;
strncpy(recipe.instructions[recipe.n_instructions], inst, strlen(inst)-1);
recipe.n_instructions++;
}
else if(strcmp(line, "END") == 0) {
recipes[n] = recipe;
n++;
break;
}
line[0] = 0;
}
fclose(fin);
printf("%s contains %d recipes:\n", filename, n);
int nstr;
char user_input;
int option;
while(1) {
// Display recipes
printf("====================\n");
for(int i = 0; i < n; i++) {
printf("[%d] %s\n", i+1, recipes[i].title);
}
printf("====================\n");
printf("Please select the number of the recipe, or 'q' to quit: ");
scanf(" %c", &user_input);
if(user_input == 'q') {
printf("Good Bye!");
break;
} else {
option = user_input - '0';
if(option >= 1 && option <= n) {
recipe = recipes[option-1];
// Display title
nstr = strlen(recipe.title);
for(int j = 0; j < nstr; j++) {
printf("=");
}
printf("\n");
printf("%s\n", recipe.title);
for(int j = 0; j < nstr; j++) {
printf("=");
}
printf("\n");
printf("Ingredients:\n");
for(int i = 0; i < recipe.n_ingredients; i++) {
printf("%s\n", recipe.ingredients[i]);
}
printf("\n\n");
printf("Instructions:\n");
for(int i = 0; i < recipe.n_instructions; i++) {
printf("%s\n", recipe.instructions[i]);
}
}
}
}
return 0;
}
Related Samples
Explore our C Assignments samples featuring essential topics such as arrays, pointers, loops, and functions. Master programming fundamentals with annotated code examples and practical exercises. Enhance your understanding and problem-solving abilities in C programming with curated assignments designed to support your academic and learning goals.
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C