Instructions
Objective
If you need C++ assignment help, don't worry! We can assist you in writing a program to create an integer tracking system using the C++ language. Whether you're a beginner or facing specific challenges with your assignment, our experts are here to guide you through the process. Let's get started with the program implementation!
Requirements and Specifications
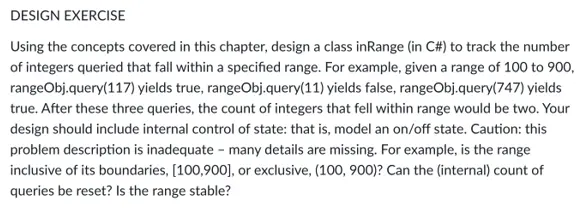
Source Code
IN RANGE
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace YangAssignment
{
class inRange
{
private int counter;
private int lowerBound;
private int upperBound;
private bool active; // control variable. When the control variable is off, the class won't count
public inRange(int lower, int upper)
{
/*
* This constructor creates an inRange object given the lower and upper bounds of the range
* The lower bound is inclusive while the upper bound is exclusive
*
* */
// check that upper is higher than lower
if (upper > lower)
{
// Define the lower and upper bounds of the range
this.lowerBound = lower;
this.upperBound = upper;
}
else
{
Console.WriteLine("Failed to construct object of type inRange -> Upper bound is less or equal than Lower bound.");
throw new ArgumentOutOfRangeException();
}
}
// getters and setters
public int getLowerBound() { return lowerBound; }
public int getUpperBound() { return upperBound; }
public int getCounter() { return counter; }
// Function to test the element
public bool query(int n)
{
if (n >= lowerBound && n < upperBound && active)
{
counter += 1;
return true;
}
else
return false;
}
// function to reset counter
public void reset() { counter = 0; }
// method to enable/disable the control variable
public void switchOn() { active = true; }
public void switchOff() { active = false; }
}
}
PROGRAM
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace YangAssignment
{
class Program
{
static void Main(string[] args)
{
inRange rangeObj = new inRange(100, 900);
Console.WriteLine(rangeObj.query(117));
Console.WriteLine(rangeObj.query(11));
Console.WriteLine(rangeObj.query(747));
Console.WriteLine(rangeObj.getCounter());
Console.Read();
}
}
}
Related Samples
Check out our free C++ assignment samples to enhance your understanding and skills. These examples provide detailed solutions and valuable insights, simplifying complex concepts and boosting your academic performance.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++