Instructions
Objective
Write a java assignment program to create game project in java language.
Requirements and Specifications
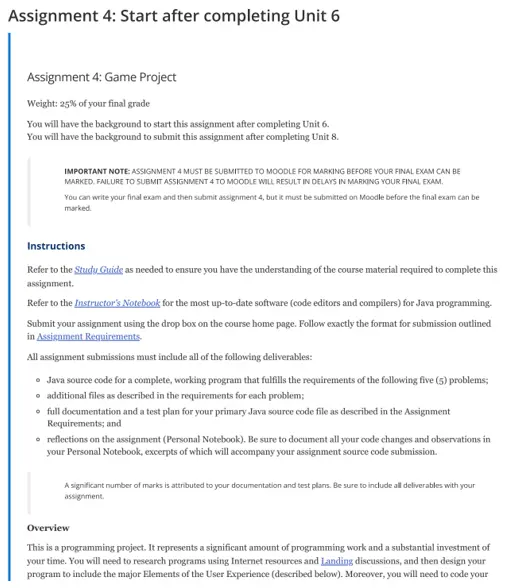
Source Code
APP
public class App {
public static void main(String[] args) throws Exception {
Game game = new Game();
game.loadData("locations.txt", "items.txt", "characters.txt");
game.start();
}
}
CHARACTER
import java.util.List;
public class Character {
private int id;
private String name;
private String description;
private int sex; // 1 for male and 0 for female
private String dialog;
private int requiresAnswer;
private int requiredItemId;
private int rewardItemId;
private boolean rewardDelivered;
// Default constructor
public Character()
{
dialog = "";
name = "";
description = "";
id = -1;
requiredItemId = -1;
rewardItemId = -1;
rewardDelivered = false;
}
public int getId() {
return this.id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return this.name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return this.description;
}
public void setDescription(String description) {
this.description = description;
}
public int getSex() {
return this.sex;
}
public void setSex(int sex) {
this.sex = sex;
}
public String getDialog() {
return this.dialog;
}
public void setDialog(String dialog) {
this.dialog = dialog;
}
public int getRequiredItemId() {
return this.requiredItemId;
}
public void setRequiredItemId(int requiredItemId) {
this.requiredItemId = requiredItemId;
}
public int getRewardItemId() {
return this.rewardItemId;
}
public void setRewardItemId(int rewardItemId) {
this.rewardItemId = rewardItemId;
}
// static method to parse data
public static Character parseData(String data) {
String row[] = data.split(",");
Character character = new Character();
character.setId(Integer.valueOf(row[0]));
character.setName(row[1]);
character.setDescription(row[2]);
character.setSex(Integer.valueOf(row[3]));
character.setDialog(row[4]);
character.requiresAnswer = Integer.valueOf(row[5]);
character.setRequiredItemId(Integer.valueOf(row[6]));
character.setRewardItemId(Integer.valueOf(row[7]));
return character;
}
// method
public boolean startDialog(Control control, Player player, List items)
{
if(requiresAnswer == 1) {
System.out.print(getName() + ": " + getDialog());
int input = control.getYesNo();
if(input == 1) {
// check if player has item
Item item = null;
for(Item it: items) {
if(it.getId() == requiredItemId) {
item = it;
break;
}
}
if(player.hasItem(item)) {
System.out.print(getName() + ": Thank you Alice");
player.removeItem(item);
if(rewardItemId != -1) {
Item reward = null;
for(Item it: items) {
if(it.getId() == rewardItemId) {
reward = it;
break;
}
}
System.out.print("!, please take this reward with you!\n");
System.out.println("You received: " + reward.toString());
player.getInventory().addItem(reward);
requiresAnswer = 0;
}
else {
System.out.print("!\n");
}
return true;
}
else {
System.out.println(getName() + ": Do not lie to me Alice, you do not have it!");
return false;
}
}
else{
System.out.println(getName() + ": Then, go back and find it.");
return false;
}
}
else
{
System.out.print(getName() + ": Hello Alice, good to see you!");
return true;
}
}
}
CONTROL
import java.util.Scanner;
public class Control {
private Scanner sc;
public Control()
{
sc = new Scanner(System.in);
}
public int getMovement(){
String input;
while(true)
{
// ask user for movement (north, south, etc)
input = sc.nextLine().toLowerCase();
if(input.compareTo("north") == 0)
return 0;
else if(input.compareTo("south") == 0)
return 1;
else if(input.compareTo("east") == 0)
return 2;
else if(input.compareTo("west") == 0)
return 3;
else
System.out.println("Please enter a valid movement.");
}
}
public int getTakeOrIgnore()
{
String input;
while(true)
{
// ask user for action (take or ignore)
input = sc.nextLine().toLowerCase();
String[] input_lst = input.split(" ");
if(input_lst[0].compareTo("take") == 0)
return Integer.valueOf(input_lst[1]);
else if(input.compareTo("ignore") == 0)
return 0;
else
System.out.println("Please enter a valid action.");
}
}
public int getYesNo() {
String input;
while(true)
{
// ask user for action (take or ignore)
input = sc.nextLine().toLowerCase();
if(input.compareTo("yes") == 0)
return 1;
else if(input.compareTo("no") == 0)
return 0;
else
return -1;
}
}
}
Similar Samples
Discover exemplary programming homework solutions at ProgrammingHomeworkHelp.com. Our comprehensive samples span various programming languages and topics, offering insights into our proficiency and dedication to academic excellence. Explore how our expertly crafted assignments can aid in enhancing your understanding and skills in programming.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java