Instructions
Objective
Write a program to create number sequence in java language
Requirements and Specifications
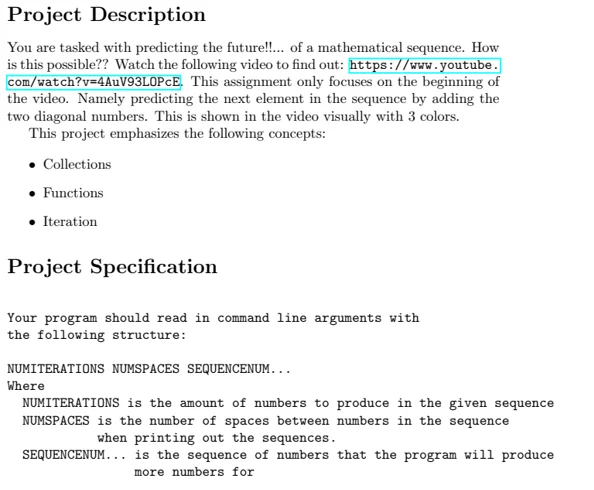
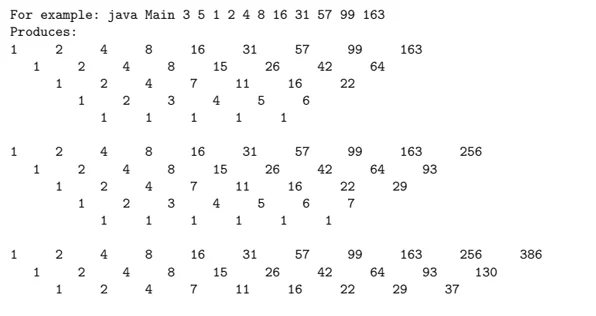
Source Code
PAIR
import java.util.Objects;
/**
* Generic pair that hold two pieces of data
*/
public class Pair {
private final T fst;
private final V snd;
public Pair(T fst, V snd) {
this.fst = fst;
this.snd = snd;
}
public T getFst() {
return fst;
}
public V getSnd() {
return snd;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Pair pair = (Pair) o;
return fst.equals(pair.fst) && snd.equals(pair.snd);
}
@Override
public int hashCode() {
return Objects.hash(fst, snd);
}
}
SEQUENCE
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
* Represents a sequence of Integers.
* Backed by a list of integers.
* Defines two iterables, one that iterates over the
* backing list normally, elementwise.
* The other iterates over the list pairwise.
*/
public class Sequence {
private final List sequence;
public Sequence(List sequence) {
this.sequence = sequence;
}
public void add(Integer i) {
sequence.add(i);
}
public Integer get(int index) {
return sequence.get(index);
}
public int size() {
return sequence.size();
}
public Iterable elementWiseIterator() {
return new Iterable<>() {
@Override
public Iterator iterator() {
return sequence.iterator();
}
};
}
/**
* Defines a pairwise iterator for the sequence.
* Pairwise iteration iterates over the list in pairs.
* For example with the following sequence: [1,2,3]
* Pairwise iteration would first return (1,2) then (2,3)
* then stops.
*/
public Iterable> pairWiseIterator() {
// TODO: Replace return null with your code
// Create list of Pairs
List> pairs = new ArrayList>();
for(int i = 0; i < sequence.size()-1; i++)
{
int x = sequence.get(i);
int y = sequence.get(i+1);
Pair pair = new Pair(x,y);
pairs.add(pair);
}
Iterable> iterable = (Iterable>)pairs;
return iterable;
}
@Override
public String toString() {
return sequence.toString();
}
}
Similar Samples
Explore our sample programming assignments to understand our approach. Each sample showcases our expert solutions, detailed explanations, and commitment to helping you grasp complex concepts with ease. Trust us to elevate your programming skills and ace your assignments.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java