Instructions
Requirements and Specifications
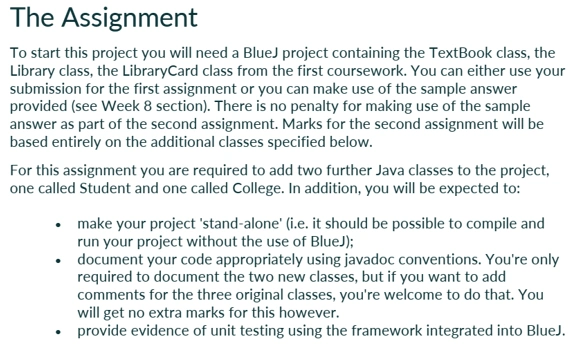
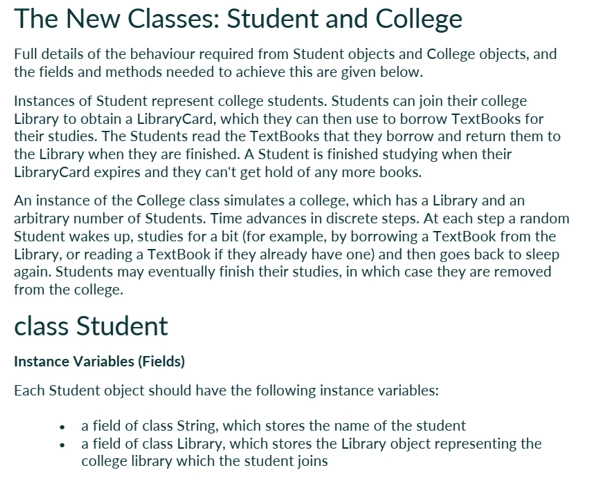
Source Code
COLLEGE
import java.util.ArrayList;
import java.util.Random;
public class College {
private ArrayList students;
private Library library;
private Random random;
public College(int n_students, int n_books) {
library = new Library(n_books);
tudents = new ArrayList ();
// now, add n_dtudents
for(int i = 0; i < n_students; i++) {
students.add(new Student("Student " + (i+1), library));
}
random = new Random();
}
// getters and setters
public ArrayList getStudents() {return students;}
public Library getLibrary() {return library;}
public Random getRandom() {return random;}
// methods
public void describe() {
System.out.println("The college currently has " + getStudents().size() + " hard-working students");
getLibrary().describe();
}
private void nextStep() {
if(getStudents().size() == 0) // no students left
{
System.out.println("Everything has gone quiet.");
}
else
{
// Pick a random student
// Pick index
int index = getRandom().nextInt(getStudents().size());
Student student = getStudents().get(index);
if(student.finishedStudies())
{
// Remove student
students.remove(index);
System.out.println("The student has graduated and left the college.");
}
else{
student.study();
}
}
}
public void runCollege(int nSteps) {
for(int i = 1; i <= nSteps; i++)
{
System.out.println("Step " + i);
describe();
nextStep();
}
}
public static void main(String[] args) {
// First of all, create a College
// Define the number of students and books
int n_students = 10;
int n_books = 15;
College college = new College(n_students, n_books);
college.runCollege(100);
}
}
LIBRARY
public class Library
{
private TextBook[] bookShelf;
private int nextBook;
private int borrowers;
public Library(int numOfBooks)
{
bookShelf = new TextBook[numOfBooks];
for (int i = 0; i < bookShelf.length; i++ ) {
String title = "text_" + i;
bookShelf[i] = new TextBook(title,5);
}
nextBook = 0;
borrowers = 0;
}
public LibraryCard issueCard()
{
LibraryCard aCard = new LibraryCard(borrowers, 5);
borrowers++;
return aCard;
}
public TextBook borrowBook(LibraryCard card)
{
TextBook book = null;
if ( (nextBook < bookShelf.length) && !card.expired() ) {
book = bookShelf[ nextBook ];
bookShelf[ nextBook ] = null;
nextBook++;
card.swipe();
}
return book;
}
public void returnBook(TextBook book)
{
bookShelf[ nextBook-1 ] = book;
nextBook--;
}
public void describe()
{
System.out.print("The library has ");
System.out.print((bookShelf.length-nextBook) + " books on the shelf, and ");
System.out.println("has issued " + borrowers + " cards.");
}
}
STUDENT
public class Student {
private String name;
private Library library;
private LibraryCard libraryCard;
private TextBook textBook;
// Constructor
public Student(String name, Library library) {
this.name = name;
this.library = library;
// Initialize library card
this.libraryCard = this.library.issueCard();
this.textBook = null;
}
// getters
public String getName() {return name;}
public Library getLibrary() {return library;}
public LibraryCard getLibraryCard() {return libraryCard;}
public TextBook getTextBook() {return textBook;}
// setters
public void setName(String newname) {name = newname;}
public void setLibrary(Library newlib) {library = newlib;}
public void setLibraryCard(LibraryCard newcard) {libraryCard = newcard;}
public void setTextBook(TextBook book) {textBook = book;}
// methods
public boolean finishedStudies() {
return getTextBook() == null && getLibraryCard().expired();
}
public void study() {
if(getTextBook() == null) { // student does not have a book
// try to borrow one from libraty
setTextBook(getLibrary().borrowBook(getLibraryCard()));
}
else // student has a book
{
if(getTextBook().isFinished()) // book is not finished
{
getTextBook().readNextChapter();
}
else // book is finished
{
getTextBook().closeBook(); // close it
getLibrary().returnBook(getTextBook()); // return to library
setTextBook(null); // now student does not have a book
}
}
}
public void describe() {
if(getTextBook() == null) {
System.out.print("Student " + getName() + " does not have a book and ");
getLibraryCard().describe();
}
else // has a book
{
System.out.print("Student " + getName() + " has a book ");
getTextBook().describe();
if(!getTextBook().isFinished()) {
System.out.println("The student is reading the book.");
}
else {
System.out.println("The book is finished");
}
}
}
}
Related Samples
Dive into our Database Assignments sample section for hands-on learning. Explore SQL queries, database design, normalization techniques, and more. Each assignment is tailored to sharpen your database management skills and deepen your understanding of relational databases. Master database concepts with our expertly crafted assignments and excel in your studies.
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database
Database